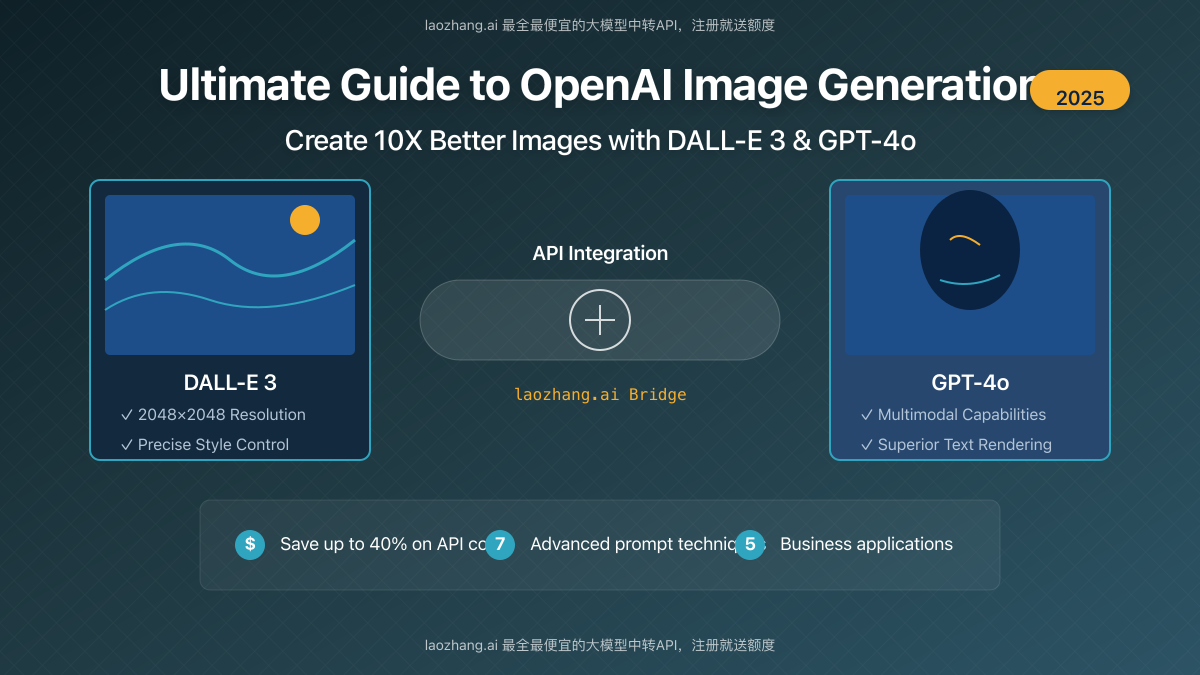
OpenAI has revolutionized AI image generation with its powerful DALL-E models and the recent introduction of GPT-4o’s native multimodal image capabilities. In 2025, these technologies have matured dramatically, enabling unprecedented photorealistic outputs, precise control, and remarkable creative possibilities for businesses, developers, and content creators.
This comprehensive guide cuts through the complexity to deliver everything you need to know about leveraging OpenAI’s image generation capabilities effectively – whether you’re a developer integrating the API, a business owner creating marketing assets, or a creative professional expanding your toolkit.
What you’ll learn:
- How to access and use OpenAI’s latest image generation models (DALL-E 3 and GPT-4o)
- Step-by-step API implementation with code examples
- 7 advanced prompt engineering techniques for superior image results
- Cost optimization strategies (save up to 40% on API calls)
- 5 practical business applications with real-world case studies
What’s New in OpenAI Image Generation for 2025
OpenAI has made significant advancements in its image generation capabilities this year, with two major developments transforming the landscape:
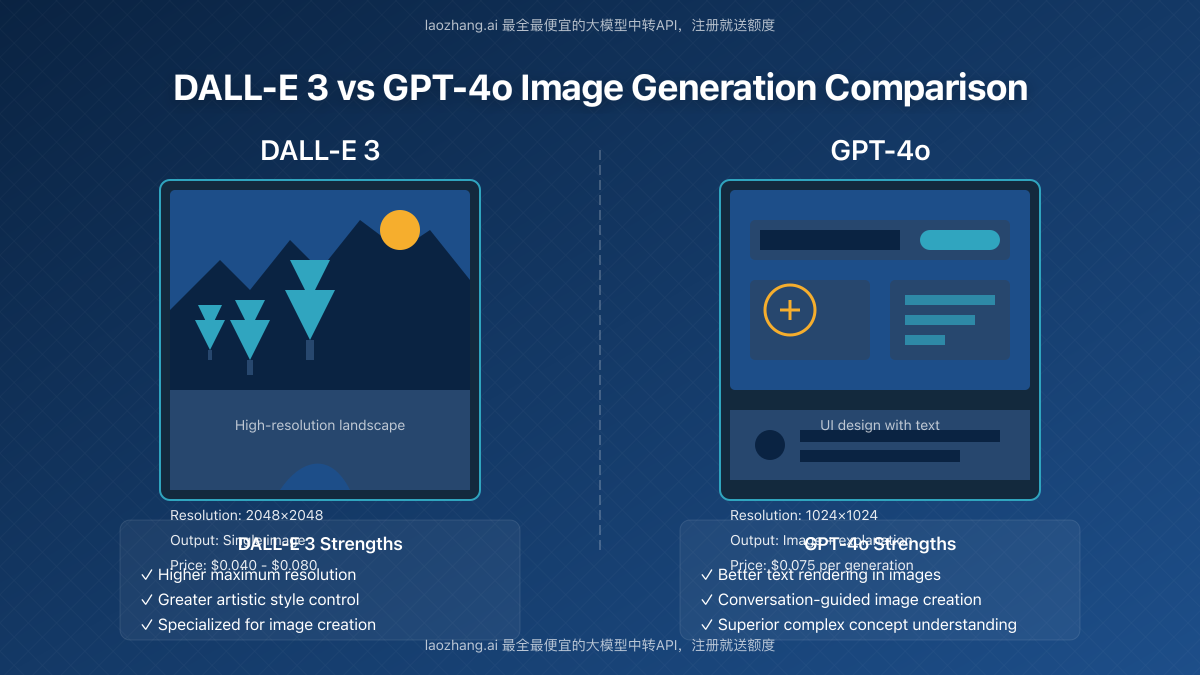
1. GPT-4o’s Integrated Image Generation
Released in March 2025, GPT-4o represents a fundamental shift in AI image generation. Unlike previous models that required separate systems for text and image generation, GPT-4o is natively multimodal, allowing for:
- Seamless text-to-image generation within the same model
- Superior understanding of complex visual concepts and nuanced prompts
- Enhanced photorealism with remarkable attention to detail
- Improved accuracy for text rendering within images
- Better adherence to specific style requests
2. DALL-E 3 API Improvements
While GPT-4o has captured headlines, DALL-E 3 remains OpenAI’s dedicated image generation model, with several key improvements in 2025:
