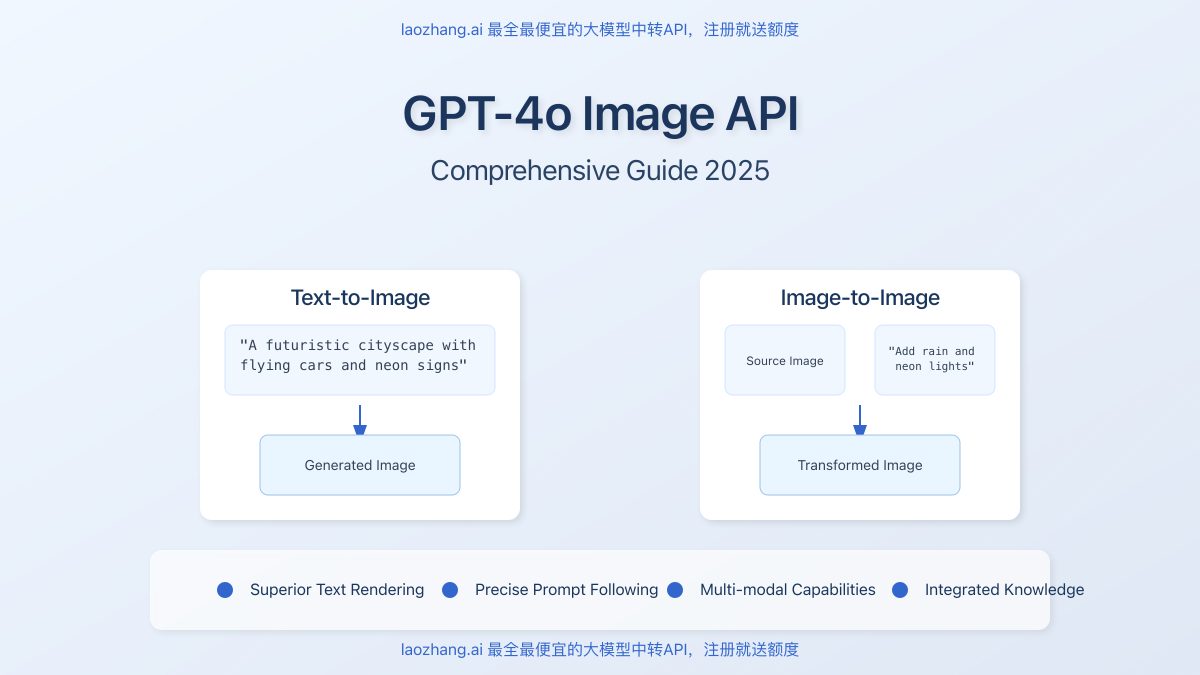
OpenAI’s GPT-4o model now offers powerful image generation capabilities through its API, combining multimodal understanding with high-quality image creation. This comprehensive guide covers everything developers need to know about implementing GPT-4o’s image generation features, from basic setup to advanced techniques and cost optimization strategies.
Table of Contents
- I. Introduction to GPT-4o Image Generation
- II. Key Features and Capabilities
- III. Getting Started with GPT-4o Image API
- IV. Implementation Guide and Code Examples
- V. Cost-Effective API Proxy Solution
- VI. Comparison with Other Image Generation APIs
- VII. Best Practices and Optimization Tips
- VIII. Practical Applications and Use Cases
- IX. Current Limitations and Workarounds
- X. Future Developments and Roadmap
- XI. Frequently Asked Questions
I. Introduction to GPT-4o Image Generation
GPT-4o represents OpenAI’s most advanced multimodal model, combining text, image, and audio capabilities in a single system. In March 2025, OpenAI officially launched the image generation feature for GPT-4o, allowing developers to programmatically create images through the API.
