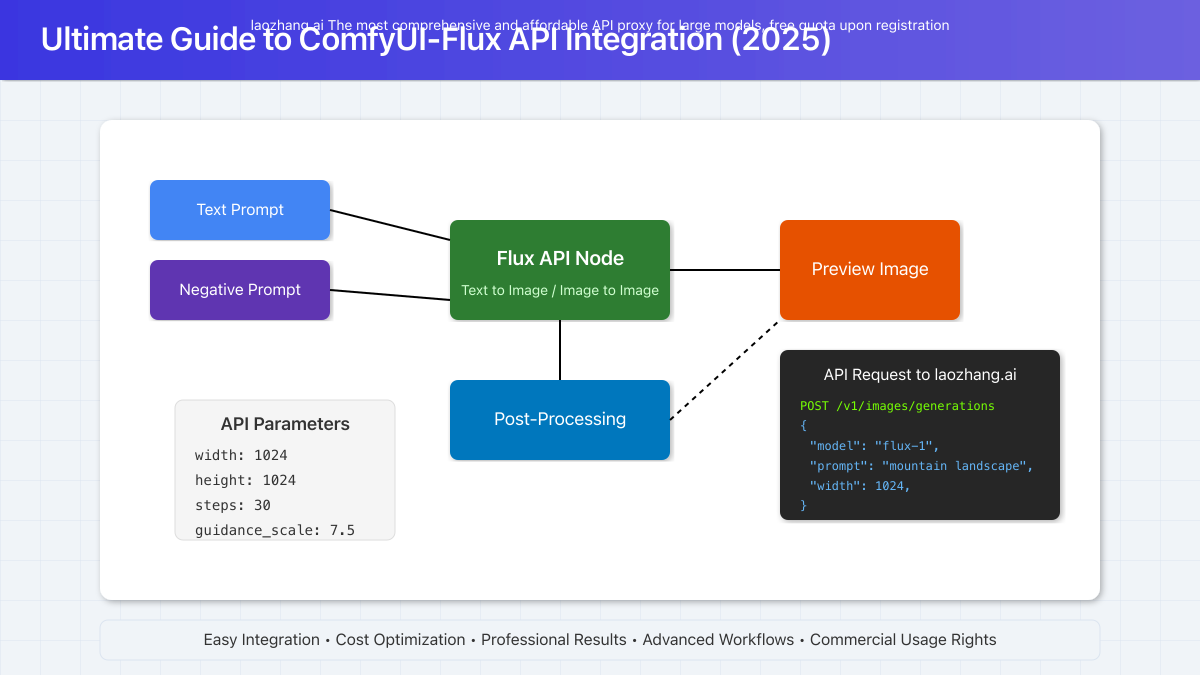
The integration of Flux API with ComfyUI represents a significant advancement for AI image generation workflows in 2025. Whether you’re a developer, content creator, or AI enthusiast, this comprehensive guide will walk you through everything you need to know about implementing Flux API in ComfyUI, from basic setup to advanced optimization techniques that deliver superior results.
What is Flux API and Why Use it with ComfyUI?
Flux is a state-of-the-art text-to-image and image-to-image model developed by Black Forest Labs (founded by former core Stability AI team members). When integrated with ComfyUI, it offers exceptional image generation capabilities with three primary versions:
- Flux Pro: Available only via API, offers commercial usage rights
- Flux Ultra: Premium version with enhanced capabilities
- Flux Dev: Local deployment version, non-commercial use only
ComfyUI, as a powerful node-based UI for Stable Diffusion, provides the flexibility and control needed to leverage Flux’s capabilities fully. The combination unlocks advanced workflows for professional image generation with precise control over the output.