- Higher resolution outputs (now up to 2048×2048 pixels)
- More consistent artistic style application
- Better handling of complex scenes with multiple subjects
- Reduced hallucinations and improved anatomical accuracy
- New endpoint options for specialized image types
Feature | DALL-E 3 | GPT-4o |
---|---|---|
Primary purpose | Dedicated image generation | Multimodal AI (text, vision, and image generation) |
Max resolution | 2048×2048 pixels | 1024×1024 pixels |
Style control | Very high (specialized) | High (integrated with text understanding) |
Text accuracy in images | Good | Excellent |
API pricing (1024×1024) | $0.040 per image | $0.075 per image generation |
Availability | API, ChatGPT Plus | API, ChatGPT Plus |
How to Access OpenAI Image Generation
There are three primary ways to access OpenAI’s image generation capabilities, each with different use cases and requirements:
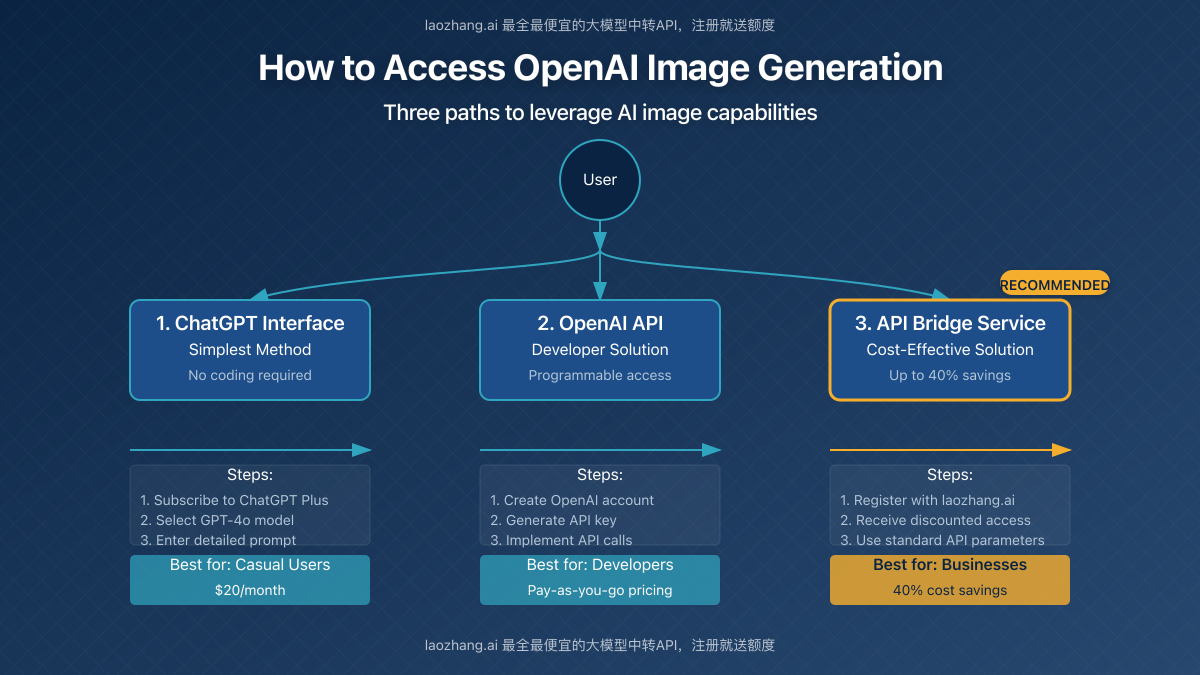
1. ChatGPT Interface (Simplest Method)
For casual users or those testing concepts, the ChatGPT web interface provides the most straightforward access:
- Subscribe to ChatGPT Plus ($20/month) or ChatGPT Team ($25/user/month)
- Access GPT-4o with image generation capabilities
- Enter a detailed prompt describing your desired image
- Use the “Generate image” option or simply ask it to create an image
Limitations: While convenient, this method lacks API integration, has usage caps, and doesn’t support automated workflows or high-volume needs.
2. OpenAI API (Developer Solution)
For developers and businesses requiring programmatic access, custom applications, or higher volumes:
- Create an OpenAI developer account at platform.openai.com
- Add a payment method (credit card required)
- Generate an API key from your dashboard
- Implement API calls to the image generation endpoints
Considerations: While powerful, direct API access requires development expertise, comes with higher costs for scaling applications, and may have rate limits for new accounts.
3. API Bridge Service (Cost-Effective Solution)
