Free GPT O3 API in 2025: Ultimate Guide to Access OpenAI’s Advanced Reasoning Models
The release of OpenAI’s O3 Mini has revolutionized how developers can implement advanced AI reasoning capabilities in their applications. As of April 2025, there are several ways to access this powerful model without paying for API credits. This comprehensive guide explores legitimate methods to use O3 Mini API for free, complete with code examples and implementation tips.
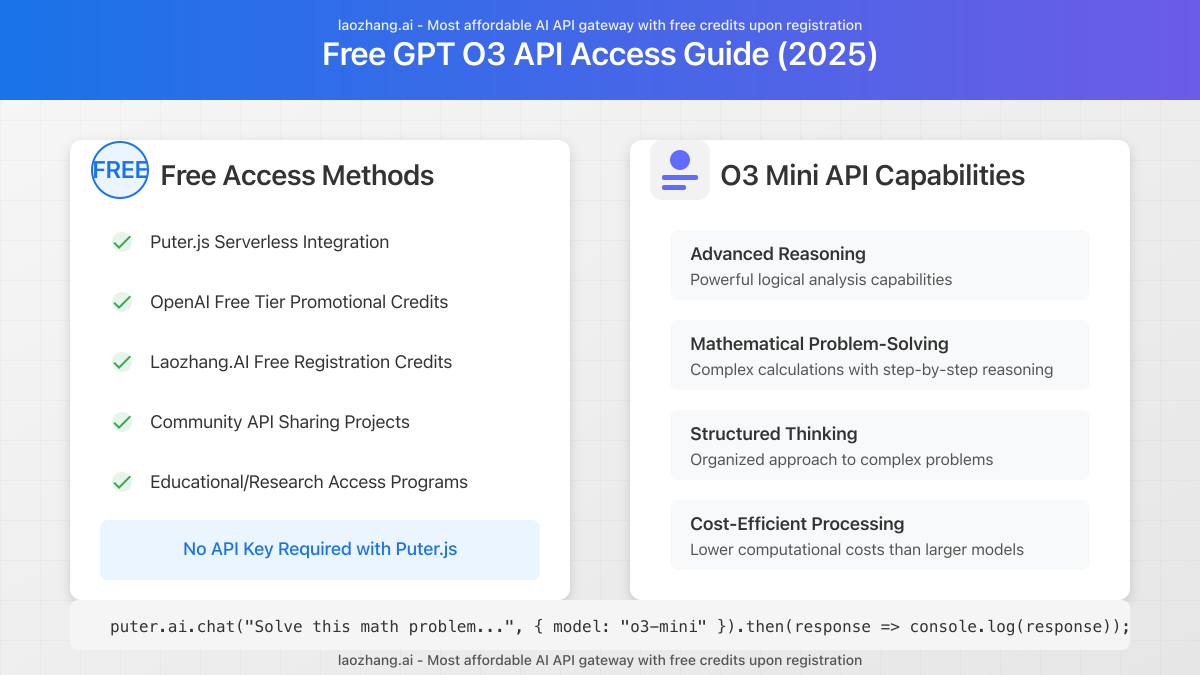
What is O3 Mini and Why It Matters
Released in January 2025, O3 Mini is OpenAI’s most cost-efficient reasoning model designed specifically for complex problem-solving tasks. Unlike traditional language models, O3 Mini excels at logical analysis, mathematical reasoning, and structured thinking—all while maintaining exceptional efficiency.