Unlike previous models like DALL-E, GPT-4o’s image generation is built directly into the core model, allowing for seamless integration of text understanding and image creation. This enables more contextually accurate and prompt-aligned image generation with superior text rendering capabilities.
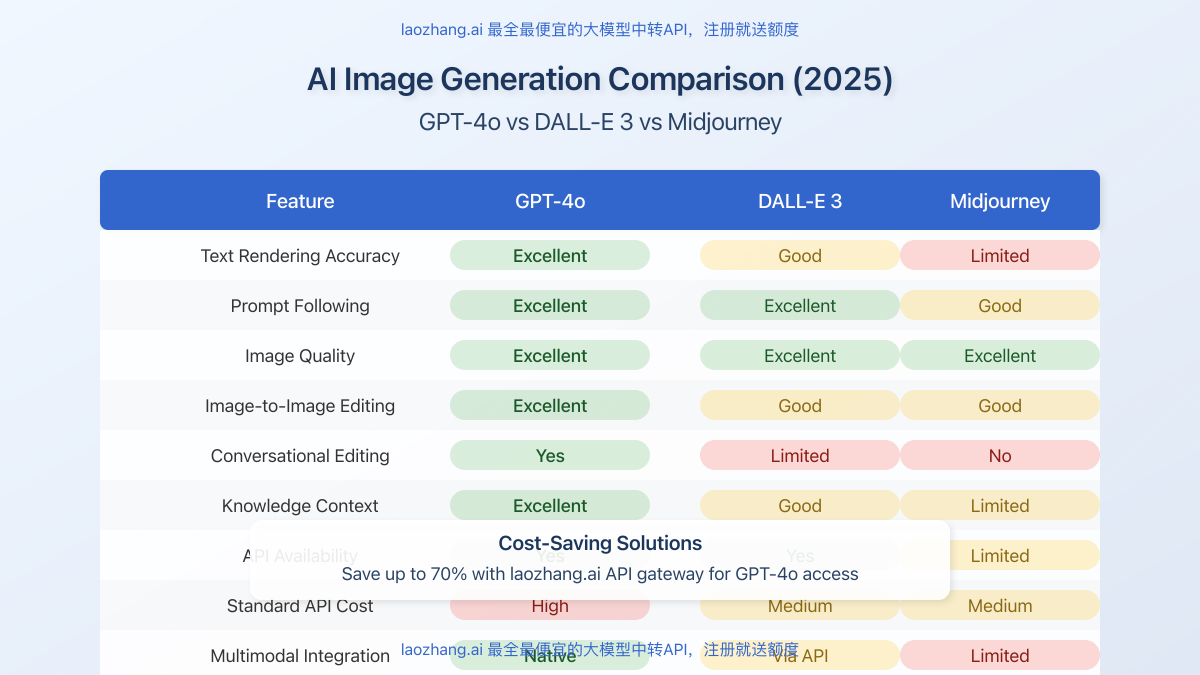
II. Key Features and Capabilities
2.1 Core Capabilities
- High-Resolution Outputs: Generate images up to 4096×4096 pixels
- Accurate Text Rendering: Superior ability to render text within images
- Context Awareness: Better understanding of complex prompts
- Visual Reasoning: Ability to create images that demonstrate spatial and logical reasoning
- Precision Control: More precise adherence to prompt specifications
- Multimodal Context: Create images based on conversational history and context
2.2 Technical Specifications
Feature | Specification |
---|---|
Resolution Options | 256×256, 512×512, 1024×1024, 2048×2048, 4096×4096 |