For businesses seeking cost optimization, simplified integration, and reliable access:
- Register with an API bridge provider like laozhang.ai
- Receive discounted access to OpenAI’s API (up to 40% savings)
- Use standard OpenAI API parameters with the bridge endpoint
- Benefit from additional features like usage analytics and proxy handling
Benefits: This approach significantly reduces costs, simplifies implementation with direct API compatibility, and often includes value-added services like enhanced reliability and technical support.
Implementing OpenAI Image Generation API
Basic API Implementation
Here’s how to implement OpenAI’s image generation API in your applications:
1. Authentication Setup
// Store your API key securely, preferably as an environment variable
const OPENAI_API_KEY = process.env.OPENAI_API_KEY;
// For laozhang.ai API bridge (cost-effective option)
const API_ENDPOINT = "https://api.laozhang.ai/v1/images/generations";
// For direct OpenAI access
// const API_ENDPOINT = "https://api.openai.com/v1/images/generations";
2. Basic Image Generation Request
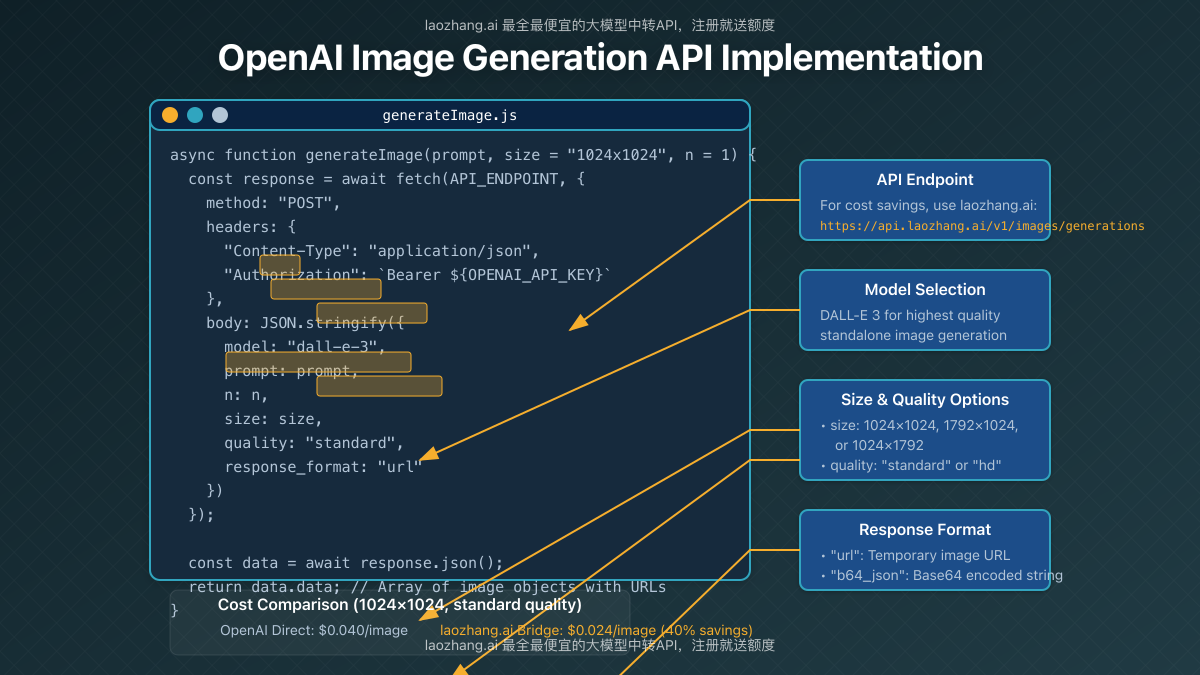
async function generateImage(prompt, size = "1024x1024", n = 1) {
const response = await fetch(API_ENDPOINT, {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${OPENAI_API_KEY}`
},
body: JSON.stringify({
model: "dall-e-3",
prompt: prompt,
n: n,
size: size,
quality: "standard",
response_format: "url"
})
});
const data = await response.json();
return data.data; // Array of image objects with URLs
}
// Example usage
generateImage("A photorealistic cityscape of Tokyo at night with neon lights reflected in rain puddles")
.then(images => console.log(images[0].url))
.catch(error => console.error("Generation failed:", error));
3. Python Implementation
import os
import requests
# Use environment variables for API keys
api_key = os.environ.get("OPENAI_API_KEY")
# For laozhang.ai API bridge (40% cost savings)
api_endpoint = "https://api.laozhang.ai/v1/images/generations"