Key features that make O3 Mini stand out:
- Advanced reasoning capabilities at significantly lower computational costs
- Optimized for intricate problem-solving scenarios
- Superior performance in structured reasoning tasks compared to previous models
- Flexible integration options with existing systems and workflows
- Available through OpenAI’s standard API interfaces (Chat Completions, Assistants, Batch)
5 Ways to Access O3 Mini API for Free in 2025
Developers and researchers have several options to leverage O3 Mini’s capabilities without direct API costs. Let’s explore the most reliable methods:
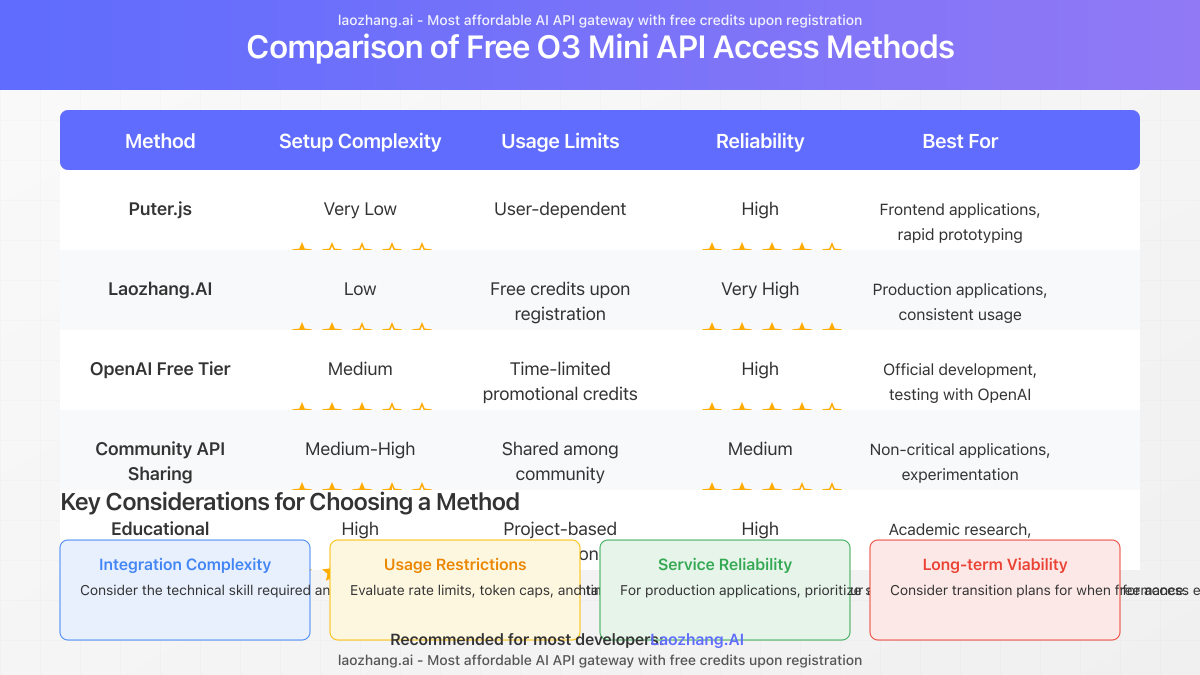
1. Puter.js: Serverless AI Integration
Puter.js offers a revolutionary “User Pays” model that allows developers to integrate O3 Mini capabilities without any API keys or server-side setup. This approach enables free access to OpenAI’s models directly from frontend code.
How to Implement Puter.js for O3 Mini Access:
Include the Puter.js script in your HTML file:
<script src="https://js.puter.com/v2/"></script>
Access O3 Mini with a simple function call:
// Using o3-mini model
puter.ai.chat(
"Solve this math problem: If a train travels at 60 mph for 2.5 hours, how far will it go?",
{ model: "o3-mini" }
).then(response => {
console.log(response);
});
Complete implementation example:
<html>
<body>
<div id="result"></div>
<script src="https://js.puter.com/v2/"></script>
<script>
// Using o3-mini model
puter.ai.chat(
"Solve this math problem: If a train travels at 60 mph for 2.5 hours, how far will it go?",
{ model: "o3-mini" }
).then(response => {
document.getElementById("result").innerText = response;
});
</script>
</body>
</html>
Benefits of Puter.js approach:
- No API key required
- Zero server-side setup
- Users cover their own usage costs
- Simple integration in frontend JavaScript
- Support for multiple OpenAI models including O3 Mini
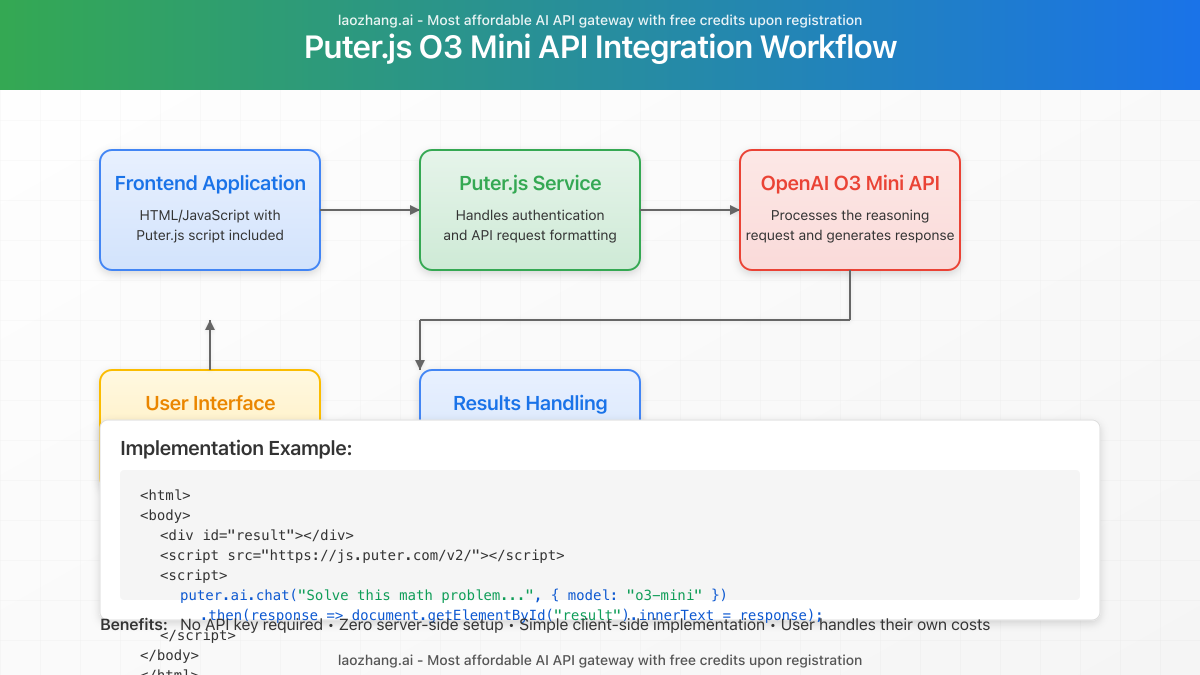
2. Laozhang.AI: Cost-Effective API Gateway
