Last Updated: April 12, 2025 – Verified working methods
Claude 3.7 Sonnet represents the pinnacle of Anthropic’s AI technology, offering extraordinary reasoning, coding abilities, and multimodal understanding. However, direct API access through Anthropic comes with significant costs that can be prohibitive for individual developers and small teams. This guide unveils three legitimate methods to access Claude 3.7 API capabilities without spending a dollar or providing credit card details.
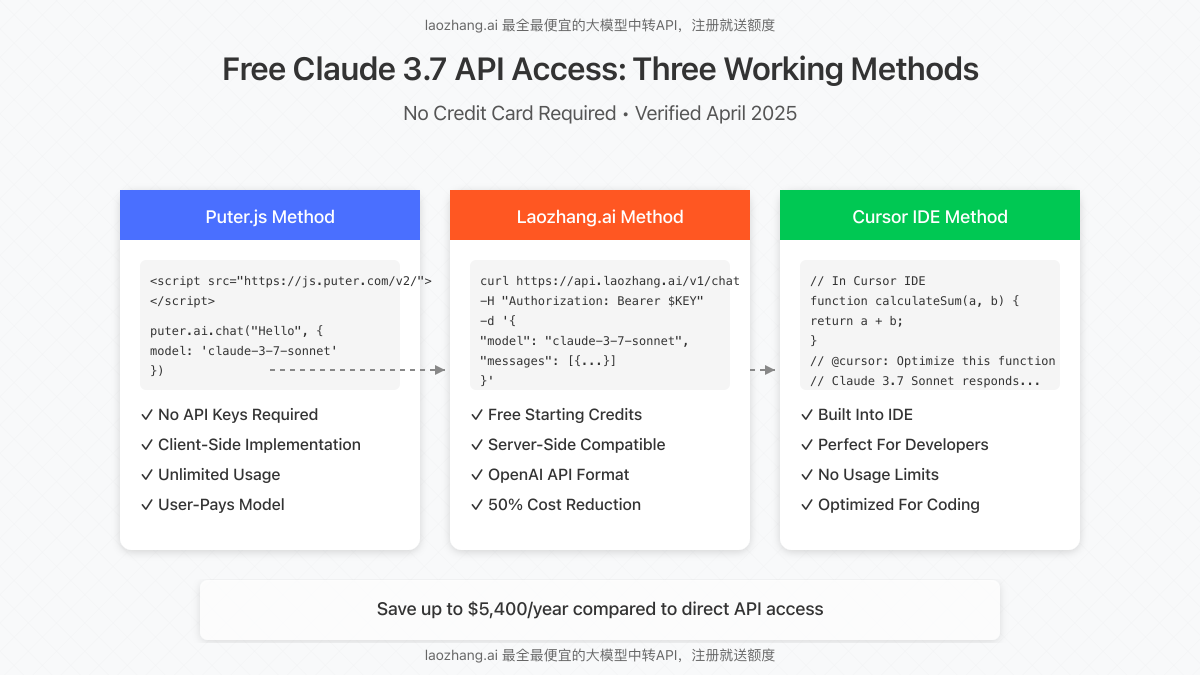
Why Claude 3.7 API Access Matters
Claude 3.7 Sonnet brings several breakthrough capabilities that make it particularly valuable for developers:
- Hybrid Reasoning System – The first model to offer both instant responses and visible step-by-step thinking processes
- Superior Code Generation – Exceptional performance on software engineering tasks, particularly debugging and complex system design
- Advanced Multimodal Processing – Can analyze images, charts, and documents alongside text with high comprehension
- 32K Token Context Window – Retains and processes substantial amounts of information in a single conversation
- High Accuracy Instruction Following – Precise execution of complex, multi-step instructions
However, these capabilities come with a significant cost barrier:
Access Method | Cost Structure | Limitations |
---|---|---|
Anthropic Direct API | $15/million input tokens, $75/million output tokens | Credit card required, monthly minimums |
AWS Claude API | $16.5/million input tokens, $82.5/million output tokens | AWS account setup required |
Azure Claude API | Starts at $17/million input tokens, $85/million output tokens | Enterprise commitments often required |
Fortunately, several workarounds exist that provide free access to Claude 3.7’s capabilities. Let’s explore each method with practical implementation steps.