# For direct OpenAI access
# api_endpoint = "https://api.openai.com/v1/images/generations"
def generate_image(prompt, size="1024x1024", n=1):
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}"
}
payload = {
"model": "dall-e-3",
"prompt": prompt,
"n": n,
"size": size,
"quality": "standard",
"response_format": "url"
}
response = requests.post(api_endpoint, headers=headers, json=payload)
return response.json()["data"]
# Example usage
result = generate_image("A detailed architectural rendering of a futuristic sustainable city with vertical gardens")
print(result[0]["url"])
Advanced API Features
Beyond basic implementation, OpenAI’s image generation API offers several advanced features:
1. Image Variations
Create variations of existing images using the variations endpoint:
async function createImageVariation(imageFile, n = 1, size = "1024x1024") {
const formData = new FormData();
formData.append("image", imageFile);
formData.append("n", n);
formData.append("size", size);
const response = await fetch("https://api.laozhang.ai/v1/images/variations", {
method: "POST",
headers: {
"Authorization": `Bearer ${OPENAI_API_KEY}`
},
body: formData
});
const data = await response.json();
return data.data;
}
2. Image Quality & Size Options
DALL-E 3 offers multiple quality and size options to balance cost and detail:
Size Option | Dimensions | Standard Quality Price | HD Quality Price |
---|---|---|---|
1024×1024 | 1 megapixel | $0.040 | $0.080 |
1024×1792 | 1.8 megapixels | $0.080 | $0.120 |
1792×1024 | 1.8 megapixels | $0.080 | $0.120 |
Use “quality”: “hd” in your API requests to enable higher-detail generation with improved aesthetics for more complex prompts.
3. Response Formats
The API supports multiple return formats for the generated images:
- URL (default): Returns a temporary URL to the generated image
- b64_json: Returns the image as a base64-encoded JSON string
Important: URL responses from OpenAI expire after 1 hour. Always download and store generated images promptly if you need to retain them.