Laozhang.AI provides a reliable and affordable API gateway service for accessing OpenAI’s models, including O3 Mini. While not completely free, it offers significant cost savings compared to direct OpenAI API usage, plus free credits upon registration.
Getting Started with Laozhang.AI:
- Register for an account at https://api.laozhang.ai/register/?aff_code=JnIT
- Receive free API credits upon registration
- Generate an API key from your dashboard
- Implement API calls using standard OpenAI SDK patterns
Example API request with Laozhang.AI:
curl -X POST "https://api.laozhang.ai/v1/chat/completions" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "o3-mini",
"stream": false,
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": "Analyze the logical fallacies in this argument: All birds can fly. Penguins are birds. Therefore, penguins can fly."
}
]
}
]
}'
Python implementation with Laozhang.AI gateway:
import requests
import json
api_key = "your_laozhang_api_key"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}"
}
payload = {
"model": "o3-mini",
"stream": False,
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": "Analyze the logical fallacies in this argument: All birds can fly. Penguins are birds. Therefore, penguins can fly."
}
]
}
]
}
response = requests.post(
"https://api.laozhang.ai/v1/chat/completions",
headers=headers,
json=payload
)
print(json.dumps(response.json(), indent=2))
Key advantages of Laozhang.AI:
- Significantly lower costs compared to direct OpenAI API usage
- Free initial credits for testing and development
- Support for all OpenAI models including O3 Mini
- Standard API compatibility with OpenAI’s documentation
- Reliable and stable service with excellent uptime
- Transparent pricing structure without hidden fees
3. OpenAI’s Free Tier Access
OpenAI occasionally provides limited free access to their newest models through promotional periods. For O3 Mini, they initially offered a free tier with usage limits to encourage developer adoption.
