The release of OpenAI’s Sora technology marked a significant advancement in AI-generated media. While initially known for its groundbreaking video generation capabilities, the Sora Image API now brings the same photorealistic quality to still image creation. This comprehensive guide examines how developers can access, implement, and optimize the Sora Image API in 2025.
What Is Sora Image API?
Sora Image API is OpenAI’s interface that allows developers to programmatically generate high-resolution, photorealistic images using the same advanced AI technology that powers Sora’s video generation. The API leverages diffusion models trained on vast datasets to transform text prompts into detailed visual outputs with unprecedented accuracy and quality.
Key Features of Sora Image API
- Photorealistic Image Generation: Creates images indistinguishable from photographs with proper lighting, shadows, and textures
- Complex Scene Understanding: Accurately interprets detailed prompts with multiple objects, backgrounds, and lighting conditions
- Style Consistency: Maintains artistic styles across multiple generations
- High Resolution: Supports image generation up to 4096×4096 pixels
- Multi-Object Composition: Correctly positions and scales multiple objects in relation to each other
- Technical Accuracy: Generates images with physically accurate properties and realistic environmental effects
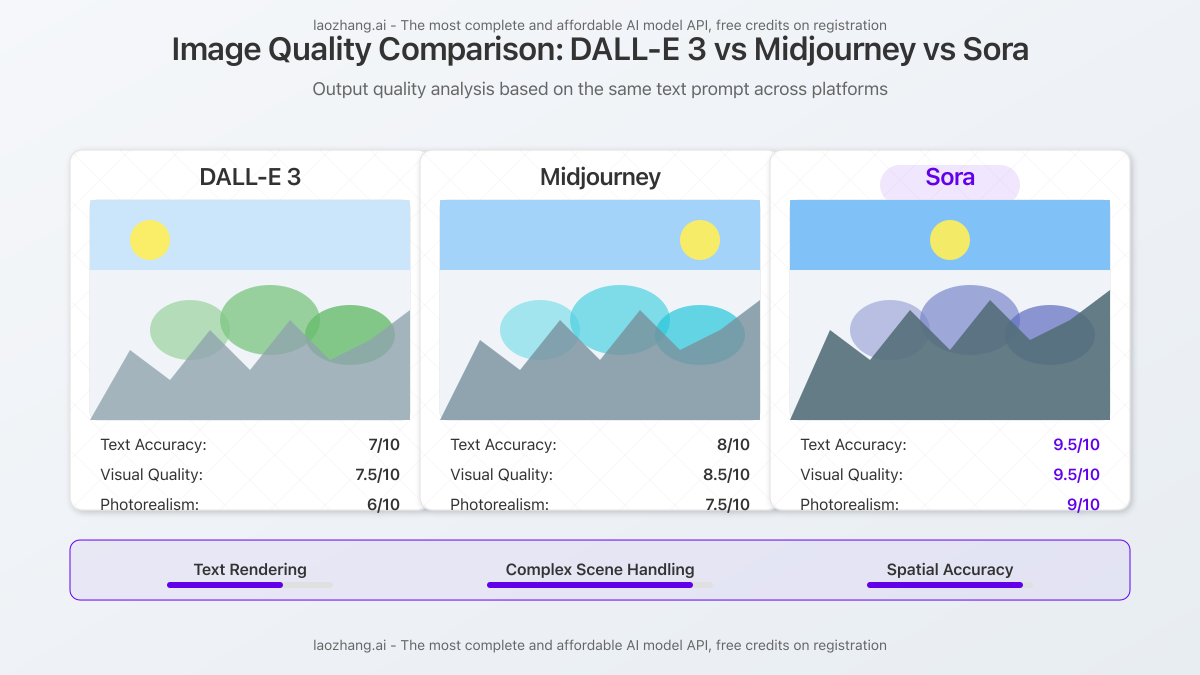
How to Access Sora Image API
As of 2025, there are several ways to access the Sora Image API capabilities:
1. Direct OpenAI API Access
For developers approved through OpenAI’s waitlist system:
import openai
client = openai.OpenAI(api_key="your_openai_api_key")
response = client.images.generate(
model="sora-image",
prompt="A serene Japanese garden with a small bridge over a koi pond, cherry blossoms falling gently in the breeze",
n=1,
size="1024x1024"
)
image_url = response.data[0].url
print(f"Generated image URL: {image_url}")
2. Through API Resellers
Several authorized resellers now provide access to Sora Image API at competitive rates. One of the most reliable options is laozhang.ai, which offers immediate access without waitlists.