7 Advanced Prompt Engineering Techniques for Better Images
The quality of your image generation results depends heavily on your prompt construction. These seven techniques will significantly improve your outputs:
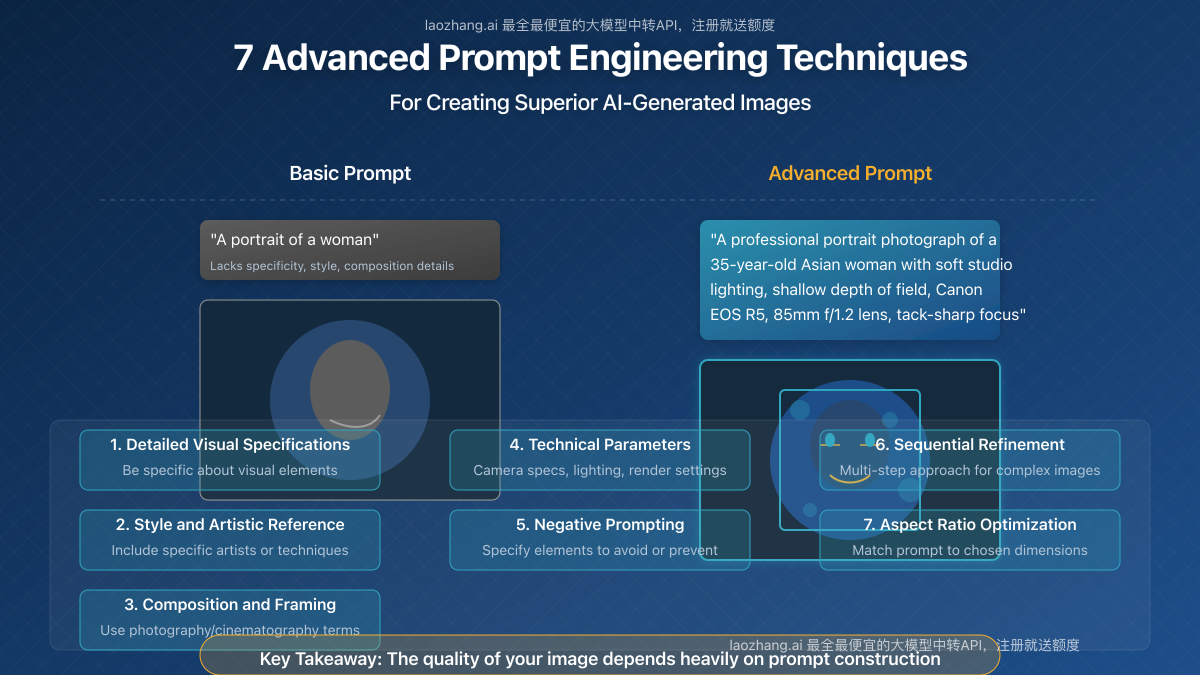
1. Detailed Visual Specifications
Be extremely specific about visual elements, providing clear details about:
Basic prompt: “A portrait of a woman”
Advanced prompt: “A professional portrait photograph of a 35-year-old Asian woman with shoulder-length black hair and subtle makeup, wearing a navy blue blazer against a neutral gray background, shot with soft studio lighting creating gentle shadows, shallow depth of field with tack-sharp focus on her eyes, photographed with a Canon EOS R5 and 85mm f/1.2 lens”
2. Style and Artistic Reference
Include specific artistic styles, techniques, or reference artists:
Basic prompt: “A mountain landscape”
Advanced prompt: “A breathtaking mountain landscape in the detailed style of Thomas Kinkade meets Albert Bierstadt, with dramatic lighting, atmospheric perspective showing layers of distant peaks, vibrant color palette emphasizing golden hour illumination, highly detailed foreground elements including pine trees and wildflowers, oil painting technique with visible brushstrokes and rich texture”
3. Composition and Framing
Direct the visual composition with photography and cinematography terms:
Basic prompt: “A city street at night”
Advanced prompt: “A cinematic wide-angle view of a rain-slicked Tokyo street at night, shot from a low angle with strong perspective lines leading to a vanishing point, featuring neon signs creating colorful reflections in puddles, atmospheric fog adding depth, rule-of-thirds composition with a balanced arrangement of visual elements, dramatic contrast between deep shadows and bright highlights typical of neo-noir cinematography”
4. Technical Parameters
Include technical photography terms to guide the rendering:
- Camera models (Canon EOS R5, Hasselblad H6D)
- Lens specifications (85mm f/1.2, 16-35mm ultra-wide)
- Lighting setups (three-point lighting, split lighting)
- Render settings (raytracing, 8K resolution)
5. Negative Prompting
While DALL-E 3 doesn’t explicitly support negative prompts, you can incorporate preventative language:
“A serene forest landscape with sunlight filtering through trees. The image should avoid any human figures, buildings, or artificial structures. Maintain botanical accuracy with no misshapen plants or anatomically incorrect animals.”