How to access OpenAI’s free tier for O3 Mini:
- Create an OpenAI account at https://platform.openai.com
- Check for available promotional credits in your account dashboard
- Generate an API key under the API Keys section
- Monitor your usage to stay within free tier limits
import openai
# Configure the OpenAI API key
openai.api_key = "your_openai_api_key"
# Make a request to the O3 Mini model
response = openai.chat.completions.create(
model="o3-mini",
messages=[
{"role": "system", "content": "You are a logical reasoning assistant."},
{"role": "user", "content": "Evaluate whether this statement is true or false: If it's raining, then the ground is wet. The ground is wet. Therefore, it's raining."}
]
)
print(response.choices[0].message.content)
Important considerations for OpenAI’s free tier:
- Limited credit amount that expires after a set period
- Usage caps to prevent overutilization
- Temporary availability subject to OpenAI’s promotional decisions
- Requires creating and managing an OpenAI account
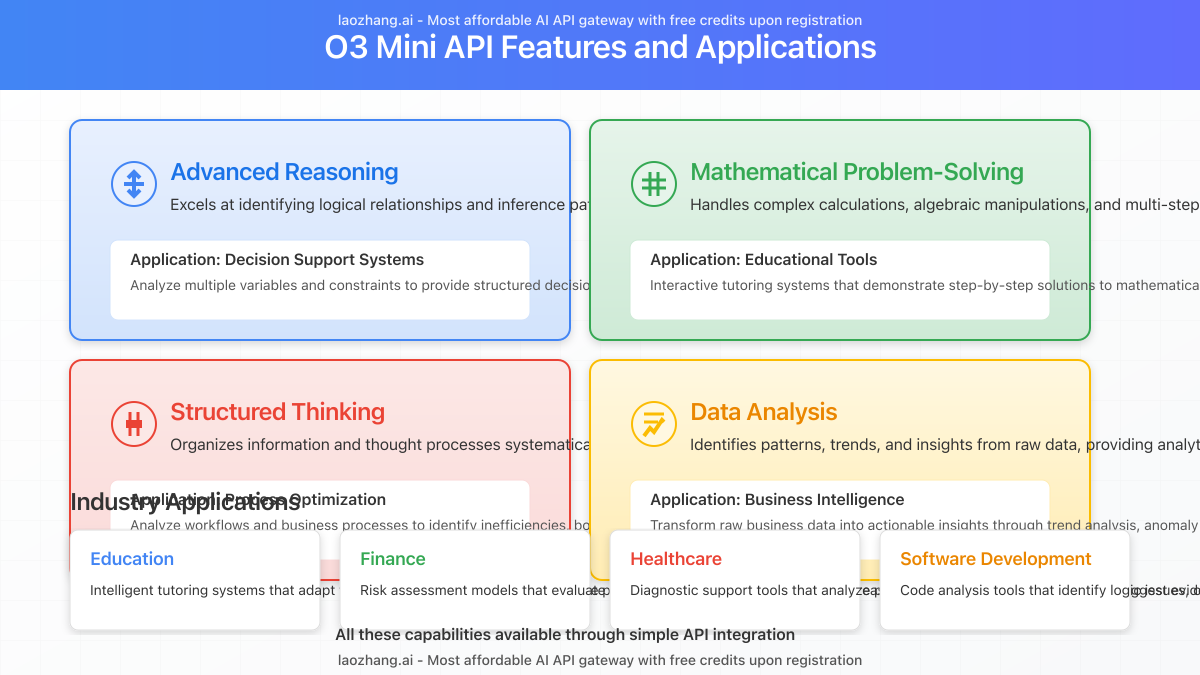
4. Community-Based API Sharing Projects
Several open-source projects aim to democratize access to advanced AI models through community-based sharing mechanisms. These projects provide shared API access through collective resources.