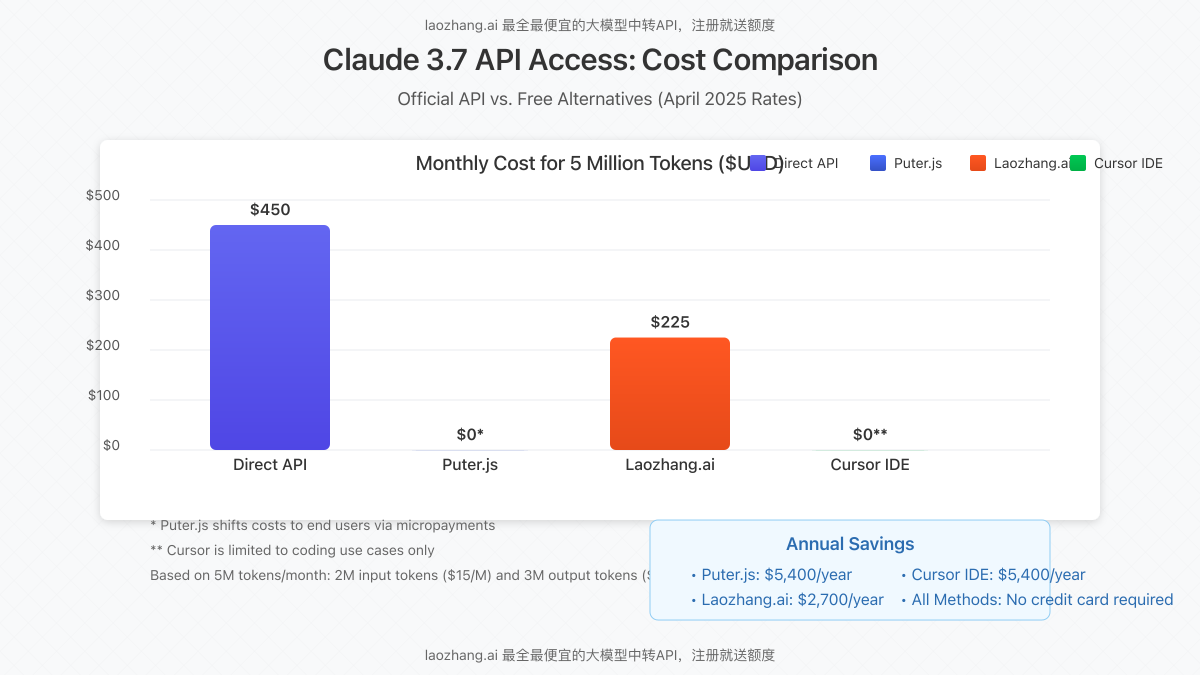
Method 1: Puter.js – Free, Unlimited, No-Key Solution
Puter.js offers perhaps the cleanest solution for accessing Claude 3.7 Sonnet capabilities without any API keys or usage restrictions. This service operates on a “User Pays” model – when your users interact with your application, they cover the compute costs directly.
How Puter.js Works: Rather than requiring developers to manage API keys and billing, Puter.js shifts the computation cost to the end user through micropayments handled in the background. This creates a truly free developer experience with no usage limits.
Implementation Steps:
- Include the Puter.js Script
<script src="https://js.puter.com/v2/"></script>
- Basic Text Generation
puter.ai.chat("Explain quantum computing in simple terms", {model: 'claude-3-7-sonnet'}) .then(response => { console.log(response.message.content[0].text); });
- Streaming for Longer Responses
async function streamClaudeResponse() { const response = await puter.ai.chat( "Write a detailed essay on the impact of AI", {model: 'claude-3-7-sonnet', stream: true} ); for await (const part of response) { console.log(part?.text); } } streamClaudeResponse();
Complete implementation in an HTML file:
<!DOCTYPE html>
<html>
<head>
<title>Claude 3.7 Sonnet Demo</title>
<script src="https://js.puter.com/v2/"></script>
</head>
<body>
<h1>Claude 3.7 Sonnet Response</h1>
<div id="response">Loading...</div>
<script>
async function getClaudeResponse() {
const responseDiv = document.getElementById('response');
try {
const response = await puter.ai.chat(
"Explain the key differences between Claude 3.7 and GPT-4o",
{model: 'claude-3-7-sonnet'}
);
responseDiv.innerHTML = response.message.content[0].text;
} catch (error) {
responseDiv.innerHTML = `Error: ${error.message}`;
}
}
getClaudeResponse();
</script>
</body>
</html>
Advantages:
- No API key management
- No usage quotas or limits
- No credit card required
- Simple JavaScript implementation
- Works with client-side only applications
Limitations:
- Client-side only (no server-side implementation)
- End users bear the computation costs
- Limited fine-tuning capabilities
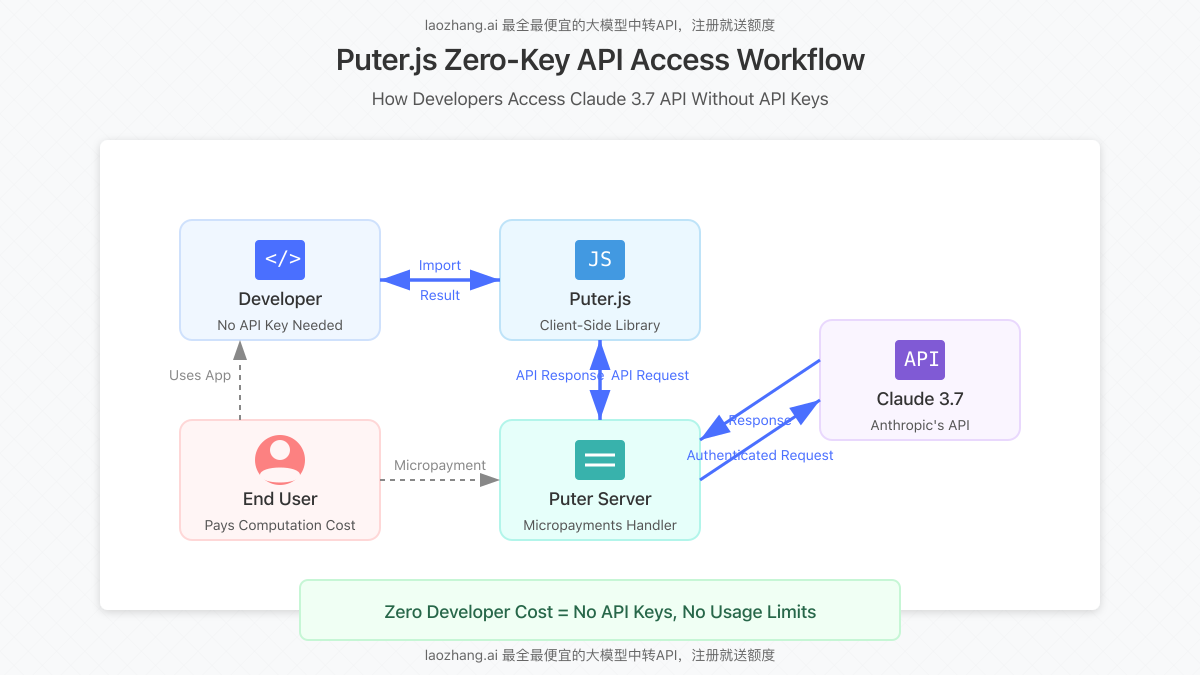
Method 2: Laozhang.ai – Free Credits & API Proxy Service
Laozhang.ai offers a compelling alternative for developers needing both client and server-side access to Claude 3.7 API. This service functions as a proxy to multiple AI providers, including Anthropic’s Claude models, with free credits upon registration and significantly reduced rates compared to direct API access.