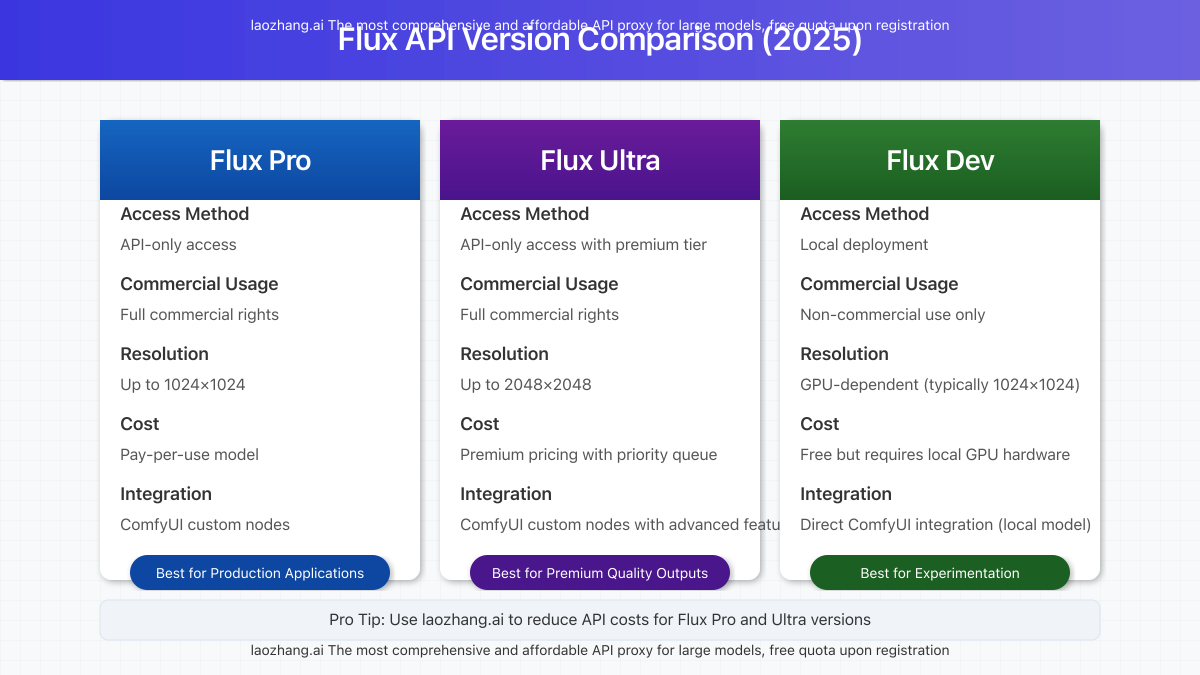
Setting Up ComfyUI for Flux API Integration
Prerequisites
Before integrating Flux API with ComfyUI, ensure you have:
- ComfyUI installed and functioning correctly
- Basic understanding of ComfyUI workflows
- An API key from a provider supporting Flux
- Required custom nodes installed
Important: While there are multiple ways to access Flux API, using a cost-effective API proxy service like laozhang.ai can significantly reduce expenses while providing full access to Flux capabilities.
Step 1: Install Required Custom Nodes
To integrate Flux API with ComfyUI, you’ll need to install specific custom nodes. Here are two popular options:
- ComfyUI-Cloud-APIs: A versatile extension supporting multiple cloud APIs including Flux
- ComfyUI-fal-API: Custom nodes specifically designed for fal.ai services including Flux
Install these custom nodes using ComfyUI Manager or manual installation:
cd ComfyUI/custom_nodes
git clone https://github.com/yhayano-ponotech/ComfyUI-Fal-API-Flux
cd ComfyUI-Fal-API-Flux
pip install -r requirements.txt
Step 2: Configure Your API Key
After installation, you’ll need to configure your API key:
- Create a text file named
keys.txt
in the extension folder - Add your API key in the format:
FAL_KEY=your_api_key_here
- Save the file and restart ComfyUI
Pro Tip: With laozhang.ai proxy service, you can access Flux API at more affordable rates. Register at api.laozhang.ai to receive free quota upon registration.