Popular community API sharing resources:
- ChatAnyware GitHub repository: Offers free API keys to GPT and other LLMs
- AI/ML API pooling services: Aggregate usage across multiple users
- Developer collectives that share API costs across members
Implementation example using a community API provider:
import requests
community_api_url = "https://community-api-provider.example/v1/chat/completions"
shared_key = "community_shared_api_key"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {shared_key}"
}
payload = {
"model": "o3-mini",
"messages": [
{"role": "user", "content": "Develop a logical proof for the statement: If a implies b, and b implies c, then a implies c."}
]
}
response = requests.post(community_api_url, headers=headers, json=payload)
print(response.json())
Considerations for community API sharing:
- Rate limits due to shared usage across multiple users
- Potential reliability issues during peak demand periods
- May require contribution to the community in some form
- Security considerations when using shared credentials
5. Educational and Research Access Programs
OpenAI and various organizations offer educational access programs that provide free or heavily discounted API credits for academic and research purposes.
How to qualify for educational access:
- Verify eligibility with an academic email address (.edu domains)
- Submit an application describing your research or educational project
- Provide details about expected API usage and research outcomes
- Await approval and credit allocation to your account
Implementation for educational projects remains the same as standard OpenAI API calls, but with special access credentials provided through the program.
Practical Applications of O3 Mini API
O3 Mini’s specialized reasoning capabilities make it particularly valuable for specific use cases:
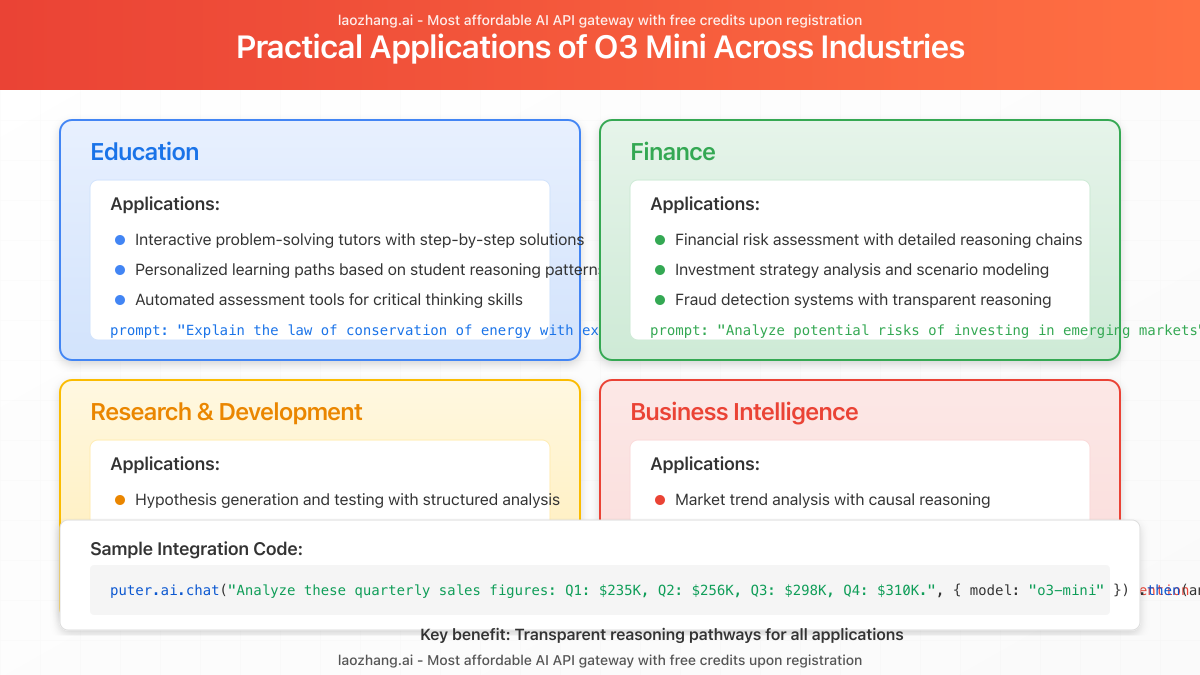
Advanced Problem Solving
// Using O3 Mini for complex reasoning tasks
puter.ai.chat(
"A bin contains 10 red balls, 15 blue balls, and 5 green balls. If 3 balls are drawn at random without replacement, what is the probability that all 3 balls are the same color?",
{ model: "o3-mini" }
).then(response => {
console.log("Solution:", response);
});
Logical Analysis and Evaluation
// Using O3 Mini for analyzing logical structures
const analyzeLogic = async () => {
const response = await puter.ai.chat(
"Analyze this logical argument structure: P1: All humans are mortal. P2: Socrates is human. C: Therefore, Socrates is mortal. Is this valid? Explain the logical form.",
{ model: "o3-mini" }
);