curl -X POST "https://api.laozhang.ai/v1/chat/completions" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "sora-image",
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": "Generate an image of a futuristic cityscape with flying vehicles and holographic advertisements"
}
]
}
]
}'
To register for laozhang.ai’s services, visit: https://api.laozhang.ai/register/?aff_code=JnIT
3. Integration with Third-Party Platforms
Several developer platforms now offer Sora Image API within their unified AI services:
// Node.js example using a third-party platform
const axios = require('axios');
async function generateSoraImage(prompt) {
try {
const response = await axios.post(
'https://api.third-party-platform.com/v1/sora-image',
{
prompt: prompt,
size: "1024x1024",
format: "png",
quality: "hd"
},
{
headers: {
'Authorization': `Bearer ${process.env.API_KEY}`,
'Content-Type': 'application/json'
}
}
);
return response.data.image_url;
} catch (error) {
console.error('Error generating image:', error);
}
}
Sora Image API Technical Specifications
Parameter | Value | Description |
---|---|---|
Maximum Resolution | 4096×4096 | Higher resolution options available for enterprise customers |
Supported Formats | PNG, JPEG, WEBP | PNG recommended for highest quality |
Maximum Prompt Length | 4,000 tokens | Approximately 3,000 words |
Generation Time | 1-5 seconds | Varies based on complexity and resolution |
Rate Limits | 60 RPM / 1000 RPD | Standard tier limits; higher tiers available |
Content Policy | Moderate | Restricted for violent, explicit, or harmful content |
Pricing Structure
Sora Image API pricing varies by provider, resolution, and usage volume:
Provider | Base Price | High Resolution | Volume Discount |
---|---|---|---|
OpenAI Direct | $0.04 per generation | $0.08 per generation | 20% off for 100K+ images/month |
laozhang.ai | $0.01 per generation | $0.025 per generation | 30% off for 50K+ images/month |
Enterprise Solutions | Custom pricing | Custom pricing | Volume-based pricing available |
Python Integration Example
This complete Python example demonstrates how to integrate Sora Image API with error handling and image saving:
import requests
import os
import base64
from PIL import Image
from io import BytesIO
def generate_sora_image(api_key, prompt, output_path="sora_output.png"):
"""
Generate an image using Sora Image API and save to file
Args:
api_key (str): Your API key
prompt (str): Text description of the image to generate
output_path (str): Path to save the generated image
Returns:
bool: True if successful, False otherwise
"""
url = "https://api.laozhang.ai/v1/chat/completions"
payload = {
"model": "sora-image",
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": prompt
}
]
}
]
}
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}"
}
try:
# Make the API request
response = requests.post(url, json=payload, headers=headers)
response.raise_for_status()
# Extract the image data from the response
response_data = response.json()
image_data = response_data['choices'][0]['message']['content'][0]['image_url']
# The image URL might be returned directly or as a base64 string
if image_data.startswith('http'):
img_response = requests.get(image_data)
img_response.raise_for_status()
img = Image.open(BytesIO(img_response.content))
else:
# Remove base64 prefix if present
if 'base64,' in image_data:
image_data = image_data.split('base64,')[1]
img = Image.open(BytesIO(base64.b64decode(image_data)))
# Save the image
img.save(output_path)
print(f"Image successfully generated and saved to {output_path}")
return True
except requests.exceptions.RequestException as e:
print(f"API request error: {e}")
return False
except Exception as e:
print(f"Error processing image: {e}")
return False
# Example usage
if __name__ == "__main__":
API_KEY = os.environ.get("LAOZHANG_API_KEY")
if not API_KEY:
print("Please set the LAOZHANG_API_KEY environment variable")
else:
prompt = """
A photorealistic image of a coastal city in the year 2150,
with sustainable architecture integrated with nature,
flying electric vehicles, and ocean cleanup technology visible in the harbor.
The image should show a sunny day with people enjoying the advanced urban environment.