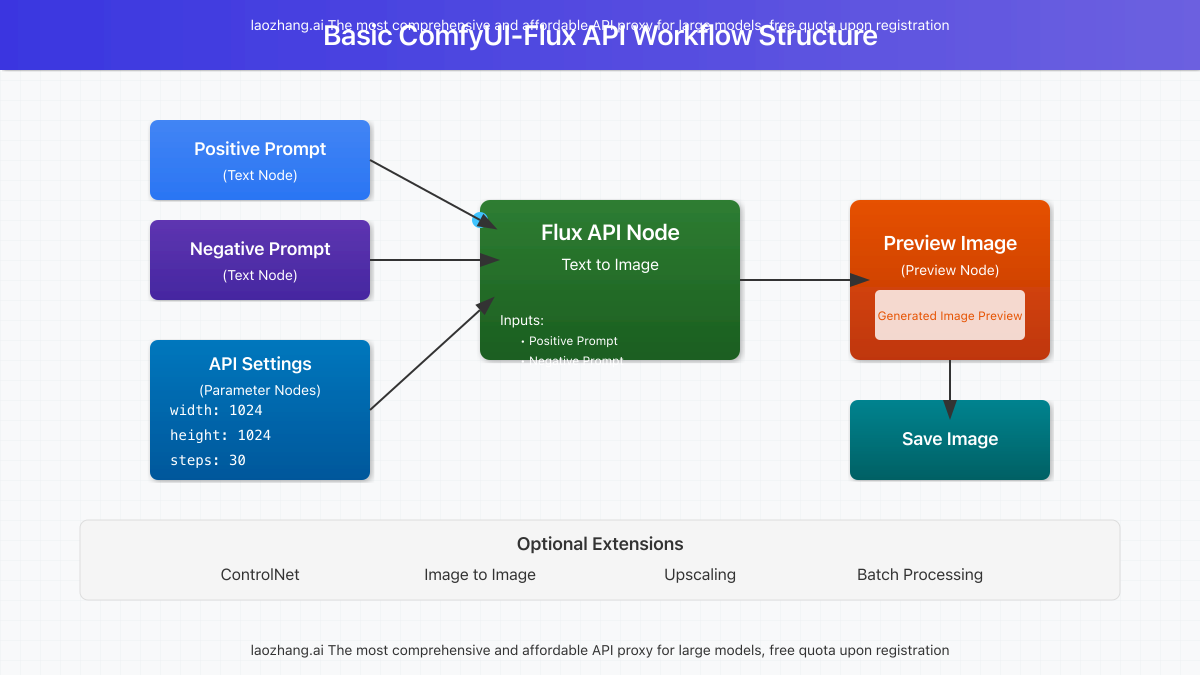
Creating Your First Flux API Workflow in ComfyUI
Now that you’ve set up the necessary components, let’s create a basic workflow for using Flux API in ComfyUI:
Text-to-Image Workflow
- Add a Text (Prompt) node for your positive prompt
- Add another Text (Prompt) node for your negative prompt
- Add the Flux API Text to Image node from your installed custom nodes
- Connect the positive prompt to the first input of the Flux API node
- Connect the negative prompt to the second input of the Flux API node
- Add a Preview Image node and connect it to the output of the Flux API node
With this basic workflow, you can generate images using Flux through the API. The node parameters allow you to control:
- Width and height of the output image
- Number of inference steps
- CFG scale (how closely the image follows your prompt)
- Seed value for reproducible results
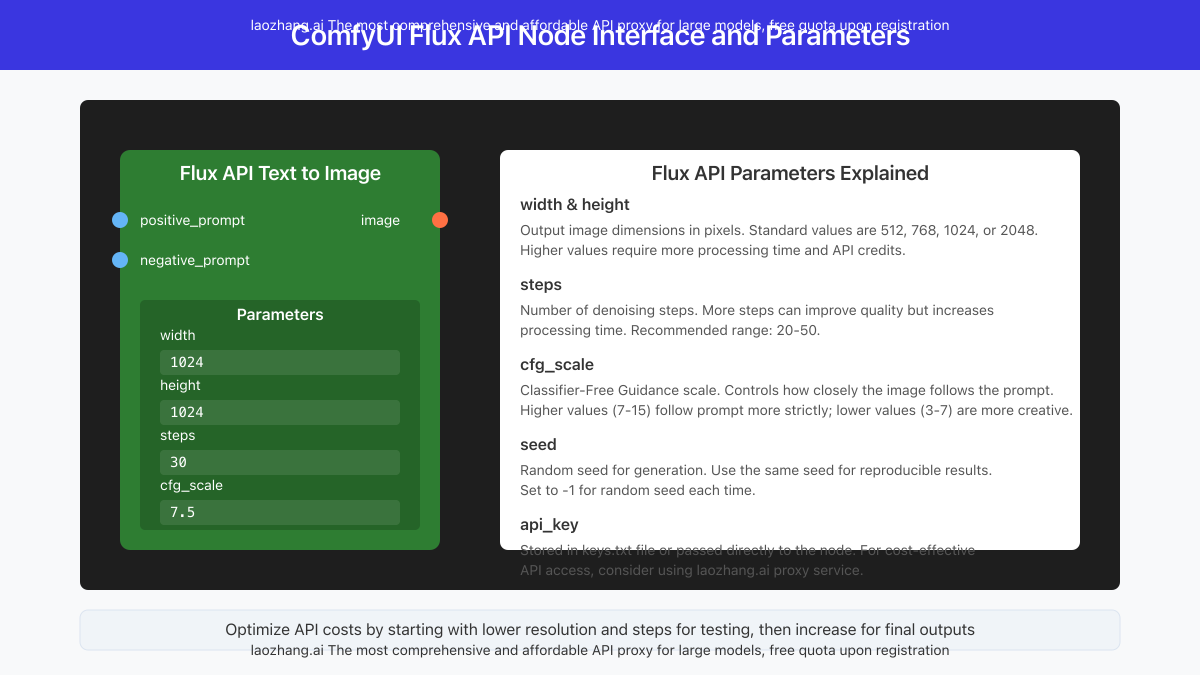
Image-to-Image Workflow
For image-to-image generation with Flux API:
- Create the basic text-to-image workflow as above
- Add a Load Image node
- Replace the Flux API Text to Image node with Flux API Image to Image
- Connect the image node to the additional input of the Flux API node
- Adjust the denoising strength parameter to control how much of the original image is preserved
Advanced Flux API Techniques in ComfyUI
Integrating ControlNet with Flux API
Combining ControlNet with Flux API allows for precise control over the generated images:
- Add a Load Image node for your control image
- Add appropriate preprocessor nodes (Canny, Depth, etc.) depending on your control method
- Connect the preprocessor output to the Flux API with ControlNet node
- Connect your prompt nodes as in the basic workflow
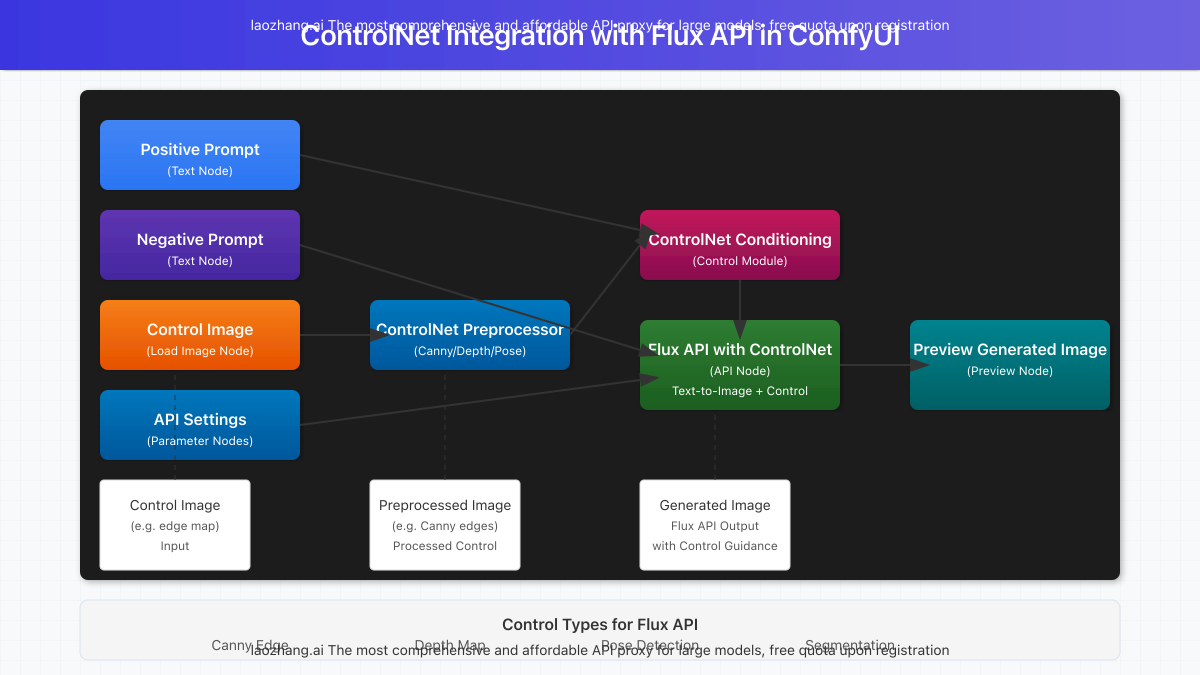
Upscaling and Post-Processing
Enhance your Flux-generated images with these post-processing techniques:
- Connect the Flux API output to a High-Resolution Upscaler node
- Implement face restoration with GFPGAN or CodeFormer nodes
- Apply Detail Enhancer nodes for sharper details
These post-processing steps can significantly improve the quality of your generated images while maintaining the distinctive Flux style.
Batch Processing with Flux API
For efficient batch processing:
- Add an Integer node set to your desired batch count
- Connect it to the Flux API Batch node
- Use Primitive Nodes to create loops for processing multiple prompts
Warning: Batch processing with API calls can quickly consume your API quota. Monitor usage carefully or use cost-effective providers like laozhang.ai to optimize expenses.
Creating a Scalable API Endpoint with ComfyUI and Flux
For developers looking to create a scalable service, you can convert your ComfyUI workflow into an API endpoint:
Using Modal for API Deployment
Modal.com offers a straightforward way to turn your ComfyUI workflow into an API:
import modal
stub = modal.Stub("comfyui-flux-api")
@stub.function(gpu="A10G")