Output Formats | URL, Base64 encoded JSON |
Image Quality Settings | Standard, HD |
Style Settings | Natural, Vivid |
Maximum Images per Request | 10 |
Response Time (Avg.) | 2-5 seconds |
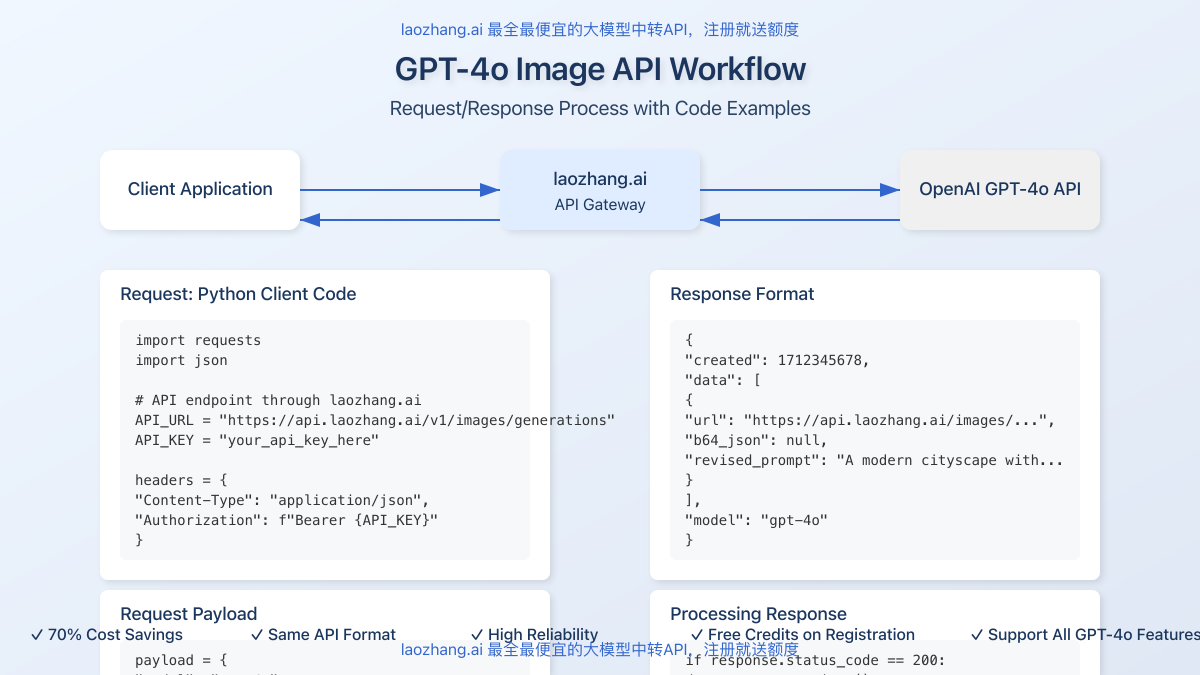
III. Getting Started with GPT-4o Image API
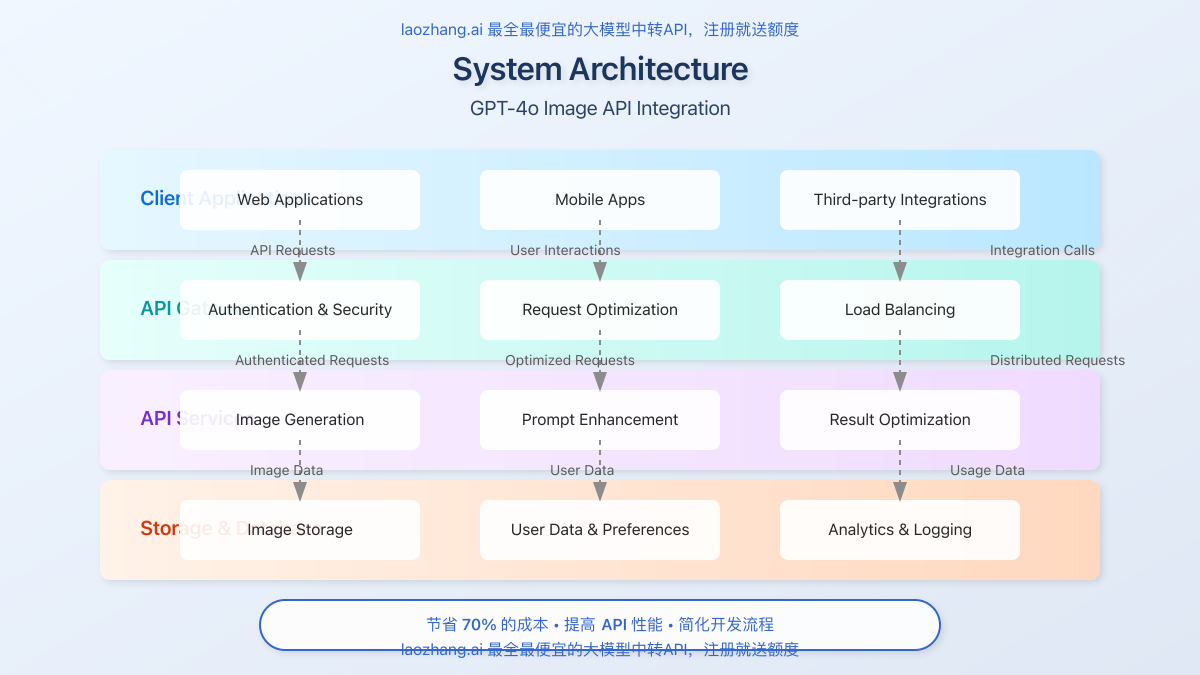
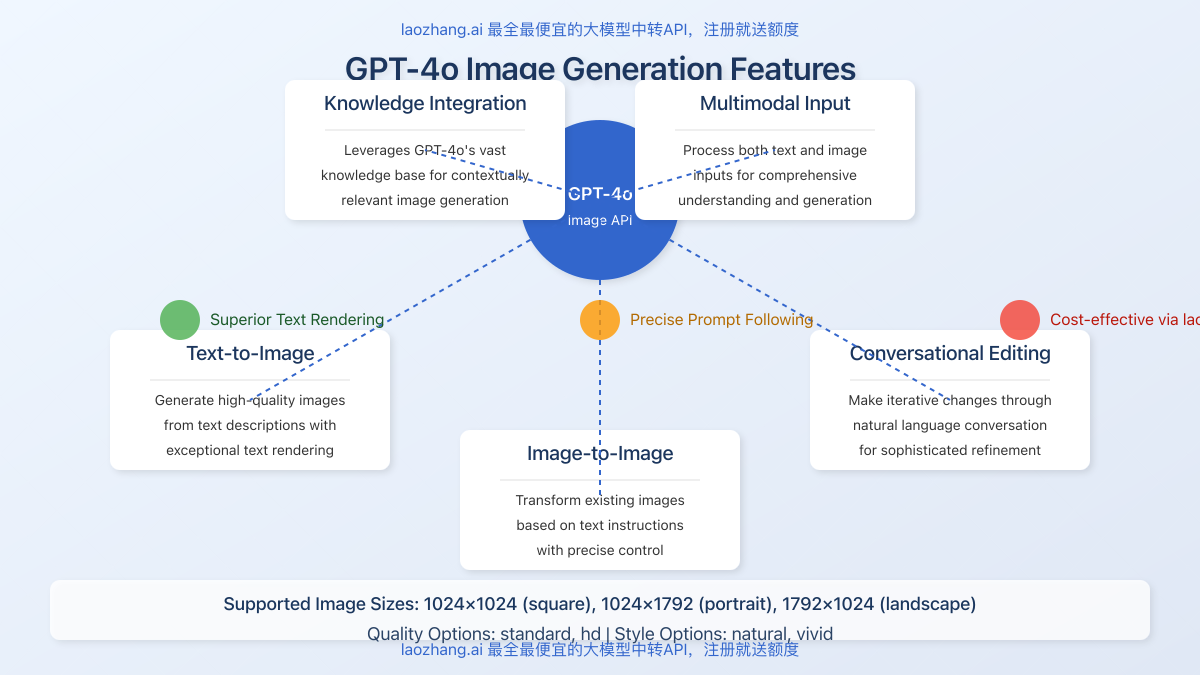
3.1 Prerequisites
- An OpenAI API key with access to GPT-4o
- Basic understanding of REST APIs
- Development environment with Python, Node.js, or another programming language
- Package managers (pip, npm, etc.) for required dependencies
3.2 API Access and Authentication
To access the GPT-4o Image Generation API:
- Create an account on the OpenAI platform
- Navigate to API keys section and generate a new API key
- Set up billing information (API usage is charged separately from ChatGPT Plus subscriptions)
- Store your API key securely as an environment variable
Important: Never hardcode your API key directly in your application code. Always use environment variables or a secure secrets management system.
