GPT-4o Image Generation API: The Ultimate Guide 2025 [8 Pro Applications]
OpenAI’s GPT-4o represents a revolutionary leap in multimodal AI, combining powerful text understanding with exceptional image generation capabilities. This comprehensive guide explores everything developers need to know about the GPT-4o image generation API – from basic implementation to advanced optimization techniques and professional applications.
Unlike previous models, GPT-4o delivers unprecedented image quality, text rendering accuracy, and a conversational interface that makes complex image creation accessible to everyone. Whether you’re building commercial applications, creative tools, or educational resources, this guide will help you harness the full potential of GPT-4o’s image capabilities.
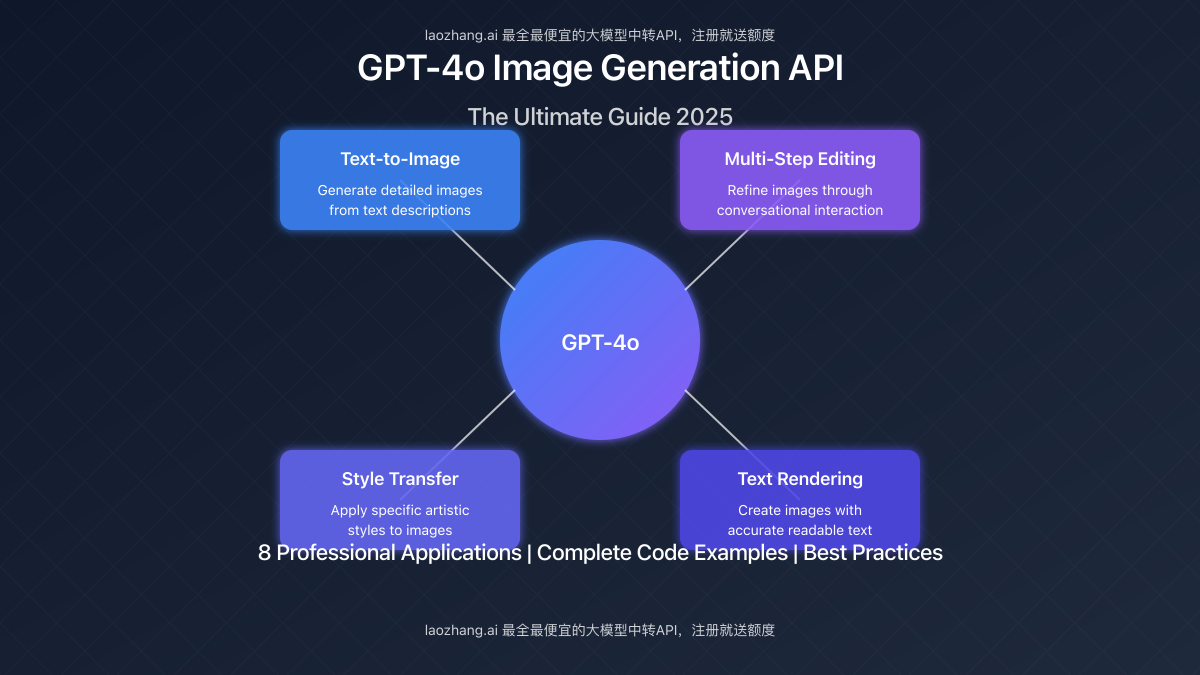
What is the GPT-4o Image Generation API?
GPT-4o (“o” for “omni”) is OpenAI’s flagship multimodal AI model launched in March 2025. The image generation component of GPT-4o enables developers to programmatically create high-quality images from text descriptions with unprecedented accuracy and creative potential.
This API represents a significant advancement over previous image generation models, combining deep visual understanding with the ability to generate images that accurately reflect complex text prompts, including proper text rendering within images.