document.getElementById("analysis").innerText = response;
};
analyzeLogic();
Educational Assistants
import requests
import json
api_key = "your_laozhang_api_key"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}"
}
# Educational assistant prompt
payload = {
"model": "o3-mini",
"messages": [
{"role": "system", "content": "You are an educational assistant that explains complex concepts step by step."},
{"role": "user", "content": "Explain the concept of quantum superposition to a high school student."}
]
}
response = requests.post(
"https://api.laozhang.ai/v1/chat/completions",
headers=headers,
json=payload
)
explanation = response.json()["choices"][0]["message"]["content"]
print(explanation)
Data Analysis and Interpretation
// Data interpretation with O3 Mini
const sales_data = `
Quarter, North, South, East, West
Q1, 120000, 95000, 135000, 115000
Q2, 135000, 110000, 155000, 130000
Q3, 145000, 118000, 162000, 142000
Q4, 160000, 125000, 178000, 156000
`;
puter.ai.chat(
`Analyze this quarterly sales data and identify key trends and patterns. Provide insights on regional performance:\n${sales_data}`,
{ model: "o3-mini" }
).then(analysis => {
document.getElementById("data-analysis").innerHTML = analysis;
});
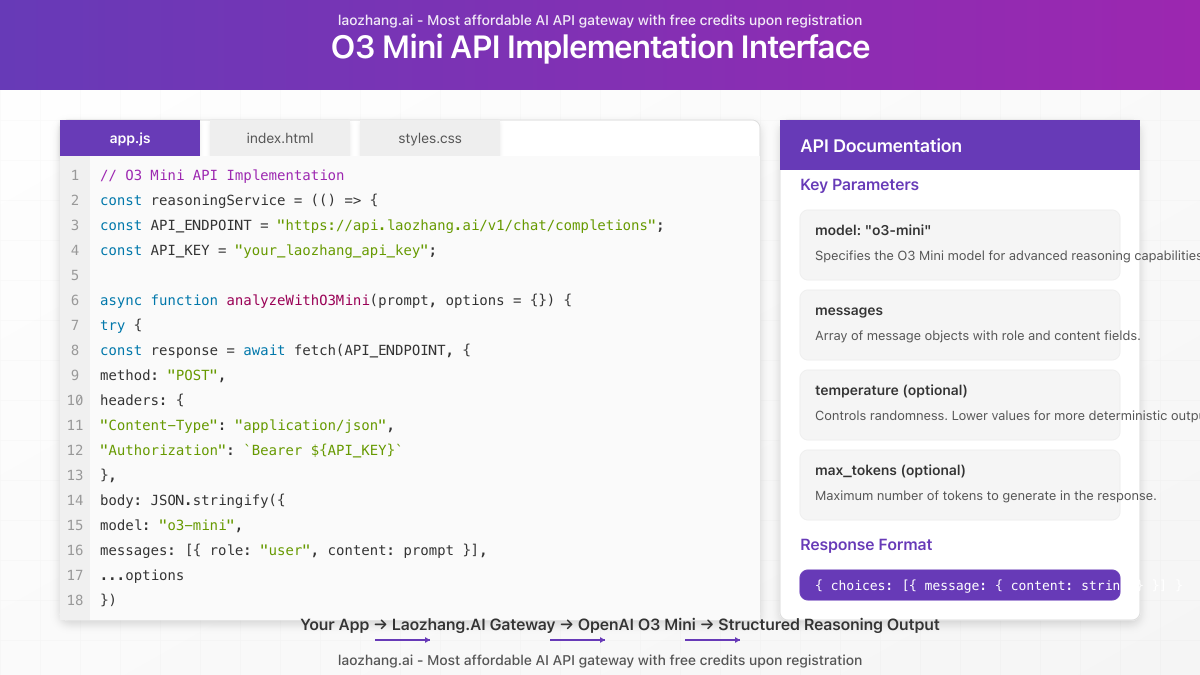
Best Practices for Free O3 Mini API Usage
To maximize the value of free O3 Mini API access while ensuring sustainable usage:
Optimize Prompt Engineering
The effectiveness of O3 Mini depends significantly on how you structure your prompts:
- Be specific and explicit about the reasoning task
- Break complex problems into smaller, manageable steps
- Provide context and constraints clearly
- Use system messages to define the model’s role (e.g., “You are a logical reasoning assistant”)
Example of optimized prompt structure:
// Poor prompt example
puter.ai.chat("Solve this problem: x^2 + 5x + 6 = 0");
// Optimized prompt example
puter.ai.chat(`
I need to solve the quadratic equation x^2 + 5x + 6 = 0
1. First, identify the values of a, b, and c
2. Use the quadratic formula: x = (-b ± √(b^2 - 4ac)) / 2a
3. Calculate the discriminant and determine the number of solutions
4. Find the exact values of all solutions
5. Verify the solutions by substituting them back into the original equation
`, { model: "o3-mini" });
Implement Caching Strategies
Reduce redundant API calls by implementing client-side caching:
// Simple cache implementation for O3 Mini responses
const responseCache = new Map();
async function getCachedResponse(prompt, model = "o3-mini") {
const cacheKey = `${prompt}|${model}`;