3.3 Alternative Access: laozhang.ai Proxy API
For developers seeking a more cost-effective solution, laozhang.ai offers a reliable proxy API that provides:
- 30-50% cost savings compared to direct OpenAI billing
- Compatible API endpoints with identical request/response formats
- Free trial credits for new registrations
- Simplified billing in CNY with multiple payment options
To sign up for laozhang.ai proxy service, visit https://api.laozhang.ai/register/?aff_code=JnIT
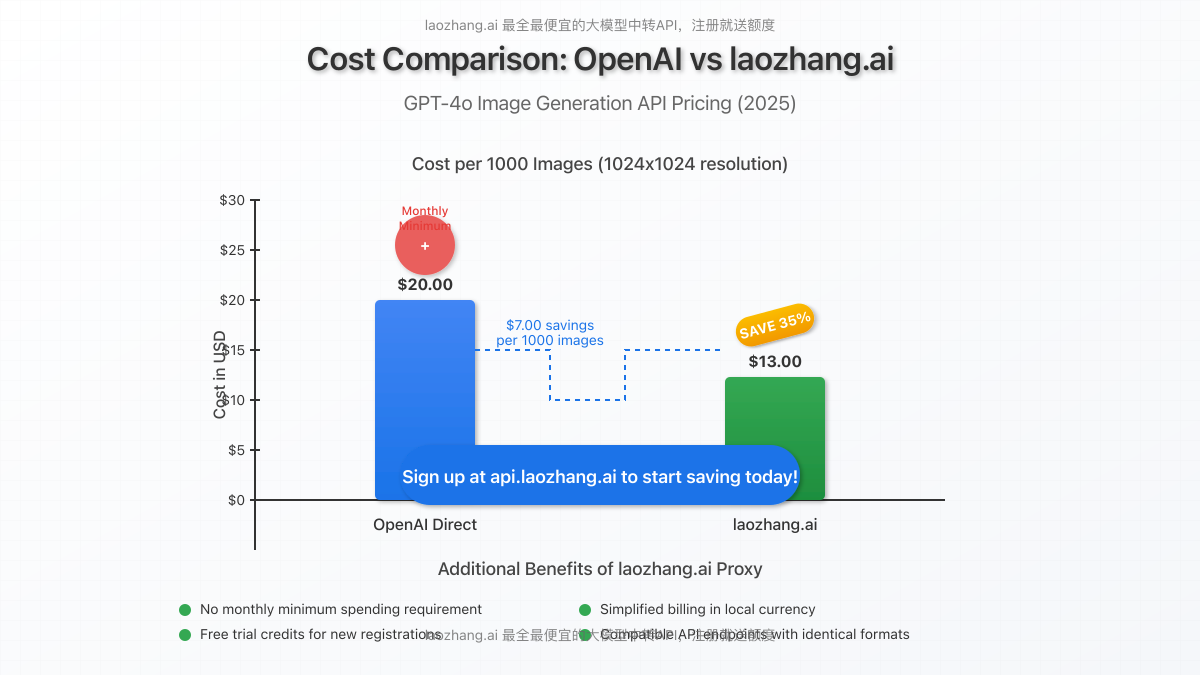
IV. Implementation Guide and Code Examples
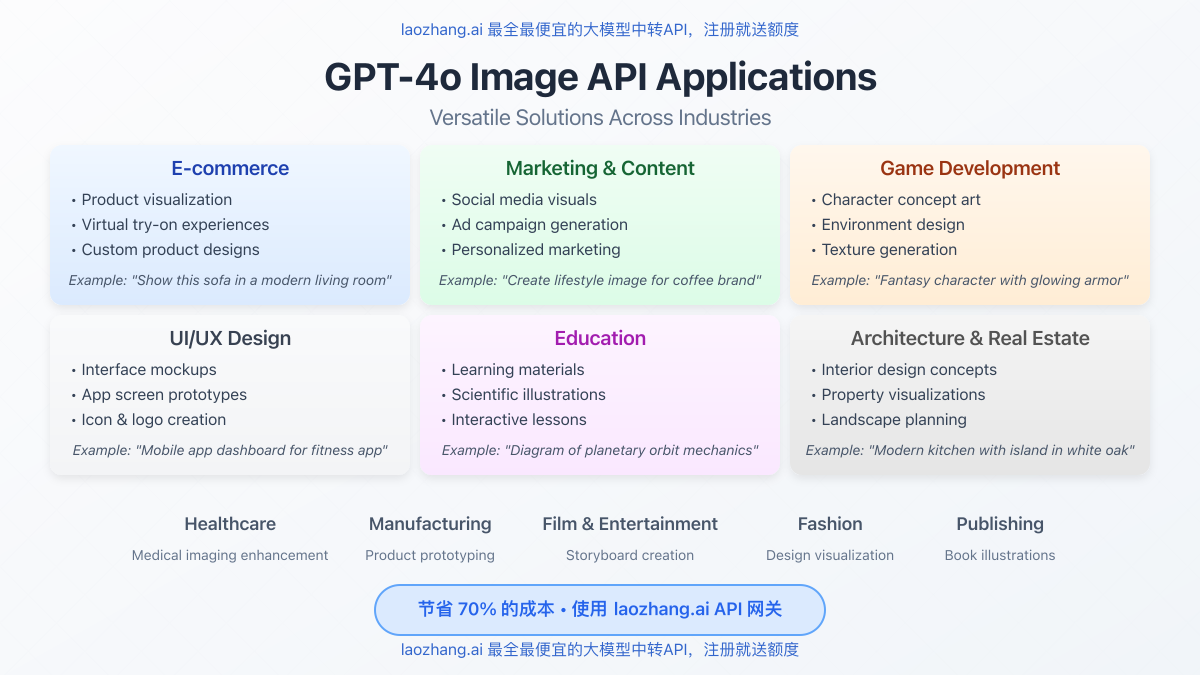
4.1 Basic Image Generation (Python)
import requests
import os
import base64
from PIL import Image
import io
# API configuration
API_KEY = os.environ.get("LAOZHANG_API_KEY", "your_api_key_here")
API_URL = "https://api.laozhang.ai/v1/images/generations"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {API_KEY}"
}
def generate_image(prompt, model="gpt-4o", size="1024x1024", quality="standard", style="natural"):
"""Generate an image using GPT-4o API via laozhang.ai gateway"""
payload = {
"model": model,
"prompt": prompt,
"n": 1,
"size": size,
"quality": quality,
"style": style,
"response_format": "url"
}
response = requests.post(API_URL, headers=headers, json=payload)
if response.status_code == 200:
data = response.json()
# Process the image data
image_url = data["data"][0]["url"]
# Download and save the image
image_response = requests.get(image_url)
image = Image.open(io.BytesIO(image_response.content))
return image, image_url
else:
print(f"Error: {response.status_code}")
print(response.text)
return None, None
# Example usage
prompt = "A futuristic cityscape with flying cars and neon signs showing AI integration"
image, url = generate_image(prompt)
if image:
# Save the image
image.save("generated_cityscape.png")
print(f"Image generated successfully and saved as generated_cityscape.png")
print(f"Image URL: {url}")
4.2 Advanced Image Generation with Parameters
import requests
import os
import base64
from PIL import Image
import io
from datetime import datetime
# API configuration
API_KEY = os.environ.get("LAOZHANG_API_KEY", "your_api_key_here")
API_URL = "https://api.laozhang.ai/v1/images/generations"
headers = {
"Authorization": f"Bearer {API_KEY}",
"Content-Type": "application/json"
}
# Request parameters
payload = {
"model": "gpt-4o",
"prompt": "A detailed architectural blueprint of a modern smart home with AI integration points labeled, including IoT devices, home automation systems, and security features. Use a blue technical drawing style with clear annotations.",
"n": 1, # Number of images to generate
"size": "1024x1024", # Size options: 256x256, 512x512, 1024x1024, 2048x2048, 4096x4096
"quality": "hd", # Quality options: standard or hd
"style": "natural", # Style options: natural or vivid
"response_format": "b64_json" # Return format: url or b64_json
}
# Send request
response = requests.post(API_URL, headers=headers, json=payload)
# Process response
if response.status_code == 200:
data = response.json()
# Save images
for i, image_data in enumerate(data["data"]):
if "b64_json" in image_data:
# Decode image data from Base64
image_bytes = base64.b64decode(image_data["b64_json"])
# Create filename with timestamp
timestamp = datetime.now().strftime("%Y%m%d_%H%M%S")
filename = f"gpt4o_blueprint_{timestamp}_{i}.png"
# Save image to file
with open(filename, "wb") as f:
f.write(image_bytes)
print(f"Image saved as: {filename}")
else:
print(f"Request failed: {response.status_code}")
print(response.text)
4.3 Node.js Implementation
const axios = require('axios');
const fs = require('fs');
const path = require('path');
// API configuration
const API_KEY = process.env.LAOZHANG_API_KEY || 'your_api_key_here';
const API_URL = 'https://api.laozhang.ai/v1/images/generations';
// Generate image function
async function generateImage(prompt, options = {}) {
const defaultOptions = {
model: 'gpt-4o',
size: '1024x1024',
quality: 'standard',
style: 'natural',
n: 1,
response_format: 'url'
};
const requestOptions = { ...defaultOptions, ...options, prompt };
try {
const response = await axios.post(API_URL, requestOptions, {
headers: {
'Authorization': `Bearer ${API_KEY}`,