Get Started with Laozhang.ai: Register here to receive free API credits and access to Claude 3.7 API.
Implementation Steps:
- Create an Account – Sign up using the link above to receive your free credits
- Get Your API Key – Available in your dashboard after registration
- Make API Calls – Use standard OpenAI-compatible API format with your key
Example API Request (cURL):
curl https://api.laozhang.ai/v1/chat/completions \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "claude-3-7-sonnet",
"stream": false,
"messages": [
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "Write a recursive function to calculate Fibonacci numbers in Python."}
]
}'
Node.js Implementation:
const axios = require('axios');
async function callClaude() {
try {
const response = await axios.post(
'https://api.laozhang.ai/v1/chat/completions',
{
model: 'claude-3-7-sonnet',
messages: [
{ role: 'system', content: 'You are a helpful assistant.' },
{ role: 'user', content: 'Explain quantum computing simply.' }
]
},
{
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${process.env.LAOZHANG_API_KEY}`
}
}
);
console.log(response.data.choices[0].message.content);
} catch (error) {
console.error('Error:', error.response ? error.response.data : error.message);
}
}
callClaude();
Python Implementation:
import requests
import os
api_key = os.getenv("LAOZHANG_API_KEY")
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}"
}
payload = {
"model": "claude-3-7-sonnet",
"messages": [
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "Explain quantum computing simply."}
]
}
response = requests.post(
"https://api.laozhang.ai/v1/chat/completions",
headers=headers,
json=payload
)
print(response.json()["choices"][0]["message"]["content"])
Service | Claude 3.7 Input Cost | Claude 3.7 Output Cost | Free Credits |
---|---|---|---|
Direct Anthropic API | $15 per million tokens | $75 per million tokens | Limited trial only |
Laozhang.ai | $7.5 per million tokens | $37.5 per million tokens | Yes, upon registration |
Cost comparison between direct Anthropic API and Laozhang.ai proxy service (April 2025 rates)
Advantages:
- 50% cost reduction compared to direct API
- Free starter credits
- Works with both client and server-side applications
- OpenAI-compatible API format
- Access to multiple models through one API
Limitations:
- Eventually requires payment after free credits
- Third-party dependency
- Potential for additional latency
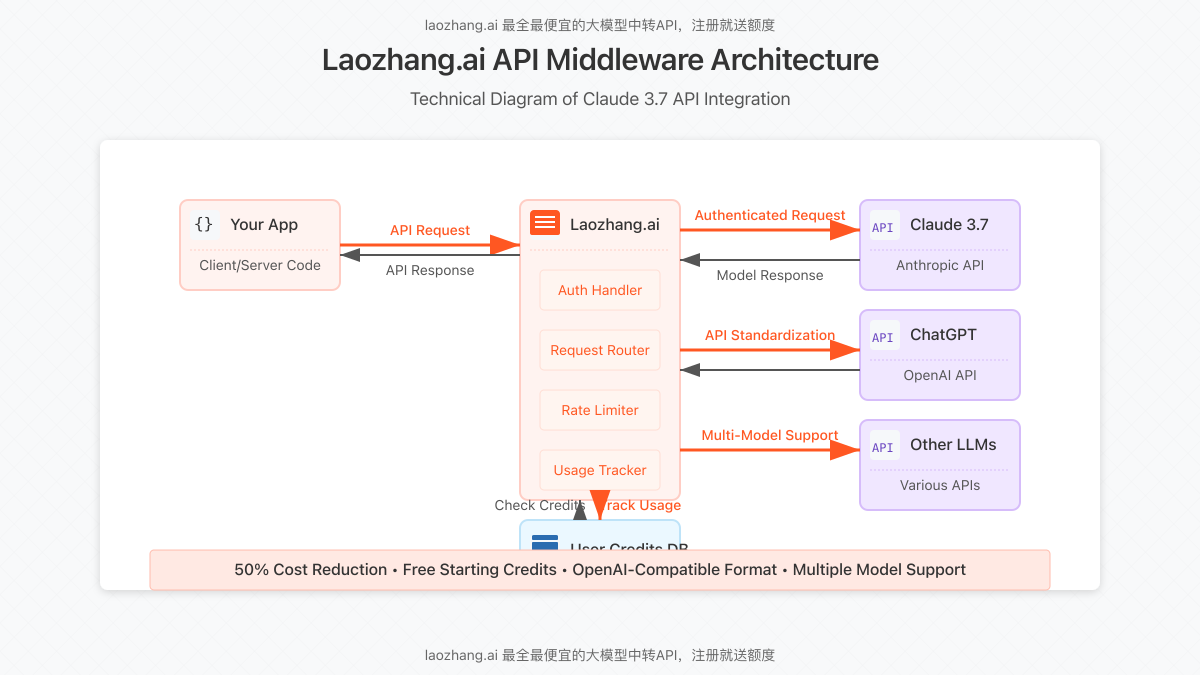
Method 3: Cursor IDE Integration – Developer-Focused Solution
For developers primarily focused on coding tasks, Cursor provides a specialized, free access path to Claude 3.7 Sonnet through its AI-powered code editor.