6. Sequential Refinement (API)
For best results with complex images, use a multi-step approach:
- Generate an initial image with core elements
- Request specific modifications in follow-up generations
- Use variations API to explore alternatives while preserving key elements
7. Aspect Ratio Optimization
Match your prompt to your chosen aspect ratio for better composition:
- Square (1024×1024): Balanced compositions, portraits, logos
- Portrait (1024×1792): Full-body portraits, book covers, mobile content
- Landscape (1792×1024): Landscapes, wide scenes, desktop wallpapers
Cost Optimization: Save Up to 40% on OpenAI Image Generation
API costs can add up quickly, especially for businesses generating multiple images. Here are effective strategies to reduce your expenditure:
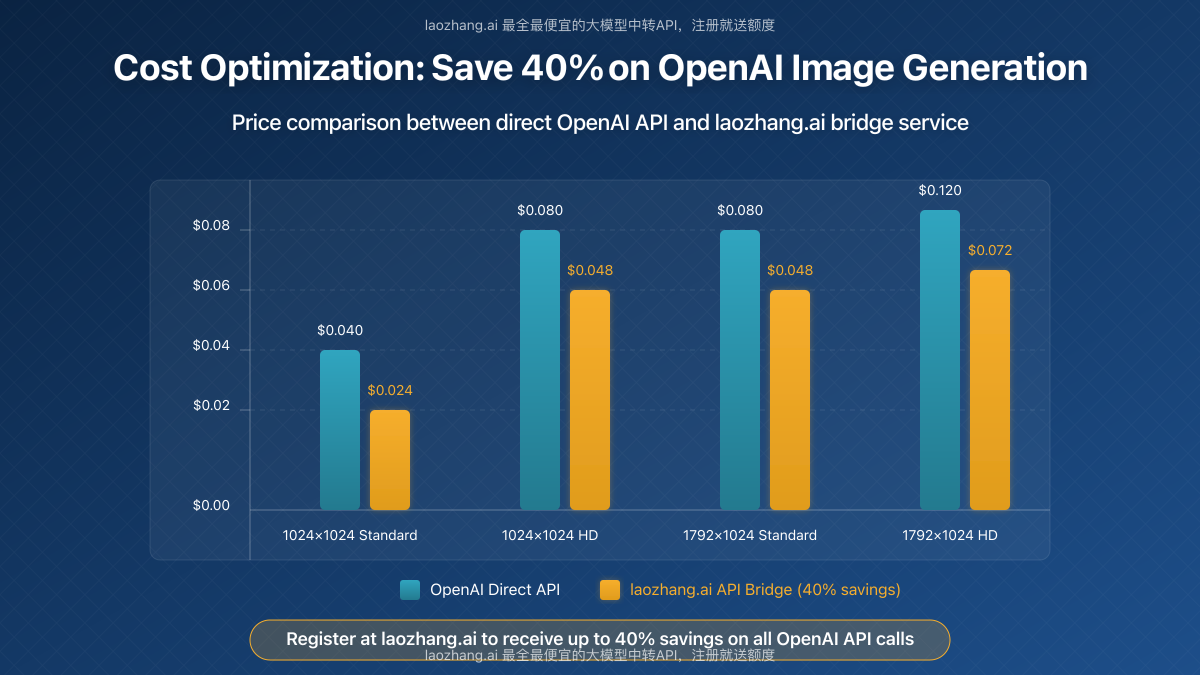
1. Use an API Bridge Service
The most substantial savings come from using an API bridge service like laozhang.ai, which offers:
- Up to 40% lower rates compared to direct OpenAI pricing
- Identical API interface requiring minimal code changes
- Free credits upon registration for testing
- Enterprise volume discounts for high-usage customers
// Replace this:
const API_ENDPOINT = "https://api.openai.com/v1/images/generations";
// With this:
const API_ENDPOINT = "https://api.laozhang.ai/v1/images/generations";
// The rest of your code remains unchanged
2. Optimize Quality Settings
Not every image requires the highest quality settings:
- Use “standard” quality for drafts and internal use
- Reserve “hd” quality for final versions and client deliverables
- Consider smaller dimensions for images that will be displayed at reduced sizes
3. Implement Caching Strategies
For applications with predictable or repeated image needs:
- Store generated images in cloud storage with appropriate metadata
- Implement a lookup system to avoid regenerating similar images
- Consider similarity-based image retrieval for finding close matches to new requests
4. Batch Processing for Volume Discounts
Some API bridge providers offer better rates for batch processing:
- Group image generation requests when possible
- Schedule non-urgent generation during off-peak hours if your provider offers time-based pricing
- Negotiate volume discounts for predictable monthly usage
5 Practical Business Applications with Case Studies
OpenAI’s image generation capabilities are transforming how businesses create visual content:
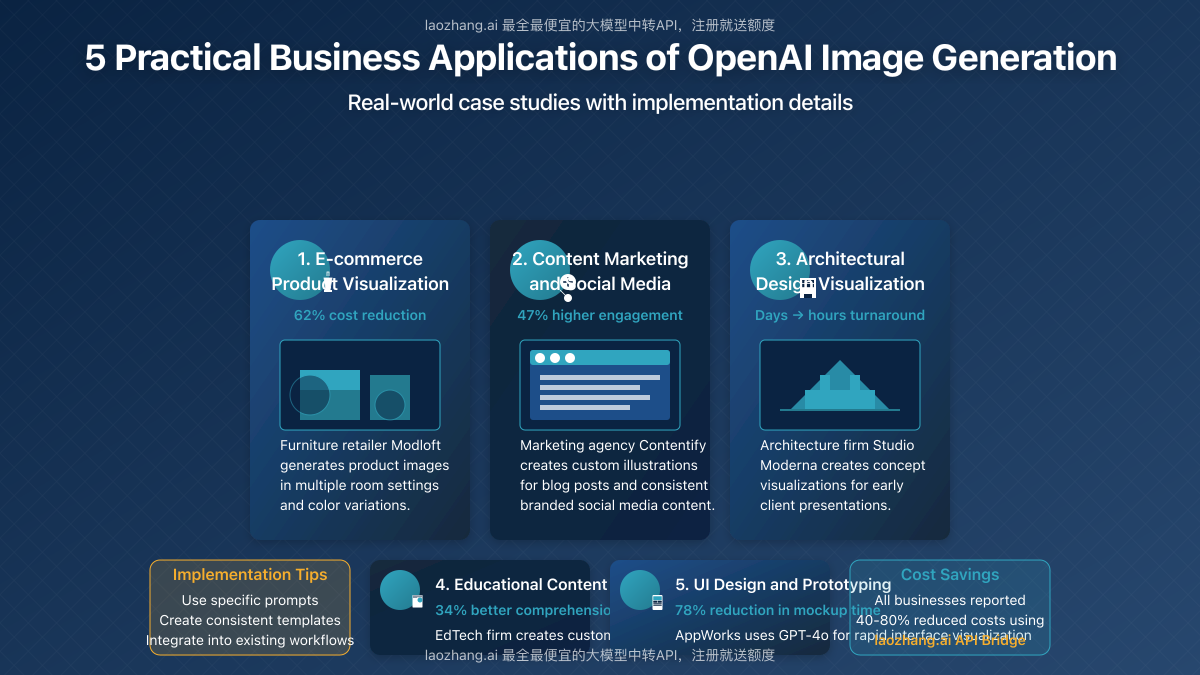
1. E-commerce Product Visualization
Case Study: Online furniture retailer Modloft reduced product photography costs by 62% by implementing DALL-E 3 to generate product visualization in multiple room settings and color variations.
Implementation:
- Created parametric prompts allowing automatic generation of each product in different environments
- Developed a style guide ensuring consistent lighting and composition
- Integrated image generation directly into their product management system
2. Content Marketing and Social Media
Case Study: Digital marketing agency Contentify increased client engagement by 47% after implementing AI-generated custom illustrations for blog posts and social media content.