Core Capabilities of GPT-4o Image API
- High-Fidelity Text-to-Image Conversion: Generate detailed, coherent images from text descriptions
- Multi-Step Image Editing: Progressively refine images through conversational interaction
- Accurate Text Rendering: Create images containing readable text with minimal errors
- Style Transfer: Apply specific artistic styles to generated images
- Image Variations: Generate multiple creative interpretations of the same concept
GPT-4o vs. Other Image Generation Models
Feature | GPT-4o | DALL-E 3 | Midjourney | Claude 3 |
---|---|---|---|---|
Text Rendering Accuracy | ★★★★★ | ★★★☆☆ | ★★☆☆☆ | ★★★☆☆ |
Image Understanding | ★★★★★ | Not Supported | Not Supported | ★★★★☆ |
Generation Speed | ★★★★☆ | ★★★☆☆ | ★★★★☆ | ★★★☆☆ |
Multi-step Editing | ★★★★★ | ★★☆☆☆ | ★★★☆☆ | ★★☆☆☆ |
API Integration Ease | ★★★★★ | ★★★★☆ | ★★☆☆☆ | ★★★★☆ |
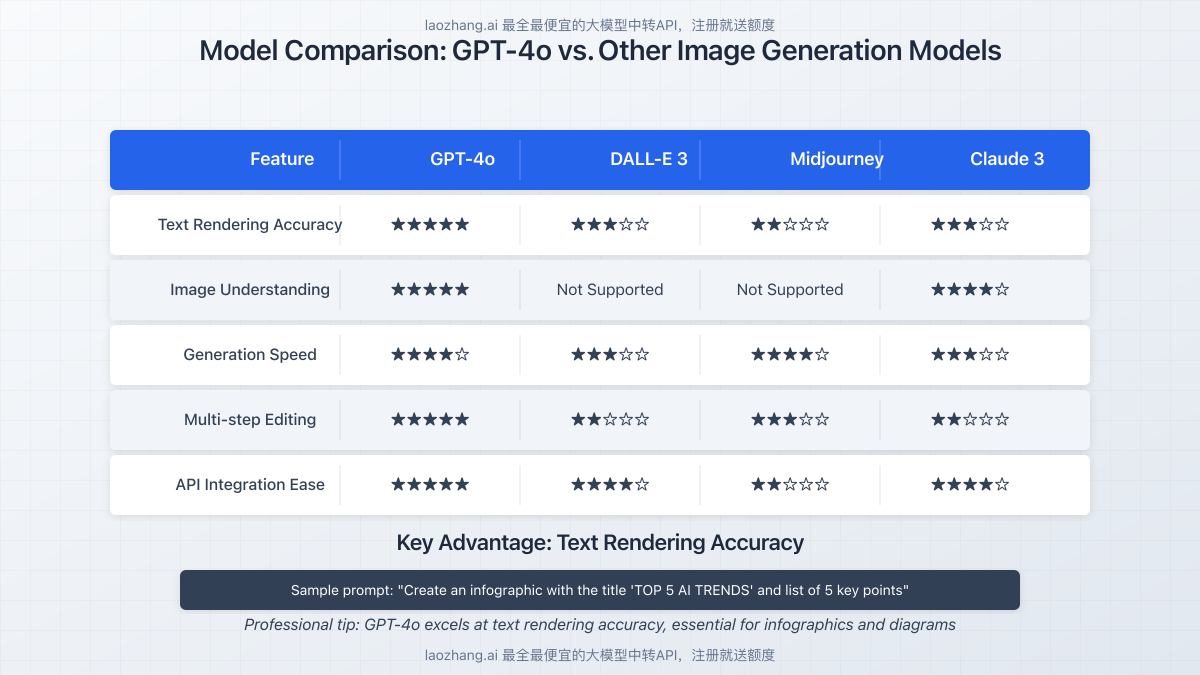
Getting Started with GPT-4o Image API
Before you can start generating images with GPT-4o, you’ll need to set up your development environment and obtain API access. This section walks through the complete setup process.
API Access Options
There are two primary ways to access the GPT-4o image API:
1. Direct OpenAI API Access
For users in regions with unrestricted access to OpenAI services:
- Create an OpenAI account at openai.com
- Navigate to the API section and complete identity verification
- Generate an API key from your account dashboard
- Add funds to your account to cover API usage
2. laozhang.ai API Proxy Service
For developers in regions with restricted access or those seeking enhanced performance:
- Register for an account at api.laozhang.ai
- Obtain your dedicated API key from the dashboard
- Add credits to your account through available payment methods
- Configure your code to use the laozhang.ai endpoint
Why Choose laozhang.ai Proxy?