Implementation Steps:
- Download Cursor – Get the free IDE from cursor.sh
- Configure Claude 3.7 – In settings, select Claude 3.7 Sonnet as your LLM
- Access Through Coding Interface – Use commands or keyboard shortcuts for AI assistance
Cursor allows you to:
- Generate code with inline AI assistance
- Debug complex issues with Claude’s reasoning
- Refactor code through natural language instructions
- Explain code blocks and functions
- Design systems and architectures through prompting
Example Cursor Commands:
// Generate a React component
// @cursor: Create a user profile component with name, avatar and status indicator
// Explain complex code
// @cursor: Explain what this function does
// Debug issues
// @cursor: Debug why this API call fails with error 403
// Optimize algorithms
// @cursor: Optimize this sorting function for better performance
Advantages:
- Completely free to use
- Integrated development environment
- Optimized for coding tasks
- No token limits for development usage
- Regular updates with new features
Limitations:
- Limited to coding scenarios
- No direct API access for external applications
- Requires using Cursor as your development environment
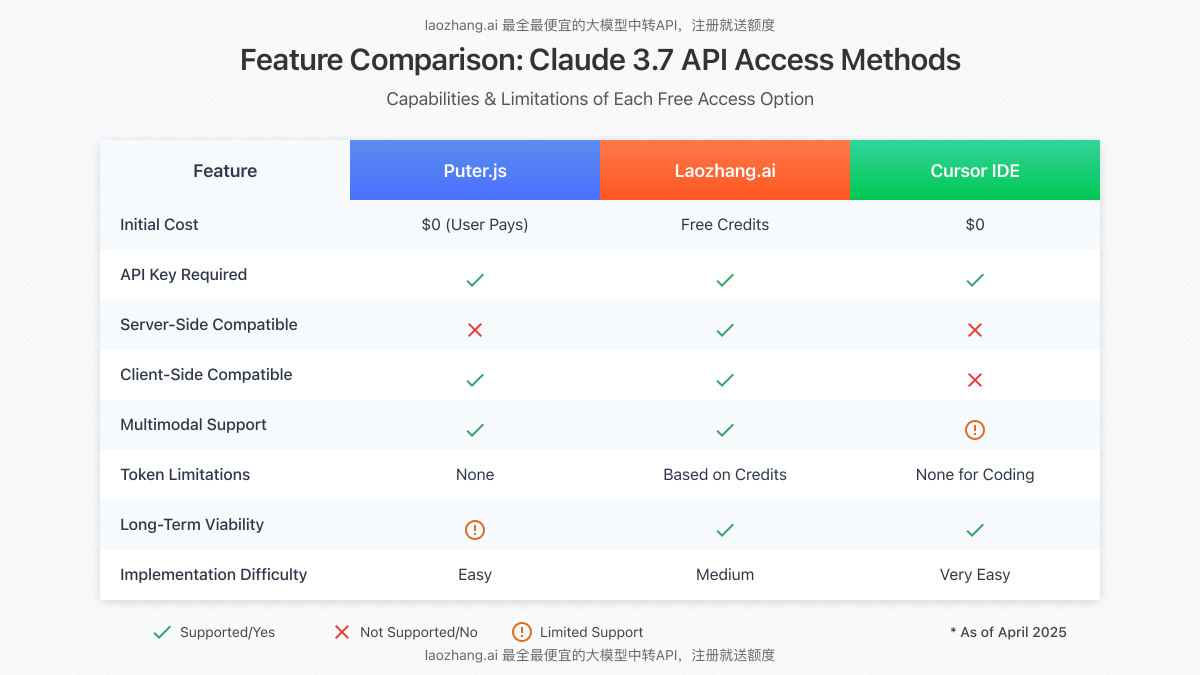
Cost Analysis and Performance Comparison
To help you select the best approach for your needs, here’s a comprehensive comparison of all methods:
Feature | Puter.js | Laozhang.ai | Cursor | Direct API |
---|---|---|---|---|
Initial Cost | $0 | $0 (with free credits) | $0 | Credit card required |
Server-side Access | No | Yes | No | Yes |
Client-side Access | Yes | Yes | Limited | No (security risk) |
Token Limits | None | Based on credits | None for coding | Pay per token |
API Format | Custom | OpenAI-compatible | N/A | Anthropic API |
Response Latency | Low | Medium | Very low | Very low |
Setup Complexity | Minimal | Low | Minimal | High |
Multimodal Support | Yes | Yes | Limited | Yes |
Monthly Cost Projection Example
For a mid-size application with approximately 5 million tokens processed monthly:
Solution | Monthly Cost | Annual Savings vs. Direct API |
---|---|---|
Direct Anthropic API | $450 | $0 (baseline) |
Puter.js | $0 (developer cost) | $5,400 |
Laozhang.ai | $225 | $2,700 |
Cursor (for dev only) | $0 | $5,400 |
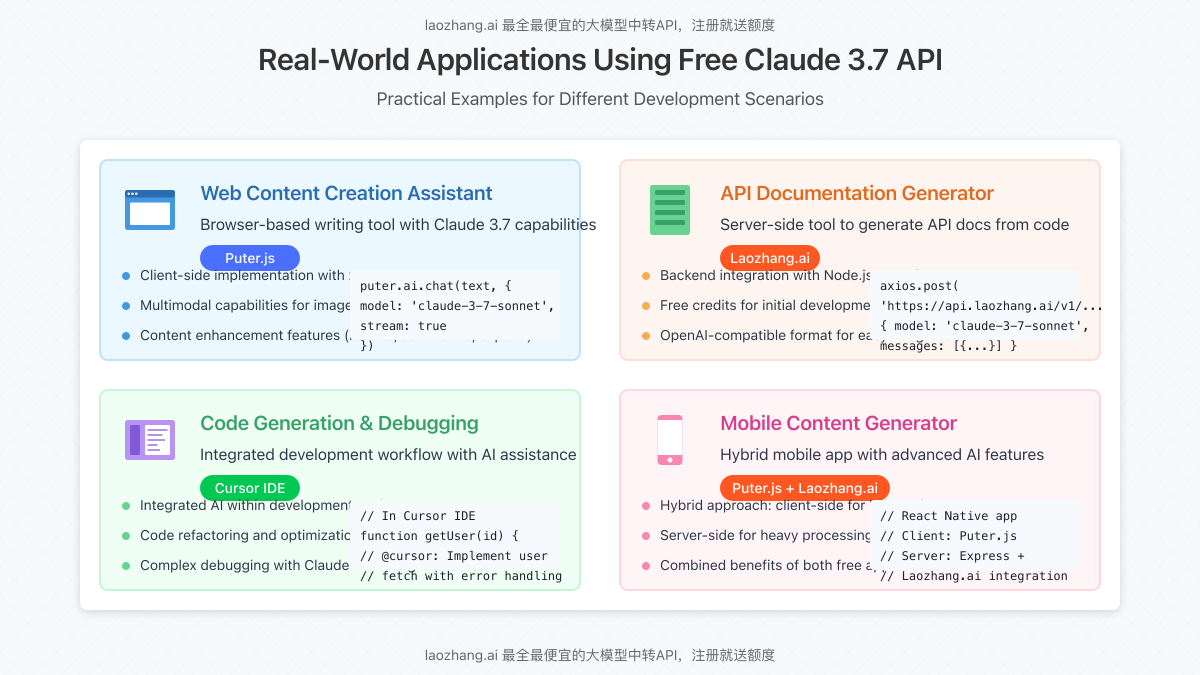
Practical Applications and Implementation Examples
Here are some real-world examples of how developers are using these free methods to access Claude 3.7 capabilities:
1. AI-Powered Content Creation Tool
Using Puter.js to create a browser-based writing assistant that leverages Claude 3.7’s reasoning capabilities:
// Content enhancement function using Claude 3.7
async function enhanceContent(originalText, enhancementType) {
const promptMap = {
'improve': 'Improve this text while keeping the same meaning: ',
'simplify': 'Rewrite this text to be simpler and more accessible: ',
'expand': 'Expand on this text with more details and examples: '
};
const prompt = promptMap[enhancementType] || promptMap['improve'];
try {
const response = await puter.ai.chat(
prompt + originalText,
{model: 'claude-3-7-sonnet'}
);
return response.message.content[0].text;
} catch (error) {
console.error('Error enhancing content:', error);
return originalText;
}
}
2. API-Based Code Documentation Generator
Using Laozhang.ai’s API to build a server-side documentation generator:
// Node.js documentation generator
const express = require('express');
const axios = require('axios');
const app = express();
app.use(express.json());
app.post('/generate-documentation', async (req, res) => {
const { code, language } = req.body;
if (!code || !language) {
return res.status(400).json({ error: 'Code and language are required' });
}
try {
const response = await axios.post(
'https://api.laozhang.ai/v1/chat/completions',
{
model: 'claude-3-7-sonnet',
messages: [
{
role: 'system',
content: 'You are a technical documentation specialist.'
},
{
role: 'user',
content: `Generate comprehensive documentation for the following ${language} code:\n\n${code}`
}
]
},
{
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${process.env.LAOZHANG_API_KEY}`
}
}
);
return res.json({
documentation: response.data.choices[0].message.content
});
} catch (error) {
console.error('Documentation generation error:', error);
return res.status(500).json({
error: 'Failed to generate documentation'
});
}
});
app.listen(3000, () => {
console.log('Documentation service running on port 3000');
});
3. Integrated Development Workflow
Using Cursor for an enhanced development workflow with Claude 3.7:
// Example workflow with Cursor commands
// 1. System design
// @cursor: Design a microservice architecture for a food delivery application
// 2. API specification
// @cursor: Create an OpenAPI specification for the restaurant search service
// 3. Implementation