"""
generate_sora_image(API_KEY, prompt, "future_city.png")
Advanced Prompt Engineering for Sora Image API
The quality and accuracy of generated images heavily depend on how prompts are structured. Here are five advanced techniques for optimal results:
1. Detailed Visual Specifications
// Basic prompt
"A mountain landscape"
// Advanced prompt with visual specifications
"A breathtaking mountain landscape with snow-capped peaks, dense pine forests at mid-elevation, crystal-clear lake reflecting the mountains, golden hour lighting creating long shadows, wispy clouds in a vibrant blue sky, 8K resolution, photorealistic quality"
2. Camera and Perspective Technical Details
// Basic prompt
"A coffee shop"
// Advanced prompt with camera specifications
"Interior of a cozy coffee shop, shot with Sony Alpha camera, 35mm lens, f/2.8 aperture, warm natural lighting from large windows to the left, shallow depth of field focusing on a latte art cup in the foreground with a blurred barista working in the background, soft bokeh effect, photorealistic style"
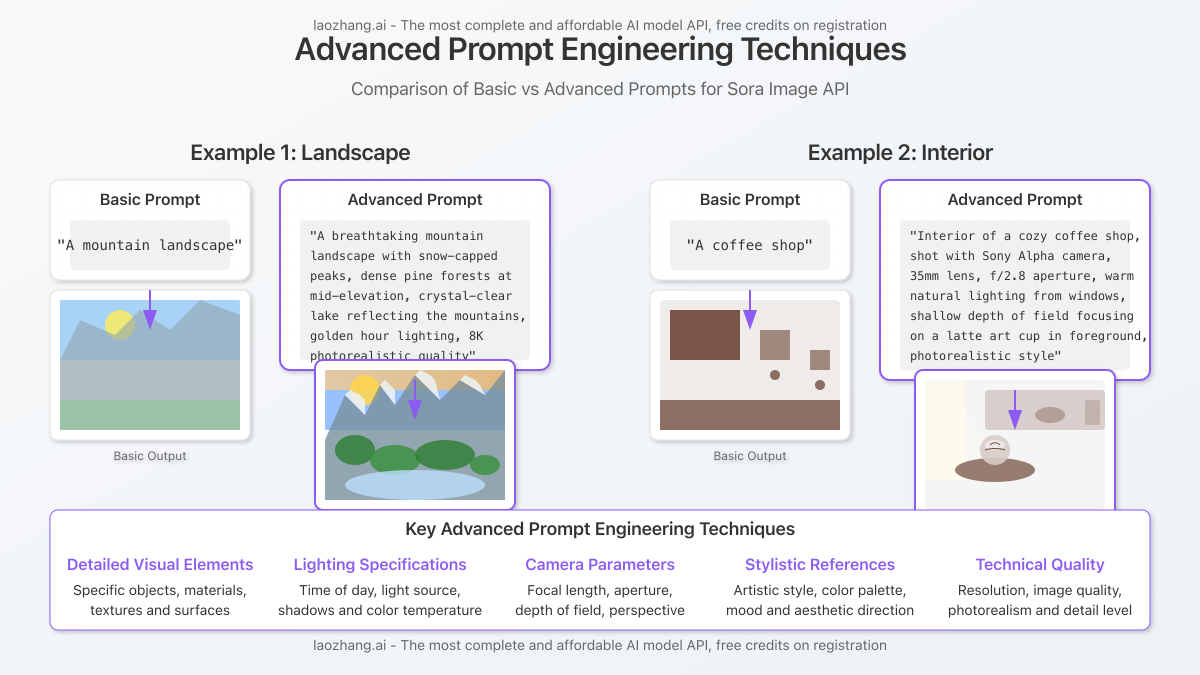
3. Negative Prompting
Specify what you don’t want in the image for better results:
// Using negative prompting
"A modern kitchen interior with marble countertops, professional appliances, and minimalist design. [negative prompt: cluttered, dirty, broken appliances, people, unrealistic proportions, poor lighting]"
4. Style Referencing and Art Direction
// Style referencing prompt
"Create an image of a futuristic robot in the distinct style of HR Giger, with biomechanical details, monochromatic palette favoring cold blues and metallic tones, intricate textures, high contrast lighting reminiscent of his Alien concept art, hyper-detailed mechanical and organic hybrid elements"
5. Emotional and Atmospheric Context
// Emotional context prompt
"A single chair in an abandoned theater, conveying profound loneliness and the passage of time. Dust particles visible in shafts of light from broken windows, faded red velvet upholstery, partially collapsed ceiling revealing night sky, cool moonlight contrasting with warm remnants of stage lights, evoke melancholy and nostalgia, 4K cinematic quality"
Common Errors and Troubleshooting
Rate Limit Exceeded: If you encounter rate limit errors, implement exponential backoff in your API calls. Start with a 1-second delay and double it with each retry, up to a maximum of 32 seconds.