def run_comfyui_workflow(prompt, negative_prompt, width=1024, height=1024):
# Import necessary libraries
import requests
import json
# Configure API request with Flux settings
payload = {
"prompt": prompt,
"negative_prompt": negative_prompt,
"width": width,
"height": height,
"model": "flux"
}
# Process workflow and return results
# ... (implementation details)
return image_result
@stub.local_entrypoint()
def main():
result = run_comfyui_workflow(
"photorealistic landscape, mountains, lake, sunset",
"blur, distortion, low quality"
)
result.save("output.png")
Direct API Integration with Python
Alternatively, you can use Python to directly integrate with the Flux API via laozhang.ai:
import requests
import json
import base64
from PIL import Image
import io
# API endpoint and authentication
API_URL = "https://api.laozhang.ai/v1/images/generations"
API_KEY = "your_api_key_here"
# Request payload
payload = {
"model": "flux-1",
"prompt": "photorealistic landscape, mountains, lake, sunset",
"negative_prompt": "blur, distortion, low quality",
"width": 1024,
"height": 1024,
"steps": 30,
"guidance_scale": 7.5
}
# Make API request
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {API_KEY}"
}
response = requests.post(API_URL, headers=headers, json=payload)
# Process and display the image
if response.status_code == 200:
data = response.json()
image_data = base64.b64decode(data['data'][0]['b64_json'])
image = Image.open(io.BytesIO(image_data))
image.save("flux_output.png")
print("Image generated successfully!")