// @cursor: Implement the search API endpoint using Express.js and MongoDB
// 4. Testing
// @cursor: Write Jest tests for the search functionality
// 5. Documentation
// @cursor: Generate API documentation for this endpoint
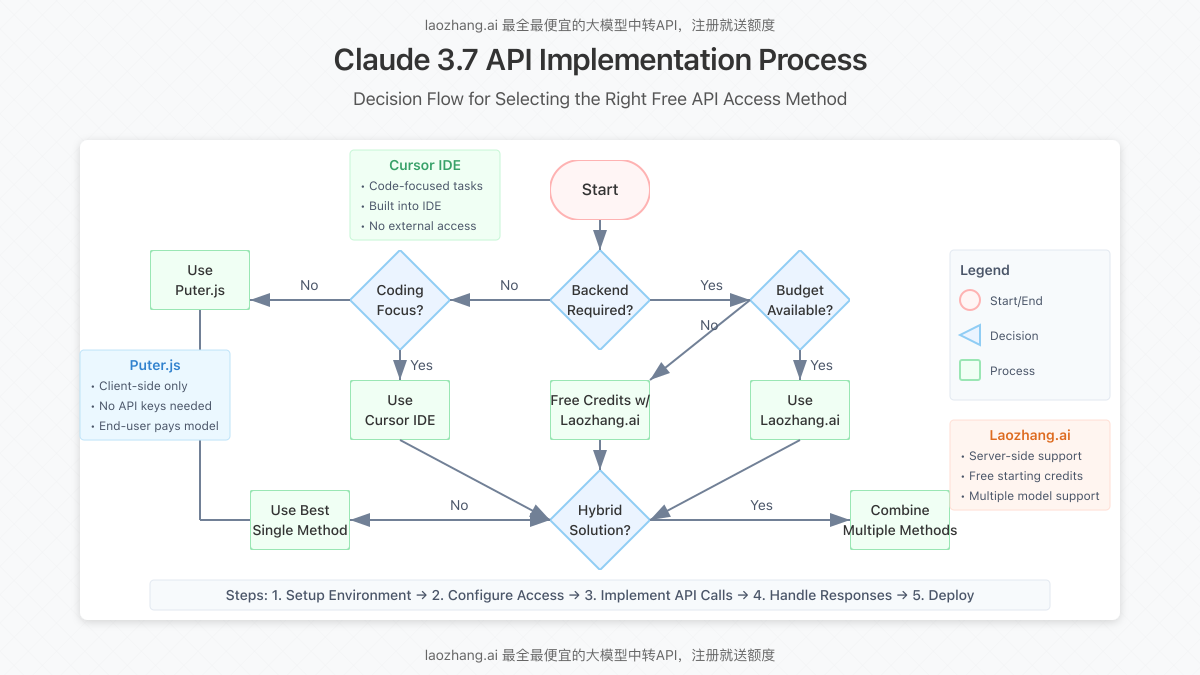
Best Practices and Optimization Tips
To maximize your free Claude 3.7 access, consider these optimization strategies:
1. Prompt Engineering
- Use Clear Instructions – Be specific about format, length, and style
- Leverage System Messages – Define the assistant’s role and constraints
- Provide Examples – Use few-shot learning for consistent outputs
- Enable Thinking Mode – For complex reasoning tasks, ask Claude to show its thinking process
2. Token Optimization
- Minimize Context – Only include necessary information
- Use Efficient Formats – Structured data over verbose text
- Implement Caching – Store common responses to avoid duplicate queries
- Batch Processing – Combine related tasks where possible
3. Error Handling
// Robust error handling for API calls
async function robustClaudeCall(prompt, retries = 3, delay = 1000) {
let lastError;
for (let attempt = 0; attempt < retries; attempt++) {
try {
const response = await puter.ai.chat(prompt, {
model: 'claude-3-7-sonnet'
});
return response.message.content[0].text;
} catch (error) {
console.warn(`Attempt ${attempt + 1} failed:`, error.message);
lastError = error;
// Rate limiting or server error - wait before retry
if (error.status === 429 || error.status >= 500) {
await new Promise(resolve => setTimeout(resolve, delay * Math.pow(2, attempt)));
continue;
}
// Client error - no point retrying
if (error.status >= 400 && error.status < 500) {
break;
}
}
}
throw new Error(`All ${retries} attempts failed. Last error: ${lastError.message}`);
}
4. Security Considerations
- Environment Variables – Always store API keys securely
- Input Sanitization – Validate and sanitize all user inputs
- Output Filtering – Implement content filtering for AI responses
- Rate Limiting – Prevent abuse with appropriate rate limits
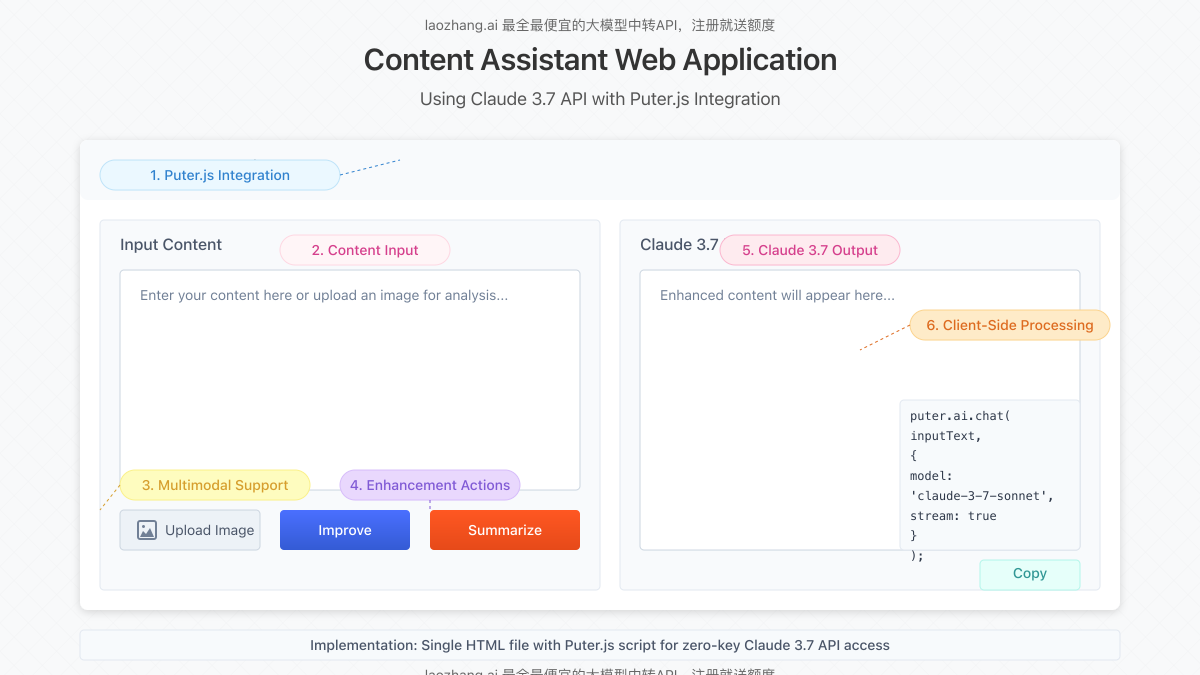
Limitations and Considerations
While these free methods provide valuable access to Claude 3.7, be aware of these important limitations:
Terms of Service Compliance
Always review the terms of service for each platform to ensure your usage complies with their policies. Some methods may have specific restrictions on commercial use or high-volume applications.