- Stable connectivity in regions with access restrictions
- Up to 60% faster response times compared to direct API access
- Simplified billing and comprehensive usage statistics
- Unified access to multiple AI models through a single API
- Full compatibility with the official OpenAI SDK
Setting Up Your Development Environment
Follow these steps to set up your Python environment for using the GPT-4o image API:
# Create and activate a virtual environment
python -m venv gpt4o-env
source gpt4o-env/bin/activate # On Windows: gpt4o-env\Scripts\activate
# Install required packages
pip install openai pillow matplotlib numpy
# Set up your API key
# For direct OpenAI access:
export OPENAI_API_KEY='your-openai-api-key'
# For laozhang.ai proxy:
export OPENAI_API_KEY='your-laozhang-api-key'
export OPENAI_BASE_URL='https://api.laozhang.ai/v1'
Basic API Test
Verify your setup with this simple test that checks connectivity to the GPT-4o API:
import openai
# Initialize the client
client = openai.OpenAI(
api_key="your-api-key", # Your API key here
base_url="https://api.laozhang.ai/v1" # Remove this line if using OpenAI directly
)
# Simple test request
response = client.chat.completions.create(
model="gpt-4o",
messages=[{"role": "user", "content": "What can your image generation capabilities do?"}]
)
print(response.choices[0].message.content)
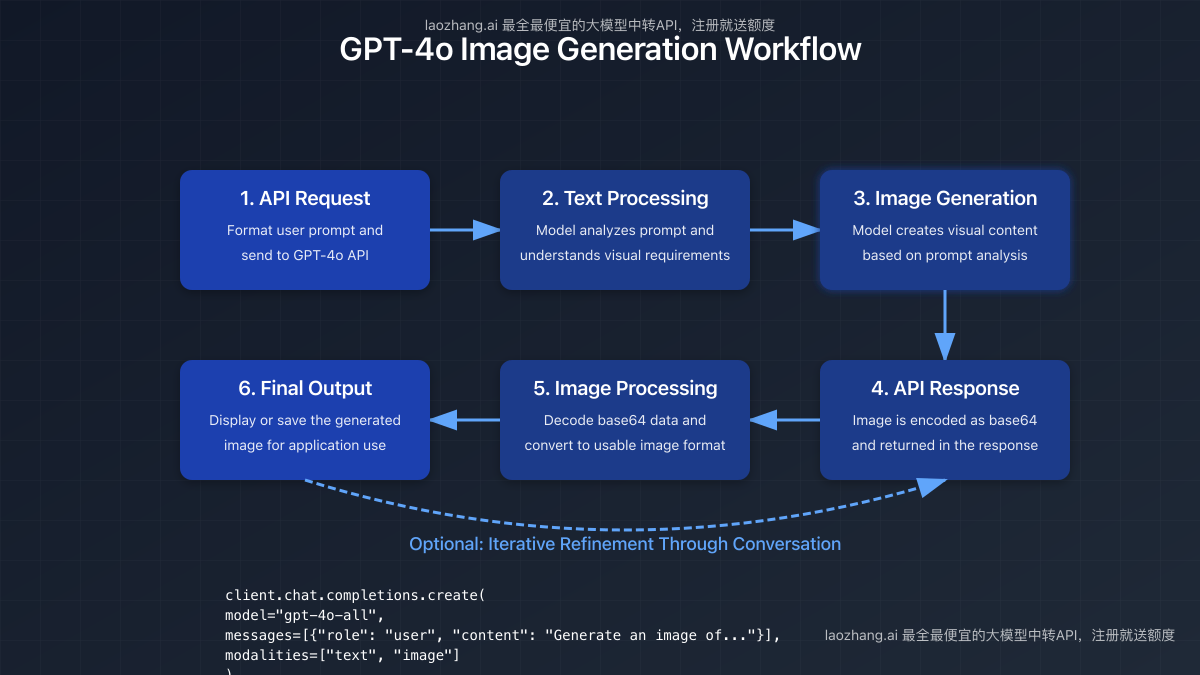
Basic Image Generation Techniques
Now that your environment is set up, let’s explore the fundamental techniques for generating images with GPT-4o.