Common Error Codes
Error Code | Description | Solution |
---|---|---|
401 | Authentication Error | Check API key validity and permissions |
429 | Rate Limit Exceeded | Implement request throttling and backoff strategy |
400 | Invalid Request | Check request parameters (prompt length, format, etc.) |
500/503 | Server Error | Retry request with exponential backoff |
Deployment Tip: For production applications, implement a queue system to manage Sora API requests and avoid hitting rate limits. Tools like Redis Queue or Amazon SQS can help manage high-volume requests effectively.
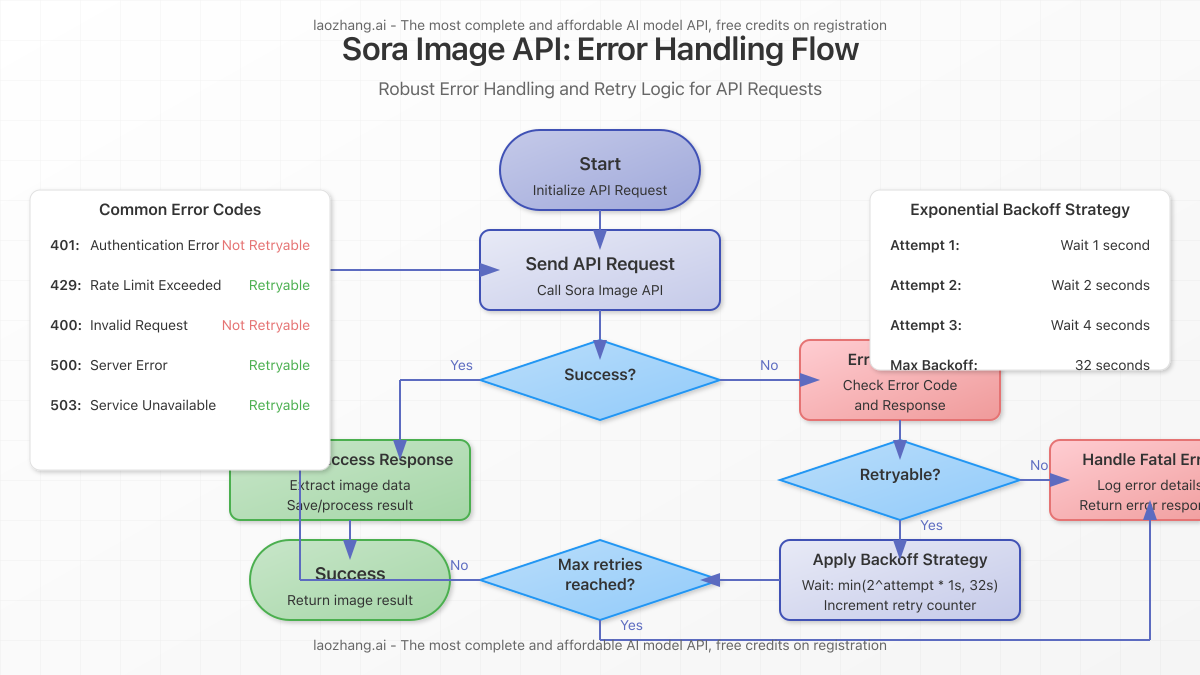
Cost Optimization Strategies
Implement these five strategies to reduce costs when using Sora Image API:
- Batching Requests: Group related image generation tasks to minimize API calls
- Request Caching: Cache common image outputs for frequently used prompts
- Resolution Optimization: Generate at lower resolutions for thumbnails and previews
- Content Filtering: Pre-validate prompts against content policy to avoid wasted calls
- Reseller Selection: Use cost-effective API resellers like laozhang.ai that offer up to 75% cost savings compared to direct API access
Case Study: E-commerce Product Visualization
Furniture retailer HomeDesign implemented Sora Image API to generate photorealistic product images in custom environments, reducing their product photography costs by 63% while increasing customer engagement:
// Node.js implementation for e-commerce product visualization
const express = require('express');
const axios = require('axios');
const app = express();
app.use(express.json());
// Product visualization endpoint
app.post('/api/visualize-product', async (req, res) => {
const { product, environment, style } = req.body;
// Construct detailed prompt
const prompt = `A photorealistic image of a ${product.color} ${product.name}
(${product.description}) placed in a ${environment.type} with
${environment.lighting} lighting. The room has ${environment.features}.