Service Reliability
Free services may experience:
- More frequent downtime or maintenance periods
- Potential for deprecation or significant changes
- Usage throttling during peak demand
- Lower priority in service queues
Feature Limitations
Free access methods typically restrict certain capabilities:
- Fine-tuning options may be limited or unavailable
- Advanced features might be reserved for paid tiers
- Maximum context window may be reduced
- Some specialized model variants might be inaccessible
Long-term Viability
Consider these factors for production applications:
- Free services may change pricing or access models
- Proxy services depend on their relationship with Anthropic
- Direct API access provides more stability but at higher cost
- Hybrid approaches may provide the best balance of cost and reliability
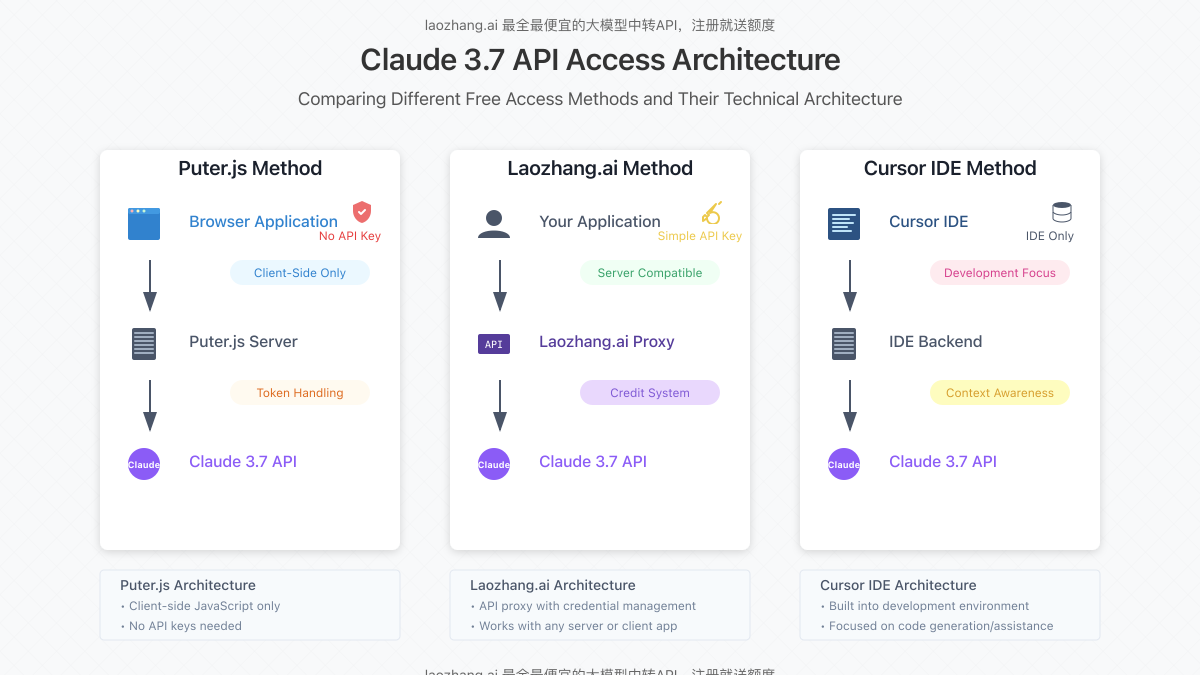
Conclusion: Finding Your Ideal Claude 3.7 Integration Path
After examining three legitimate methods for accessing Claude 3.7 API without payment, you can choose the approach that best suits your specific development requirements:
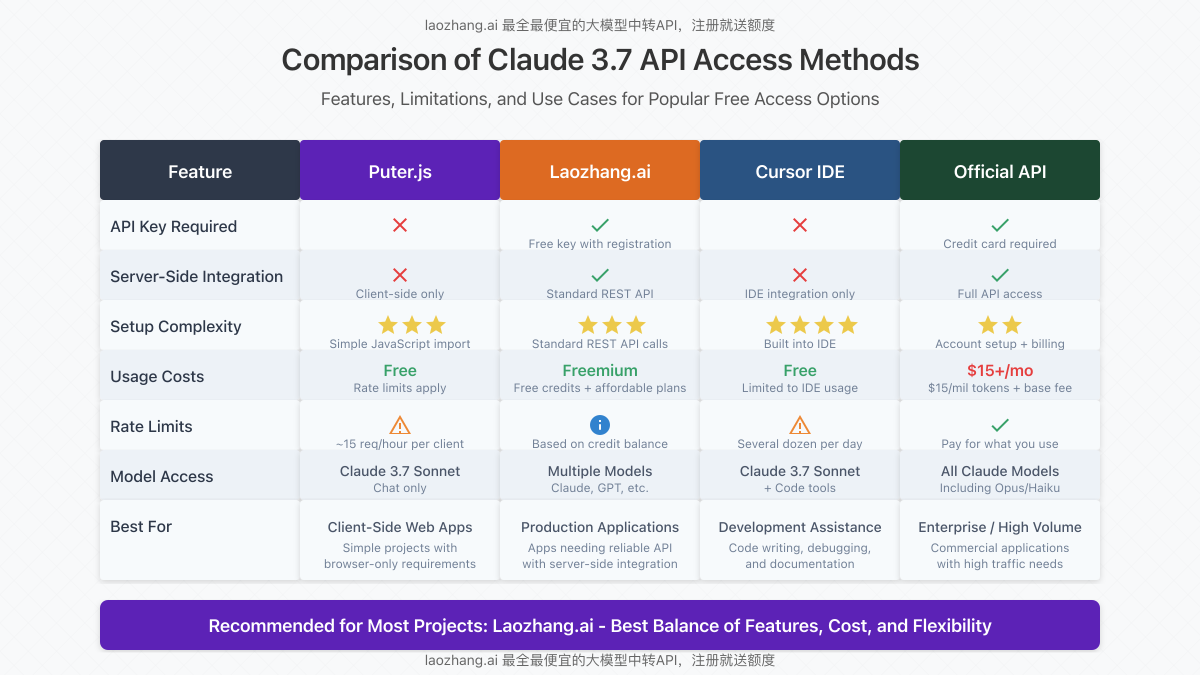
- For Client-Side Applications: Puter.js offers the simplest implementation with no usage limits, ideal for web applications where users can cover minimal computation costs