Implementation:
- Developed prompt templates for consistent brand-aligned imagery
- Created a library of base images that could be customized for different topics
- Integrated image generation into their content calendar workflow
3. Architectural and Interior Design Visualization
Case Study: Architecture firm Studio Moderna reduced concept visualization time from days to hours by using DALL-E 3 for early-stage client presentations.
Implementation:
- Created detailed prompts incorporating architectural specifications
- Developed a multi-stage process where initial concepts are refined through iterations
- Combined AI-generated concepts with traditional rendering for final presentations
4. Educational Content Creation
Case Study: EdTech company LearnSmart increased student comprehension by 34% after incorporating custom AI visualizations for complex concepts in their science curriculum.
Implementation:
- Created a systematic approach for visualizing abstract scientific concepts
- Developed age-appropriate visual styles for different grade levels
- Implemented feedback loops where student engagement metrics informed visual refinements
5. User Interface Design and Prototyping
Case Study: Software development firm AppWorks reduced UI mockup creation time by 78% using GPT-4o for rapid interface visualization.
Implementation:
- Created detailed prompt templates for different interface components
- Developed a component-based approach allowing mix-and-match of UI elements
- Implemented a workflow where AI-generated mockups informed final design implementation
Legal and Ethical Considerations
When using OpenAI’s image generation tools, be aware of these important considerations:
Usage Rights and Licensing
- OpenAI grants full usage rights to images generated through their API, including commercial use
- You can modify, distribute, and sell derived products without attribution requirements
- OpenAI retains the right to use generated images for service improvement
Important: While OpenAI grants usage rights, the legal landscape around AI-generated content is evolving. Consider consulting legal counsel for critical commercial applications.
Content Restrictions
OpenAI enforces restrictions on generating:
- Content that depicts public figures realistically
- Hateful, harassing, or violent content
- Adult or sexually explicit material
- Content that promotes illegal activities
- Content attempting to influence political processes
Transparency Best Practices
Consider adopting these ethical best practices:
- Disclose when content is AI-generated, particularly in sensitive contexts
- Maintain human oversight for critical applications
- Establish internal policies for appropriate AI image generation use
Frequently Asked Questions
Can I use OpenAI-generated images commercially?
Yes, OpenAI grants full usage rights, including commercial use, for all images generated through their API. You can modify, sell, and distribute these images without attribution requirements.
Which is better for image generation: DALL-E 3 or GPT-4o?