Simple Text-to-Image Conversion
The most basic use of GPT-4o’s image generation is converting text descriptions into images:
import openai
import base64
import io
from PIL import Image
import matplotlib.pyplot as plt
# Initialize the client (using laozhang.ai proxy)
client = openai.OpenAI(
api_key="your-laozhang-api-key", # Replace with your actual API key
base_url="https://api.laozhang.ai/v1" # Remove this line if using OpenAI directly
)
def generate_image_from_text(prompt):
"""Generate an image from a text prompt using GPT-4o"""
try:
# Send request to the GPT-4o model
response = client.chat.completions.create(
model="gpt-4o-all", # The image-capable model
messages=[
{"role": "system", "content": "You are an expert image creator. Generate high-quality images based on user descriptions."},
{"role": "user", "content": prompt}
],
modalities=["text", "image"], # Enable image generation
max_tokens=1000
)
# Extract image from response
for content in response.choices[0].message.content:
if hasattr(content, 'image_url') and content.image_url:
# Extract base64 data after the prefix
base64_data = content.image_url.split(',')[1]
# Decode base64 to image
image_data = base64.b64decode(base64_data)
image = Image.open(io.BytesIO(image_data))
return image
# If no image found in response
return None
except Exception as e:
print(f"Error generating image: {e}")
return None
# Example usage
prompt = "A futuristic skyscraper with hanging gardens and flying vehicles around it, photorealistic style"
image = generate_image_from_text(prompt)
if image:
# Display the image
plt.figure(figsize=(10, 10))
plt.imshow(image)
plt.axis('off')
plt.show()
# Save the image
image.save("futuristic_skyscraper.png")
print("Image generated and saved successfully!")
else:
print("Failed to generate image")
Prompt Engineering for Better Results
The quality of your prompt significantly impacts the generated image. Here are key elements to include in effective prompts:
- Subject Description: Clearly define the main elements you want in the image
- Style Specification: Indicate the artistic style (e.g., “photorealistic,” “watercolor painting,” “3D render”)
- Composition Details: Describe the arrangement, perspective, and framing
- Lighting and Atmosphere: Specify lighting conditions, time of day, and mood
- Technical Parameters: Include terms like “high resolution” or “detailed” if desired
Example of a Well-Crafted Prompt:
“Create a photorealistic image of a modern minimalist living room with floor-to-ceiling windows overlooking a mountain landscape at sunset. The room features a gray sectional sofa, a glass coffee table, and a few potted plants. Natural light is streaming in, creating long shadows on the polished concrete floor. Use a warm color palette with accents of teal.”
Using System Messages to Guide Image Creation
The system message can significantly influence the style and approach of the generated image:
# Example with specialized system message
architectural_system_message = """You are an expert architectural visualization artist.
Create highly detailed, professional architectural imagery with accurate proportions,
lighting, and materials. Pay close attention to spatial relationships, scale, and
architectural details. Use realistic lighting and shadows to enhance depth."""
response = client.chat.completions.create(
model="gpt-4o-all",
messages=[
{"role": "system", "content": architectural_system_message},
{"role": "user", "content": "An elegant modern house with a cantilevered second floor over a reflective pool"}
],
modalities=["text", "image"]
)
# Process response...
Advanced Image Generation Techniques
GPT-4o’s image capabilities extend far beyond basic text-to-image conversion. This section explores advanced techniques that set GPT-4o apart from other image generation models.