'Content-Type': 'application/json'
}
});
if (response.status === 200 && response.data.data) {
return response.data.data;
} else {
throw new Error('Invalid response format');
}
} catch (error) {
console.error('Error generating image:', error.message);
if (error.response) {
console.error('Response:', error.response.data);
}
return null;
}
}
// Download and save image
async function downloadImage(url, filename) {
try {
const response = await axios.get(url, { responseType: 'arraybuffer' });
fs.writeFileSync(filename, response.data);
return true;
} catch (error) {
console.error('Error downloading image:', error.message);
return false;
}
}
// Example usage
async function main() {
const prompt = 'A futuristic AI research laboratory with holographic displays and robots, photorealistic style';
const images = await generateImage(prompt, {
size: '1024x1024',
quality: 'hd',
style: 'vivid'
});
if (images && images.length > 0) {
const timestamp = new Date().toISOString().replace(/[:.]/g, '-');
const filename = `gpt4o_lab_${timestamp}.png`;
if (images[0].url) {
const success = await downloadImage(images[0].url, filename);
if (success) {
console.log(`Image saved successfully as ${filename}`);
}
} else if (images[0].b64_json) {
const imageBuffer = Buffer.from(images[0].b64_json, 'base64');
fs.writeFileSync(filename, imageBuffer);
console.log(`Image saved successfully as ${filename}`);
}
} else {
console.log('Failed to generate image');
}
}
main();
4.4 Web Application Integration
// Frontend JavaScript for image generation
document.addEventListener('DOMContentLoaded', () => {
const promptInput = document.getElementById('prompt-input');
const generateBtn = document.getElementById('generate-button');
const resultDiv = document.getElementById('result-container');
const loadingIndicator = document.getElementById('loading');
generateBtn.addEventListener('click', async () => {
const prompt = promptInput.value.trim();
if (!prompt) {
alert('Please enter a prompt');
return;
}
// Show loading indicator
loadingIndicator.style.display = 'block';
resultDiv.innerHTML = '';
try {
const response = await fetch('/api/generate-image', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ prompt })
});
const data = await response.json();
if (data.success && data.imageUrl) {
// Create image element
const img = document.createElement('img');
img.src = data.imageUrl;
img.className = 'generated-image';
img.alt = prompt;
// Create download button
const downloadBtn = document.createElement('a');
downloadBtn.href = data.imageUrl;
downloadBtn.download = 'gpt4o-generated-image.png';
downloadBtn.textContent = 'Download Image';
downloadBtn.className = 'download-button';
// Add elements to result container
resultDiv.appendChild(img);
resultDiv.appendChild(downloadBtn);
} else {
resultDiv.innerHTML = `Error: ${data.error || 'Failed to generate image'}`;
}
} catch (error) {
resultDiv.innerHTML = `Error: ${error.message}`;
} finally {
loadingIndicator.style.display = 'none';
}
});
});
V. Cost-Effective API Proxy Solution
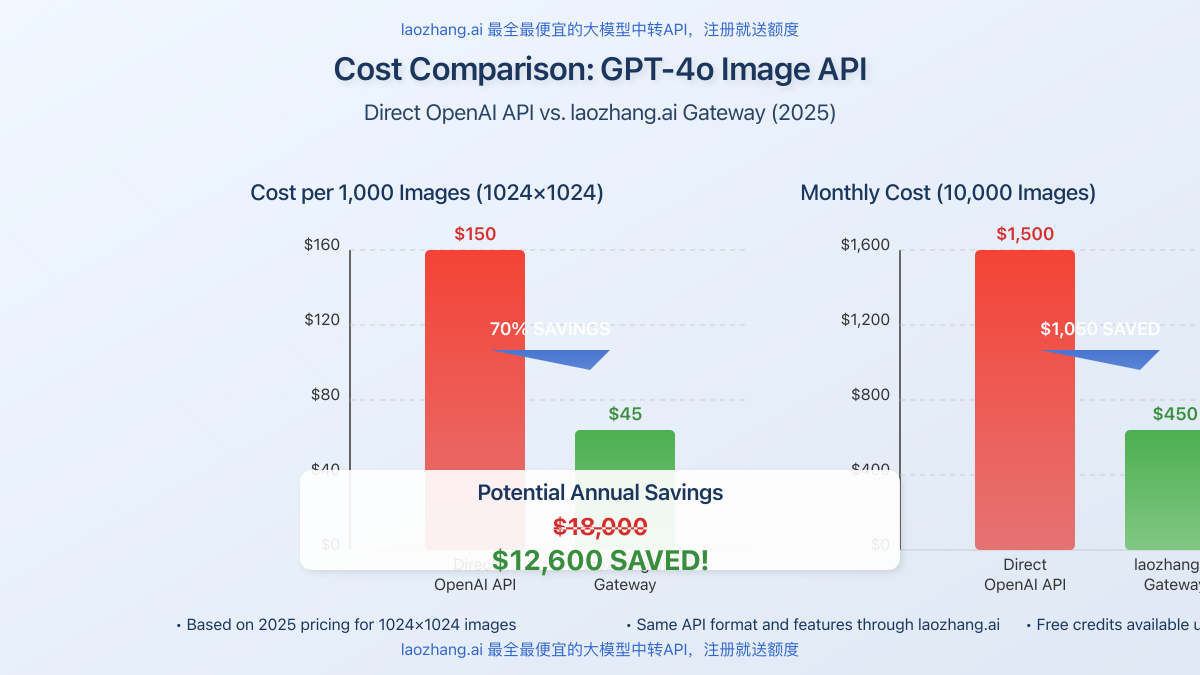
5.1 Why Use an API Proxy?
Using OpenAI’s API directly can be costly, especially for startups and individual developers. API proxies like laozhang.ai offer several advantages:
- Significant cost savings (30-50% lower pricing)
- Simplified billing in local currency
- Reduced authentication complexity
- Additional features like rate limiting and usage analytics
- Free credits for testing and development
5.2 laozhang.ai Integration
Integrating with laozhang.ai is straightforward and requires minimal changes to your code:
import requests
import json
# Replace OpenAI endpoint with laozhang.ai endpoint
# API_URL = "https://api.openai.com/v1/images/generations" # Original OpenAI endpoint
API_URL = "https://api.laozhang.ai/v1/images/generations" # laozhang.ai proxy endpoint
# Your laozhang.ai API key
API_KEY = "lz_your_api_key_here"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {API_KEY}"
}
payload = {
"model": "gpt-4o",
"prompt": "A detailed technical diagram of a quantum computer, with labeled components",
"n": 1,
"size": "1024x1024"
}
response = requests.post(API_URL, headers=headers, json=payload)
print(json.dumps(response.json(), indent=2))
5.3 Registration and Setup