DALL-E 3 generally produces higher resolution images with more precise style control, while GPT-4o excels at understanding complex concepts and rendering text accurately within images. For maximum quality, use DALL-E 3 for standalone images and GPT-4o when you need conversational guidance or text-heavy images.
How can I upload my own images to guide the generation process?
Currently, OpenAI doesn’t support direct “image-to-image” functionality where you can upload an image to guide generation. However, you can use the variations API to create alternative versions of images you’ve already generated.
Are there any limits on API usage for image generation?
Yes, OpenAI implements rate limits based on your account tier and usage history. New accounts typically have lower limits that increase over time with established usage patterns. Enterprise customers can request higher limits. API bridge services like laozhang.ai often have more generous rate limits for new accounts.
Can OpenAI generate images with transparent backgrounds?
No, currently neither DALL-E 3 nor GPT-4o supports generating images with transparent backgrounds. All images are generated with full backgrounds. For transparent backgrounds, you’ll need to process the images with additional graphics software.
How do I avoid content policy violations when generating images?
Be specific about what you want rather than what you don’t want to avoid triggering filters. Avoid prompts involving public figures, violent imagery, adult content, or deceptive content. Focus on positive descriptions and ethical use cases.
Conclusion: The Future of AI Image Generation
OpenAI’s image generation capabilities have reached an inflection point in 2025, offering unprecedented creative possibilities for businesses and developers. The combination of DALL-E 3’s specialized image generation and GPT-4o’s integrated multimodal approach provides a powerful toolkit for visual content creation.
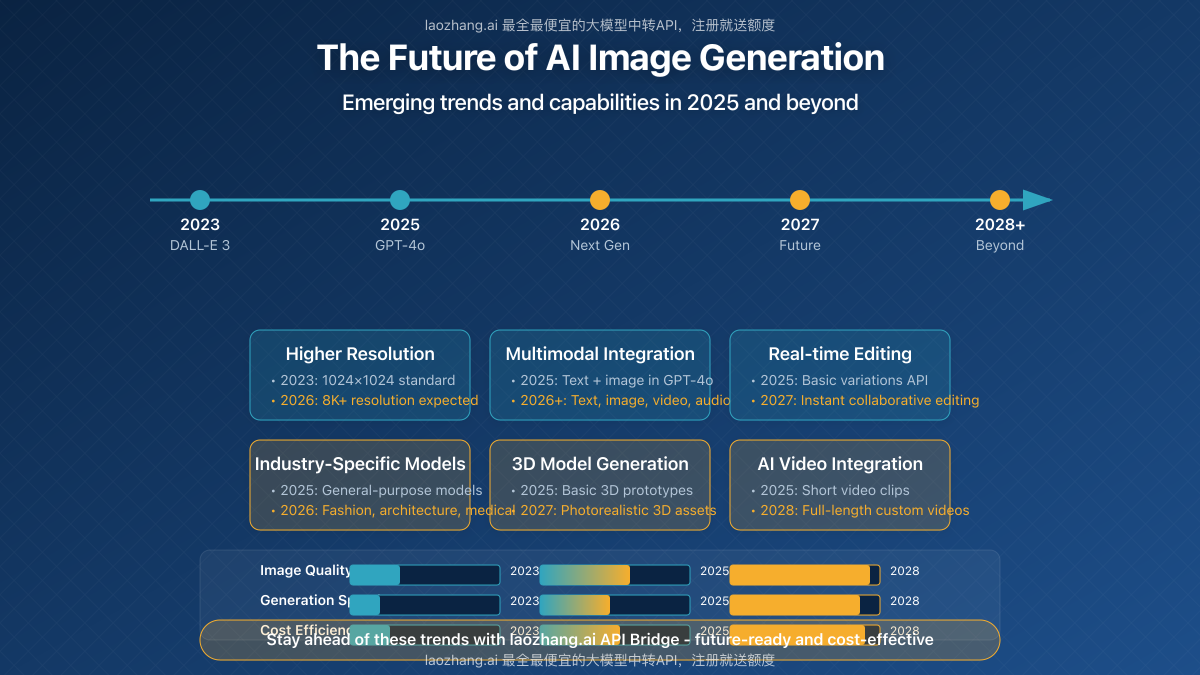
By implementing the strategies outlined in this guide—particularly leveraging cost-effective API bridge solutions like laozhang.ai—you can harness these technologies while optimizing your expenditure and development resources.
For businesses and developers looking to stay competitive, integrating AI image generation is no longer optional—it’s rapidly becoming the standard for efficient visual content creation across industries.
Ready to get started? Register for laozhang.ai’s API bridge service today and receive free credits to begin experimenting with OpenAI’s image generation capabilities: https://api.laozhang.ai/register/?aff_code=JnIT
For technical support or questions, contact: WeChat ID: ghj930213