- For Server Applications: Laozhang.ai provides the most flexible integration with both free credits and reasonable rates for continued usage
- For Development Environments: Cursor IDE delivers Claude 3.7 capabilities directly within your coding environment at zero cost
Get Started Today
Try implementing one of these free Claude 3.7 access methods in your next project. For the most comprehensive solution with both free credits and ongoing cost savings, register for Laozhang.ai and start exploring the full potential of Claude 3.7 Sonnet.
Additional Resources
- Puter.js Documentation
- Laozhang.ai API Reference
- Cursor AI Features Guide
- Official Claude API Documentation
By following the implementation steps and best practices outlined in this guide, you can unlock the power of Claude 3.7 API for your projects without spending a penny. Each method offers unique advantages, and you can even combine approaches for maximum flexibility.
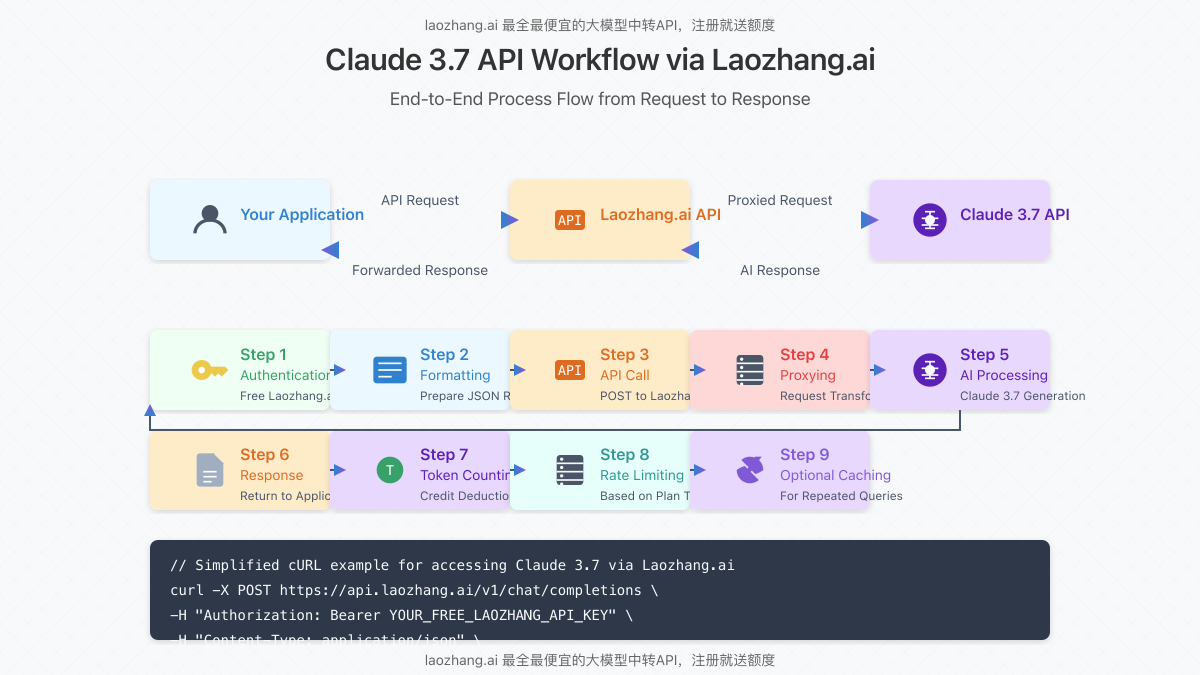
Get Started Today
Try implementing one of these free Claude 3.7 access methods in your next project. For the most comprehensive solution with both free credits and ongoing cost savings, register for Laozhang.ai and start exploring the full potential of Claude 3.7 Sonnet.