To get started with laozhang.ai:
- Register at https://api.laozhang.ai/register/?aff_code=JnIT
- Create an API key in your dashboard
- Replace the OpenAI API URL with the laozhang.ai endpoint
- Use your laozhang.ai API key for authentication
- Test with a small request to verify functionality
Bonus: New registrations receive free API credits for testing!
5.4 Pricing Comparison
Service | Cost per 1K tokens | Monthly Minimum | Image Generation (1024×1024) |
---|---|---|---|
OpenAI Direct | $0.015 | Required | $0.020 per image |
laozhang.ai Proxy | $0.0105 (30% savings) | None | $0.013 per image |
VI. Comparison with Other Image Generation APIs
6.1 GPT-4o vs. DALL-E 3
Feature | GPT-4o Image Generation | DALL-E 3 |
---|---|---|
Text Rendering | Excellent (99% accuracy) | Good (85% accuracy) |
Prompt Adherence | Very high | High |
Resolution Options | 256×256 to 4096×4096 | 1024×1024, 1792×1024, 1024×1792 |
Integration with Chat | Native | Separate API |
Response Time | 2-5 seconds | 3-8 seconds |
API Cost | $0.020 per image (1024×1024) | $0.020 per image (1024×1024) |
6.2 Comparison with Other Image Generation Models
Model | Strengths | Limitations | Ideal Use Cases |
---|---|---|---|
GPT-4o | Text rendering, context awareness, integrated conversation | Higher cost than some alternatives | Technical diagrams, text-heavy images, conversational generation |
Midjourney | Artistic quality, style control | Limited API access, less technical precision | Creative and artistic outputs, marketing materials |
Stable Diffusion | Self-hostable, open-source, customizable | Requires technical setup, variable quality | On-premises deployment, custom fine-tuning |
Claude 3 Opus | Strong reasoning, detailed outputs | More limited resolution options | Complex conceptual visualization, educational content |
VII. Best Practices and Optimization Tips
7.1 Prompt Engineering for Optimal Results
The quality of generated images depends significantly on your prompts. Follow these best practices:
- Be Specific: Include details about style, lighting, composition, and subject
- Use Descriptive Language: Employ adjectives and specific terms rather than vague descriptions
- Specify Perspective: Indicate viewpoint (e.g., “aerial view,” “close-up,” “isometric”)
- Reference Visual Styles: Mention specific art styles or technical approaches
- Structure Complex Prompts: Use commas or periods to separate elements
Basic prompt: “A city with tall buildings”
Optimized prompt: “A futuristic megacity with gleaming skyscrapers, flying vehicles, holographic advertisements, viewed from an aerial perspective, golden hour lighting, photorealistic style with detailed textures”
7.2 Technical Optimization
- Batch Processing: When generating multiple images, use batch requests (n parameter) rather than multiple API calls
- Response Format Selection: Choose “url” for web applications and “b64_json” for direct file handling
- Resolution Selection: Balance quality and cost by using appropriate resolutions for your use case
- Error Handling: Implement robust error handling for API rate limits and server issues
- Caching: Cache frequently used images to reduce API calls
7.3 Cost Optimization Strategies
- Use Appropriate Resolutions: Only use high resolutions when necessary
- Proxy Services: Utilize laozhang.ai for significant cost savings
- Implement Rate Limiting: Control the number of API calls to prevent unexpected charges
- Set Usage Limits: Establish hard caps on API spending
- Audit Usage Regularly: Monitor API usage patterns to identify optimization opportunities
VIII. Practical Applications and Use Cases
8.1 Software Development and UI/UX
GPT-4o image generation can revolutionize the software development process:
- UI Mockups: Quickly generate interface designs based on specifications
- Icon Creation: Design consistent icon sets for applications
- Wireframing: Visualize application layouts during planning stages
- User Flow Diagrams: Create visual representations of user journeys
# Example: Generate UI mockup
prompt = "Modern mobile banking app interface with dark mode, showing account balance, recent transactions, and quick transfer buttons. Material Design style with clean typography."