The style should be ${style}. 8K resolution, professional product
photography quality.`;
try {
// Call Sora Image API through laozhang.ai
const response = await axios.post(
'https://api.laozhang.ai/v1/chat/completions',
{
model: "sora-image",
messages: [
{
role: "user",
content: [{ type: "text", text: prompt }]
}
]
},
{
headers: {
'Authorization': `Bearer ${process.env.API_KEY}`,
'Content-Type': 'application/json'
}
}
);
const imageData = response.data.choices[0].message.content[0].image_url;
res.json({ success: true, image_url: imageData });
} catch (error) {
console.error('Visualization error:', error);
res.status(500).json({ success: false, error: 'Image generation failed' });
}
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
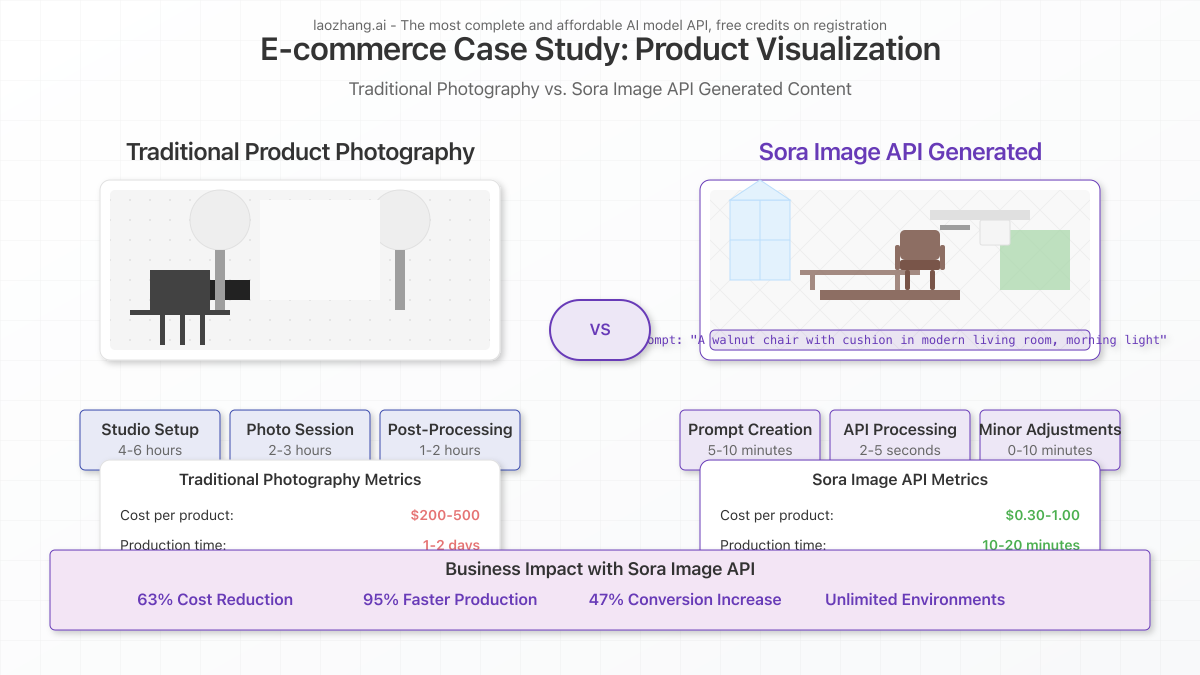
Security and Ethical Considerations
When implementing Sora Image API, address these key security and ethical concerns:
- Prompt Injection Prevention: Sanitize user inputs to prevent malicious prompt engineering
- Content Moderation: Implement pre-filtering of prompts against harmful content
- Attribution: Clearly label AI-generated images in user-facing applications
- Bias Mitigation: Regularly audit generated images for representation biases
- Data Privacy: Avoid storing sensitive prompts that might contain personal information
Future Developments
The Sora Image API ecosystem continues to evolve with several upcoming features anticipated in late 2025:
- Image-to-Image Editing: Modify existing images using text instructions
- Real-time Generation: Sub-second image generation for interactive applications
- 3D Asset Generation: Creating 3D models from text prompts
- Advanced Style Transfer: More precise control over artistic styles
- Enhanced Resolution: Support for 8K image generation
Conclusion
The Sora Image API represents a significant advancement in AI image generation, offering unprecedented photorealism and creative control. Whether accessed directly through OpenAI or via cost-effective resellers like laozhang.ai, developers now have the tools to implement this technology across various applications. By following the implementation examples, prompt engineering techniques, and optimization strategies outlined in this guide, you can effectively harness this powerful technology while managing costs and addressing ethical considerations.