Multi-Step Image Refinement
One of GPT-4o’s most powerful features is its ability to iteratively refine images through conversation:
# Function for multi-step image editing
def refine_image(initial_prompt, refinement_instructions, base64_image=None):
"""Refine an image through conversational interaction"""
messages = [
{"role": "system", "content": "You are an expert image editor who can make precise adjustments to images."}
]
if base64_image:
# If we're starting with an existing image
messages.extend([
{"role": "user", "content": initial_prompt},
{"role": "assistant", "content": [
{"type": "text", "text": "Here's the image:"},
{"type": "image_url", "image_url": {"url": f"data:image/png;base64,{base64_image}"}}
]},
{"role": "user", "content": refinement_instructions}
])
else:
# Generate initial image from scratch
messages.append({"role": "user", "content": initial_prompt})
initial_response = client.chat.completions.create(
model="gpt-4o-all",
messages=messages,
modalities=["text", "image"]
)
# Extract the initial image and add to conversation
# (Code to extract base64 image from response)
messages.extend([
{"role": "assistant", "content": [
{"type": "text", "text": "Here's the initial image:"},
{"type": "image_url", "image_url": {"url": f"data:image/png;base64,{extracted_base64}"}}
]},
{"role": "user", "content": refinement_instructions}
])
# Generate refined image
refined_response = client.chat.completions.create(
model="gpt-4o-all",
messages=messages,
modalities=["text", "image"]
)
# Extract and return refined image
# (Code to extract and return the refined image)
# Example usage
initial_prompt = "A coastal beach house with large windows"
refinement = "Make it sunset with dramatic orange and purple sky, add some palm trees"
refined_image = refine_image(initial_prompt, refinement)
Creating Infographics with Text
GPT-4o excels at generating images containing accurate text, making it ideal for creating infographics and diagrams:
infographic_prompt = """Create a clean, professional infographic about 'The 5 Steps of Machine Learning' with:
1. A numbered flow diagram showing: Data Collection → Data Preparation → Model Training → Model Evaluation → Deployment
2. Brief bullet points (2-3) explaining each step
3. Simple iconic representations for each step
4. Professional blue and gray color scheme
5. Clean, modern sans-serif fonts
6. The title 'THE MACHINE LEARNING PROCESS' at the top"""
infographic = generate_image_from_text(infographic_prompt)
Applying Artistic Styles
GPT-4o can apply specific artistic styles to create visually distinctive images:
# Function to apply artistic styles to image concepts
def generate_styled_image(subject, style):
"""Generate an image with a specific artistic style"""
style_descriptions = {
"van_gogh": "in the distinctive style of Van Gogh's 'Starry Night' with swirling, textured brushstrokes and bold colors",
"cyberpunk": "in cyberpunk style with neon lights, high contrast, urban futuristic elements, and a dark atmosphere",
"watercolor": "as a delicate watercolor painting with soft edges, translucent colors, and visible paper texture",
"anime": "in anime style with clean lines, expressive features, and vibrant colors"
}
style_desc = style_descriptions.get(style, "")
prompt = f"Create an image of {subject} {style_desc}"
return generate_image_from_text(prompt)
# Example usage
lighthouse_van_gogh = generate_styled_image("a coastal lighthouse at night", "van_gogh")
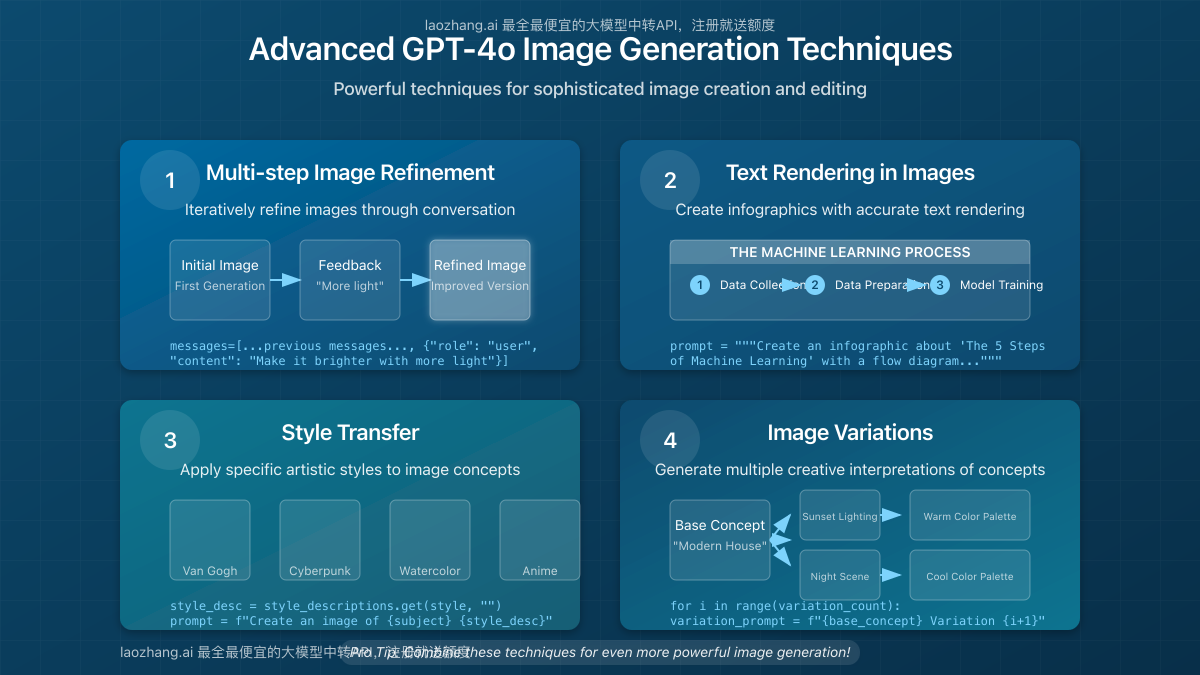
8 Professional Applications of GPT-4o Image API