else:
print(f"Error: {response.status_code}")
print(response.text)
Troubleshooting Common Flux API Issues in ComfyUI
When working with Flux API in ComfyUI, you might encounter these common issues:
Authentication Errors
- Issue: “Invalid API key” or authentication errors
- Solution: Verify your API key is correctly formatted in the keys file without any extra spaces or quotes
Timeout Errors
- Issue: API requests timing out
- Solution: Increase timeout settings in the node parameters or check network connectivity
Quota Exceeded
- Issue: “Quota exceeded” error messages
- Solution: Use a service like laozhang.ai for more affordable access or optimize your workflow to use fewer API calls
Node Connection Errors
- Issue: Nodes not connecting properly
- Solution: Verify node version compatibility and ensure all required dependencies are installed
Cost Optimization for Flux API Usage
API costs can add up quickly when using Flux API. Here are strategies to optimize your expenses:
Use API Proxy Services
laozhang.ai offers a cost-effective API proxy service for accessing Flux and other AI models:
- Significantly lower per-request costs compared to direct API access
- Free quota upon registration
- Simple integration with existing workflows
Example API request using laozhang.ai:
curl https://api.laozhang.ai/v1/images/generations \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "flux-1",
"prompt": "photorealistic mountain landscape",
"negative_prompt": "blur, distortion",
"width": 1024,
"height": 1024
}'
Batch Processing Optimization
Reduce costs by optimizing your batch processing approach:
- Group similar requests to minimize API calls
- Implement client-side caching for frequently used prompts
- Use lower resolution for initial tests, then higher resolution for final outputs
Pro Tip: Register at api.laozhang.ai to get started with free quota and significantly reduce your API costs for Flux and other AI models.
Comparing Flux with Other Text-to-Image Models in ComfyUI
Understanding how Flux compares to other models helps you choose the right tool for specific projects:
Feature | Flux | SDXL | Midjourney |
---|---|---|---|
Photorealism | Exceptional | Very Good | Excellent |
Prompt Adherence | High | Medium | Medium-High |
Integration Ease | Medium (API) | High (Local) | Low (Discord only) |
Cost | Medium-High | Low (Self-hosted) | Subscription |
Commercial Usage | Yes (Pro/Ultra) | Model-dependent | Yes (with subscription) |
Flux excels in photorealistic image generation with exceptional prompt adherence, making it ideal for professional projects requiring high-quality outputs.
FAQ: ComfyUI Flux API Integration
Can I use Flux API results commercially?
Yes, images generated with Flux Pro and Ultra versions can be used commercially. However, Flux Dev version is for non-commercial use only.
How can I reduce Flux API costs?
Use an API proxy service like laozhang.ai, implement efficient batching, and optimize your workflow to minimize API calls.
Is Flux better than SDXL for all use cases?
Not necessarily. While Flux excels at photorealism, SDXL may be better for stylized imagery or when working with limited resources.
Can I run Flux locally without API calls?
You can run Flux Dev locally for non-commercial use, but Flux Pro and Ultra are only available via API.
Do I need a powerful GPU to use Flux API?
No, since processing happens on the server side, you don’t need a powerful GPU for Flux API integration.
How do I troubleshoot “API rate limit exceeded” errors?
Either upgrade your API plan, implement request throttling, or use a proxy service like laozhang.ai with more generous rate limits.
Conclusion: Maximizing Your ComfyUI-Flux API Implementation
Integrating Flux API with ComfyUI offers powerful capabilities for professional AI image generation. By following the steps outlined in this guide, you can create sophisticated workflows that leverage Flux’s exceptional quality while optimizing costs through services like laozhang.ai.
Whether you’re developing applications, creating content, or exploring the boundaries of AI-generated imagery, the ComfyUI-Flux API combination provides the tools you need to achieve professional results efficiently.
Register at api.laozhang.ai today to get started with your free quota and experience the benefits of cost-effective Flux API access for your ComfyUI workflows.