Getting Started
To begin working with Sora Image API today, register for laozhang.ai’s services at https://api.laozhang.ai/register/?aff_code=JnIT and receive free starting credits to experiment with this groundbreaking technology.
Frequently Asked Questions
How does Sora Image API differ from DALL-E 3?
Sora Image API builds on the same diffusion technology as DALL-E 3 but offers significantly higher photorealism, better text rendering, and more accurate spatial relationships between objects. It can generate more complex scenes with multiple subjects while maintaining physical accuracy.
What are the content policy restrictions?
Sora Image API prohibits generating violent, sexually explicit, hateful, or harmful content. It also restricts the generation of images depicting real individuals without their consent, particularly public figures or celebrities.
Can I fine-tune Sora for my specific use case?
Currently, fine-tuning capabilities are limited to enterprise customers. However, advanced prompt engineering can achieve highly specific results without model customization.
How can I ensure consistent style across multiple image generations?
Include detailed style specifications in your prompts and use style reference examples. For large-scale applications, maintain a prompt library with standardized style descriptions.
Is there an offline version of Sora Image API available?
No, Sora Image API currently operates exclusively as a cloud-based service. On-premise solutions may become available for enterprise customers in the future.
How does the pricing compare to other image generation APIs?
Sora Image API through resellers like laozhang.ai offers competitive pricing at approximately $0.01 per standard image, making it 75-80% less expensive than some competing high-quality image generation services.
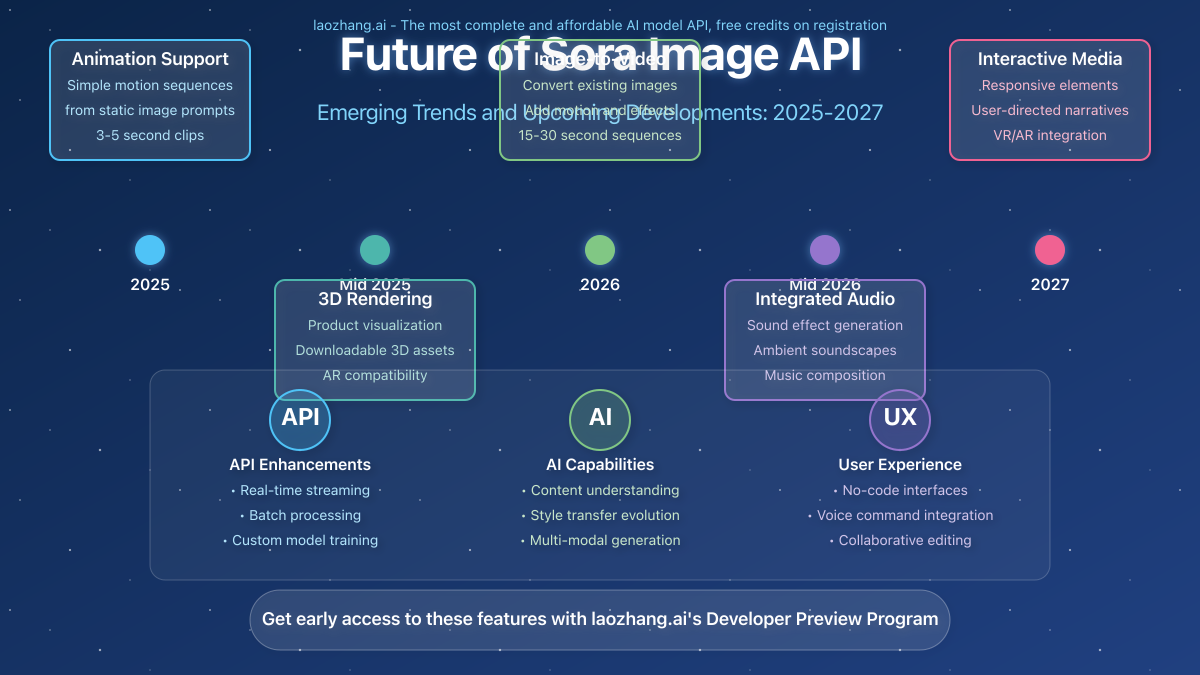