The GPT-4o image API opens up numerous possibilities for commercial applications. Here are eight powerful use cases with implementation guidance.
1. E-commerce Product Visualization
Generate custom product images based on configuration options:
def generate_product_visualization(product_type, color, material, background):
"""Generate custom product visualization for e-commerce"""
prompt = f"""Create a professional product image of a {color} {product_type} made of {material}.
Show the product against a {background} background with professional studio lighting and subtle shadows.
The image should be photorealistic, high-detail, and suitable for an e-commerce website."""
return generate_image_from_text(prompt)
# Example: Generate a customized furniture visualization
chair_image = generate_product_visualization(
product_type="ergonomic office chair",
color="navy blue",
material="premium mesh and chrome",
background="minimal white"
)
2. Real Estate Virtual Staging
Transform empty property images with virtual staging:
def virtually_stage_property(property_type, room_type, style):
"""Virtually stage an empty property image"""
prompt = f"""Create a professionally staged image of an empty {property_type} {room_type}
decorated in {style} style. Include appropriate furniture, decor, and lighting to make
the space look inviting and showcase its potential. The staging should be realistic and
tasteful, suitable for a real estate listing."""
return generate_image_from_text(prompt)
# Example: Stage an empty apartment living room
staged_image = virtually_stage_property(
property_type="apartment",
room_type="living room",
style="modern minimalist"
)
3. Marketing Campaign Visuals
Generate consistent marketing visuals across campaigns:
def create_marketing_visual(product_name, campaign_theme, audience, message):
"""Create marketing campaign visuals"""
prompt = f"""Create a marketing image for {product_name} targeting {audience}.
The visual should incorporate the campaign theme of '{campaign_theme}'
and communicate the message: '{message}'.
The image should be eye-catching, professional, and aligned with contemporary marketing aesthetics."""
return generate_image_from_text(prompt)
# Example: Create a marketing visual for a fitness app
fitness_app_visual = create_marketing_visual(
product_name="FitTrack Pro fitness app",
campaign_theme="Transform Your Life, One Step at a Time",
audience="health-conscious professionals aged 30-45",
message="Achieve your fitness goals with personalized AI coaching"
)
4. Educational Content Illustration
Create custom illustrations for educational materials:
def generate_educational_illustration(subject, concept, age_group):
"""Generate educational illustrations for specific age groups"""
prompt = f"""Create an educational illustration explaining '{concept}' for {age_group} students
studying {subject}. The image should be clear, informative, and engaging, with appropriate
labels and visual explanations. Use a color scheme and style appropriate for the age group."""
return generate_image_from_text(prompt)
# Example: Illustrate the water cycle for elementary students
water_cycle_illustration = generate_educational_illustration(
subject="environmental science",
concept="the water cycle process showing evaporation, condensation, precipitation, and collection",
age_group="elementary school (ages 8-10)"
)
5. UI/UX Design Mockups
Generate interface mockups for digital products:
def create_ui_mockup(app_type, screen_type, style, color_scheme):
"""Generate UI mockups for app development"""
prompt = f"""Create a UI mockup for a {app_type} app's {screen_type} screen.