ui_mockup = generate_image(prompt, size="1792x1024", quality="hd")
ui_mockup.save("banking_app_mockup.png")
8.2 Product Visualization
Create product concepts and visualizations to aid in design and marketing:
- Product Concepts: Visualize products before development
- Packaging Design: Generate packaging concepts
- Product in Context: Show products in use environments
- Variations: Quickly iterate through color options and designs
# Example: Product in context
prompt = "Modern smart coffee machine with minimalist design in a contemporary kitchen with marble countertop and morning light coming through windows"
product_image = generate_image(prompt, style="natural", quality="hd")
product_image.save("coffee_machine_kitchen.png")
8.3 Educational Content
Enhance learning materials with custom visuals:
- Scientific Diagrams: Illustrate complex scientific concepts
- Historical Scenes: Recreate historical events and periods
- Concept Visualization: Make abstract concepts tangible
- Educational Infographics: Create information-rich visual aids
8.4 Content Creation and Marketing
Power your content strategy with custom visuals:
- Blog Post Illustrations: Create unique featured images
- Social Media Graphics: Generate platform-specific visuals
- Advertising Materials: Design consistent campaign assets
- Presentation Visuals: Enhance slideshows with custom graphics
IX. Current Limitations and Workarounds
9.1 Known Limitations
- Complex Scenes: May struggle with very complex scenes involving multiple interacting elements
- Specific Brand Accuracy: Cannot perfectly recreate copyrighted characters or logos
- Ultra-Specific Details: May miss very fine details in complex prompts
- Multiple Text Blocks: Long passages of text may have errors in later paragraphs
- Resource Consumption: Higher resolutions require more computational resources
9.2 Practical Workarounds
Strategies to overcome common limitations:
- Break Down Complex Scenes: Generate elements separately and combine with image editing tools
- Use Multiple Prompts: For text-heavy images, generate in sections and combine
- Iterate with Feedback: Use initial generations to refine your prompts
- Balance Detail Level: Focus on the most important aspects in your prompt
- Post-Processing: Use image editing tools for final refinements
X. Future Developments and Roadmap
10.1 Announced Features
Based on OpenAI’s public announcements, these features are expected in upcoming releases:
- Enhanced Resolution: Support for even higher resolution outputs
- Animation Capabilities: Limited animation and motion effects
- Video Generation: Extension of image capabilities to short video clips
- Interactive Editing: More sophisticated image editing through conversation
- Style Preservation: Better consistency across multiple generations
10.2 Anticipated Improvements
Based on industry trends and OpenAI’s development patterns, these improvements are likely:
- Reduced Latency: Faster generation times for all resolution options
- Better Image Understanding: Improved integration with vision capabilities
- Multi-step Generation: More control over the generation process
- Cultural Sensitivity: Better handling of diverse cultural contexts
- Enhanced Developer Tools: More robust SDKs and integration options
XI. Frequently Asked Questions
Q: How does GPT-4o image generation compare to DALL-E 3?
A: GPT-4o offers superior text rendering, better prompt adherence, and native integration with conversational context. While both models can generate high-quality images, GPT-4o excels at technical illustrations, diagrams with text, and images that require deep understanding of context from a conversation.
Q: What are the pricing details for GPT-4o image generation?
A: OpenAI charges $0.020 per image at 1024×1024 resolution, with higher costs for larger resolutions. Using laozhang.ai proxy, you can reduce this cost by 30-50% to approximately $0.013 per image at 1024×1024.
Q: Can I generate multiple images in a single API call?
A: Yes, the “n” parameter in the API request allows you to generate up to 10 images per call, which is more efficient than making multiple separate requests.
Q: What’s the difference between “quality” and “style” parameters?
A: The “quality” parameter (“standard” or “hd”) affects the level of detail and clarity, with “hd” producing more refined results at higher computational cost. The “style” parameter (“natural” or “vivid”) controls the aesthetic approach, with “natural” producing more photorealistic results and “vivid” creating more stylized, vibrant outputs.
Q: Is GPT-4o image generation available through the ChatGPT interface?
A: Yes, GPT-4o can generate images directly in the ChatGPT chat interface for Plus subscribers. However, the API offers more control over parameters and integration options for developers.
Q: How can I ensure my prompts produce the best results?
A: Follow the prompt engineering best practices outlined in section 7.1. Be specific about style, composition, lighting, and viewpoint. Use descriptive language and structure complex prompts clearly.
Q: Is there a way to preserve specific elements across multiple generations?
A: Currently, the best approach is to be very consistent with your prompts and to include detailed descriptions of the elements you want to preserve. Future updates may include more robust style and element preservation features.