The design should follow {style} design principles with a {color_scheme} color scheme.
Include realistic interface elements, content, and appropriate layout.
The mockup should look professional and contemporary."""
return generate_image_from_text(prompt)
# Example: Generate a fitness app dashboard mockup
dashboard_mockup = create_ui_mockup(
app_type="fitness tracking",
screen_type="user dashboard",
style="clean, minimal",
color_scheme="blue and white with orange accents"
)
6. Custom Publication Illustrations
Generate tailored illustrations for articles, books, or blogs:
def generate_publication_illustration(publication_type, topic, style, mood):
"""Create custom illustrations for publications"""
prompt = f"""Create a {style} illustration for a {publication_type} about '{topic}'.
The image should evoke a {mood} mood and be suitable for professional publication.
The illustration should be conceptually relevant to the topic while being visually engaging."""
return generate_image_from_text(prompt)
# Example: Create an illustration for a technology blog post
tech_illustration = generate_publication_illustration(
publication_type="blog post",
topic="The future of artificial intelligence in healthcare",
style="digital minimalist",
mood="innovative and hopeful"
)
7. Social Media Content Creation
Generate tailored social media visuals:
def create_social_media_content(platform, content_type, brand_name, message, style):
"""Generate platform-specific social media content"""
prompt = f"""Create a {content_type} for {brand_name} to be posted on {platform}.
The image should communicate: '{message}'.
Use a {style} visual style that will stand out in a social media feed.
The design should be optimized for the specific platform with appropriate spacing and composition."""
return generate_image_from_text(prompt)
# Example: Create an Instagram post for a coffee brand
instagram_post = create_social_media_content(
platform="Instagram",
content_type="promotional post",
brand_name="Mountain Peak Coffee",
message="Start your morning adventure with our new Alpine Blend",
style="warm, lifestyle photography"
)
8. Product Concept Visualization
Visualize product concepts during development:
def visualize_product_concept(product_type, key_features, design_aesthetic, usage_scenario):
"""Visualize product concepts during development"""
prompt = f"""Create a product concept visualization for a {product_type} with these key features:
{key_features}. The design should follow a {design_aesthetic} aesthetic.
Show the product being used in a {usage_scenario} setting.
The visualization should look like a professional concept rendering with attention to detail and realism."""
return generate_image_from_text(prompt)
# Example: Visualize a smart home device concept
smart_home_concept = visualize_product_concept(
product_type="smart home hub device",
key_features="touchscreen interface, voice control capability, compact cylindrical design, ambient light indicators",
design_aesthetic="modern, minimalist",
usage_scenario="contemporary living room"
)
Cost Optimization and Best Practices
Maximize the value of your GPT-4o API usage with these optimization strategies and best practices.
API Pricing Understanding
GPT-4o image generation costs are determined by both input tokens (your prompt) and the complexity of the generated image. Current pricing as of April 2025:
- Input tokens: $5 per 1M tokens
- Output tokens (text): $15 per 1M tokens
- Image generation: Varies by resolution and quality, starting at approximately $0.04 per image
Cost Optimization Tips:
- Batch similar requests to maximize efficiency
- Implement caching to avoid regenerating identical content
- Use appropriate quality settings based on the application needs
- Craft concise but effective prompts to reduce token usage
- Implement exponential backoff for API rate limits
Error Handling and Reliability
Implement robust error handling to ensure your application remains stable:
def safe_image_generation(prompt, max_retries=3):
"""Generate an image with robust error handling"""
for attempt in range(max_retries):
try:
response = client.chat.completions.create(
model="gpt-4o-all",
messages=[{"role": "user", "content": prompt}],
modalities=["text", "image"]
)
# Process successful response
# ...
return image
except openai.APIError as e:
print(f"API error: {e}")
if attempt < max_retries - 1:
time.sleep(2 ** attempt) # Exponential backoff
else:
return {"error": "API error", "details": str(e)}
except openai.RateLimitError:
print("Rate limit exceeded")
if attempt < max_retries - 1:
time.sleep(5 + 5 * attempt) # Backoff with longer delays
else:
return {"error": "Rate limit", "details": "Maximum retries exceeded"}
except Exception as e:
print(f"Unexpected error: {e}")
return {"error": "Unexpected error", "details": str(e)}
return {"error": "Maximum retries exceeded"}
Content Policy Compliance
Ensure your image generation complies with OpenAI’s content policies:
- Implement pre-screening for prompt content
- Use system messages that guide toward policy-compliant outputs
- Implement human review for sensitive use cases
- Maintain audit logs of prompts and outputs
- Stay updated on OpenAI’s policy changes
Using laozhang.ai API Proxy Service
For users in regions with restricted access to OpenAI services or those seeking enhanced performance, laozhang.ai provides a reliable proxy service with full compatibility.
Integration Process
Integrating with the laozhang.ai proxy service is straightforward:
- Register for an account at api.laozhang.ai
- Navigate to your dashboard to obtain your API key
- Replace the OpenAI endpoint in your code with the laozhang.ai endpoint
Code Example
Here’s how to use the laozhang.ai proxy service with the OpenAI SDK:
import openai
# Initialize the client with laozhang.ai endpoint
client = openai.OpenAI(
api_key="your-laozhang-api-key", # Your laozhang.ai API key
base_url="https://api.laozhang.ai/v1" # laozhang.ai endpoint
)
# Use the client as you would with direct OpenAI access
response = client.chat.completions.create(
model="gpt-4o-all",
messages=[
{"role": "system", "content": "You are an expert image creator."},
{"role": "user", "content": "Generate a photorealistic image of a mountain landscape at sunset"}
],
modalities=["text", "image"]
)
# The rest of your code remains the same
curl Example
For testing or integration with other programming languages, you can use curl:
curl -X POST "https://api.laozhang.ai/v1/chat/completions" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "gpt-4o-all",
"messages": [
{
"role": "user",
"content": "Generate an image of three cats playing"
}
],
"modalities": ["text", "image"]
}'
Need Help with laozhang.ai Integration?
Contact laozhang.ai support via WeChat: ghj930213 for assistance with setup, billing, or technical questions.
Future Developments and Roadmap
The GPT-4o image API continues to evolve rapidly. Here’s what to watch for in upcoming updates:
Announced Upcoming Features
- Enhanced Resolution Options: Support for generating images at 1024×1024 resolution and beyond
- Video Generation: Capabilities for creating short video clips from text descriptions
- Precise Control Mechanisms: More granular control over specific elements in generated images
- Style Reference Images: Ability to use uploaded images as style references
- Domain-Specific Models: Specialized versions optimized for specific industries
Preparing for Future Capabilities
To position your implementation for upcoming features:
- Design flexible architectures that can adapt to new capabilities
- Implement feature flags to easily enable new features
- Collect data on which prompts work best for your specific use cases
- Stay updated through OpenAI’s announcements and developer forums
- Participate in early access programs when available
Conclusion: Unleashing Creative Potential
The GPT-4o image generation API represents a significant leap forward in AI-powered visual creation. By combining unprecedented text rendering accuracy, multimodal understanding, and intuitive conversational interactions, it opens up new possibilities for developers, designers, and businesses.
As you implement the techniques covered in this guide, remember these key takeaways:
- Prompt crafting is crucial for quality output – invest time in creating detailed, specific prompts
- Use the conversational capabilities to progressively refine images through multiple interactions
- Consider the wide range of applications from e-commerce to education and beyond
- Implement robust systems that integrate effectively with your existing workflows
- Stay adaptable as the technology continues to evolve rapidly
By mastering the GPT-4o image API, you position yourself at the forefront of the visual AI revolution, ready to create applications that blend human creativity with AI capabilities in previously impossible ways.
When working with the GPT-4o image API, focus on creating value through novel applications rather than simply replicating existing solutions. The true potential lies in building experiences that combine multiple modalities and context-aware interactions!