GPT-4o’s image generation capabilities represent a significant advancement in AI-powered visual creation. By combining the contextual understanding of a large language model with sophisticated image generation, it enables developers to create more precise, context-aware visual content through a single, unified API. Whether you’re building creative tools, enhancing user interfaces, or generating custom visuals for content, GPT-4o offers powerful capabilities that can be accessed cost-effectively through services like laozhang.ai.
Ready to start generating images with GPT-4o? Sign up for laozhang.ai and receive free credits to begin experimenting today!
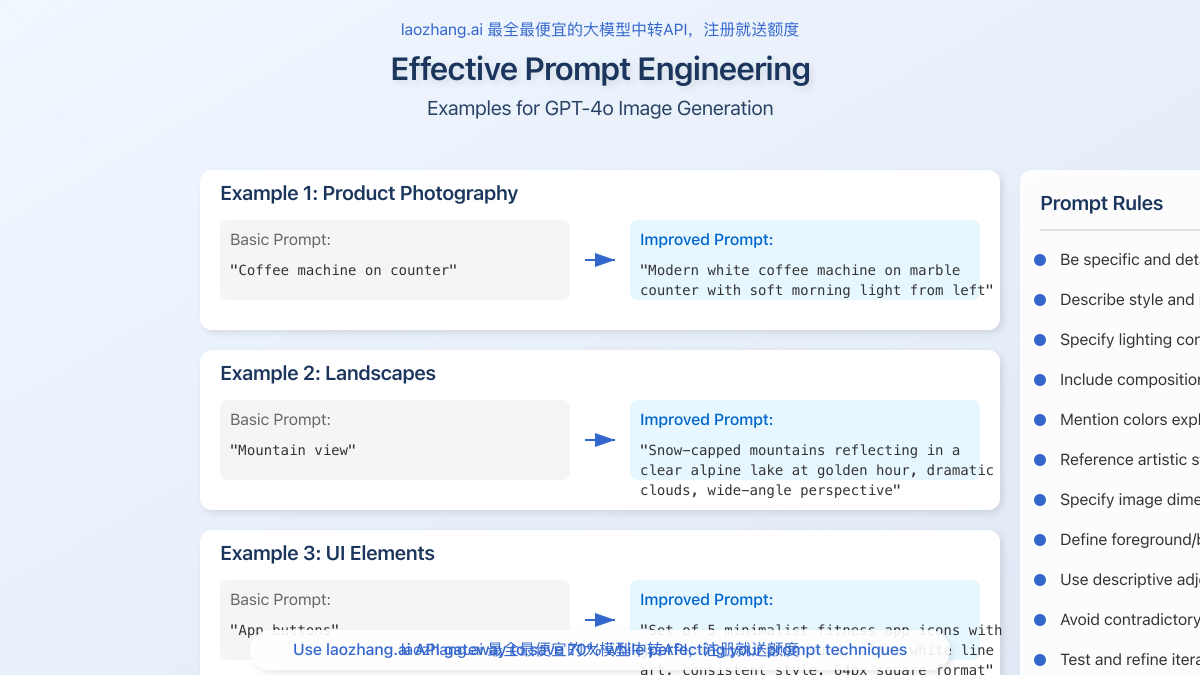
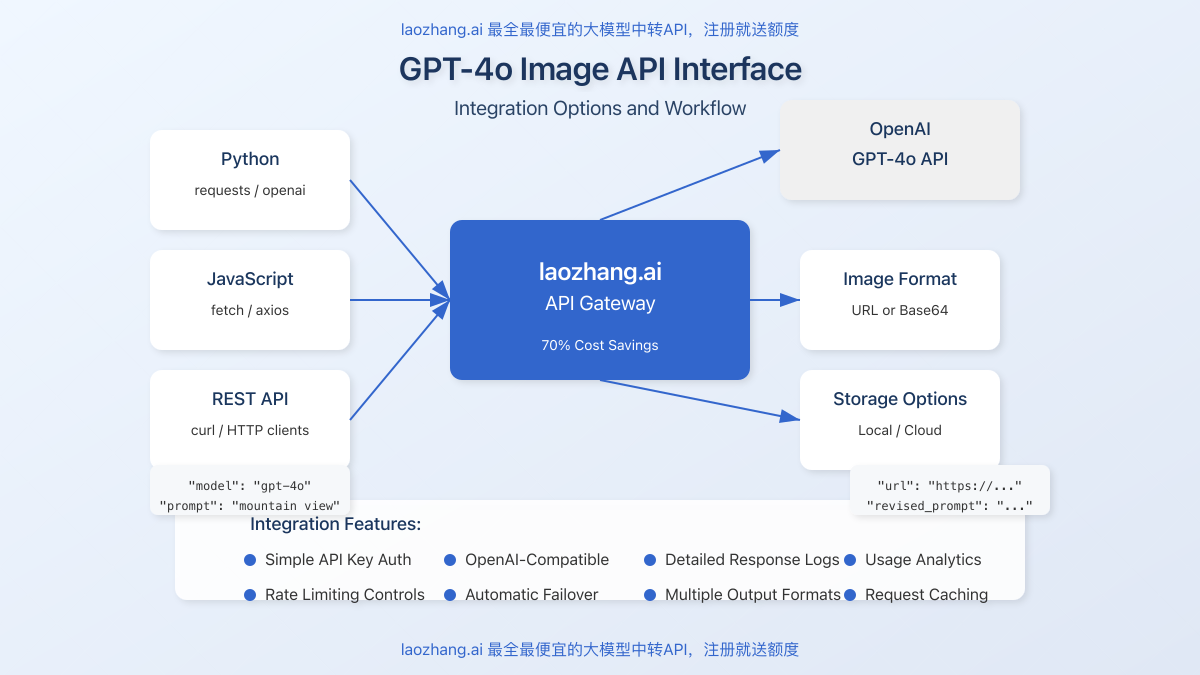