// Check if response exists in cache
if (responseCache.has(cacheKey)) {
console.log("Using cached response");
return responseCache.get(cacheKey);
}
// Get fresh response from API
console.log("Fetching new response");
const response = await puter.ai.chat(prompt, { model });
// Cache the response
responseCache.set(cacheKey, response);
return response;
}
// Usage
getCachedResponse("What is the square root of 144?").then(response => {
console.log(response);
});
Batch Processing for Efficiency
For applications requiring multiple inferences, consider batch processing approaches:
// Function to process multiple prompts efficiently
async function batchProcess(prompts, model = "o3-mini") {
// Process in batches of 5 to avoid overwhelming free services
const batchSize = 5;
let results = [];
for (let i = 0; i < prompts.length; i += batchSize) {
const batch = prompts.slice(i, i + batchSize);
const batchPromises = batch.map(prompt =>
puter.ai.chat(prompt, { model })
);
// Wait for all promises in current batch to resolve
const batchResults = await Promise.all(batchPromises);
results = [...results, ...batchResults];
// Add delay between batches if needed
if (i + batchSize < prompts.length) {
await new Promise(r => setTimeout(r, 2000));
}
}
return results;
}
// Example usage
const mathProblems = [
"Solve for x: 2x + 3 = 7",
"Calculate the area of a circle with radius 5",
"Find the derivative of f(x) = x² + 3x - 2",
"Simplify the expression: (2x + 3)(x - 4)",
"Solve the system of equations: 3x + 2y = 7, x - y = 1",
"Calculate the limit of (x² - 1)/(x - 1) as x approaches 1",
"Find the indefinite integral of 2x + 3",
"Solve the inequality: 2x - 3 > 7",
"Find the domain of f(x) = √(x - 2)",
"Calculate the probability of rolling a sum of 7 with two dice"
];
batchProcess(mathProblems).then(solutions => {
solutions.forEach((solution, index) => {
console.log(`Problem ${index + 1} Solution:`, solution);
});
});
Comparing Free O3 Mini Access Methods
Method | Setup Complexity | Usage Limits | Reliability | Best For |
---|---|---|---|---|
Puter.js | Very Low | User-dependent | High | Frontend applications, rapid prototyping |
Laozhang.AI | Low | Free credits upon registration | Very High | Production applications, consistent usage |
OpenAI Free Tier | Medium | Time-limited promotional credits | High | Official development, testing with OpenAI |
Community API Sharing | Medium-High | Shared among community | Medium | Non-critical applications, experimentation |
Educational Access | High | Project-based allocation | High | Academic research, educational projects |
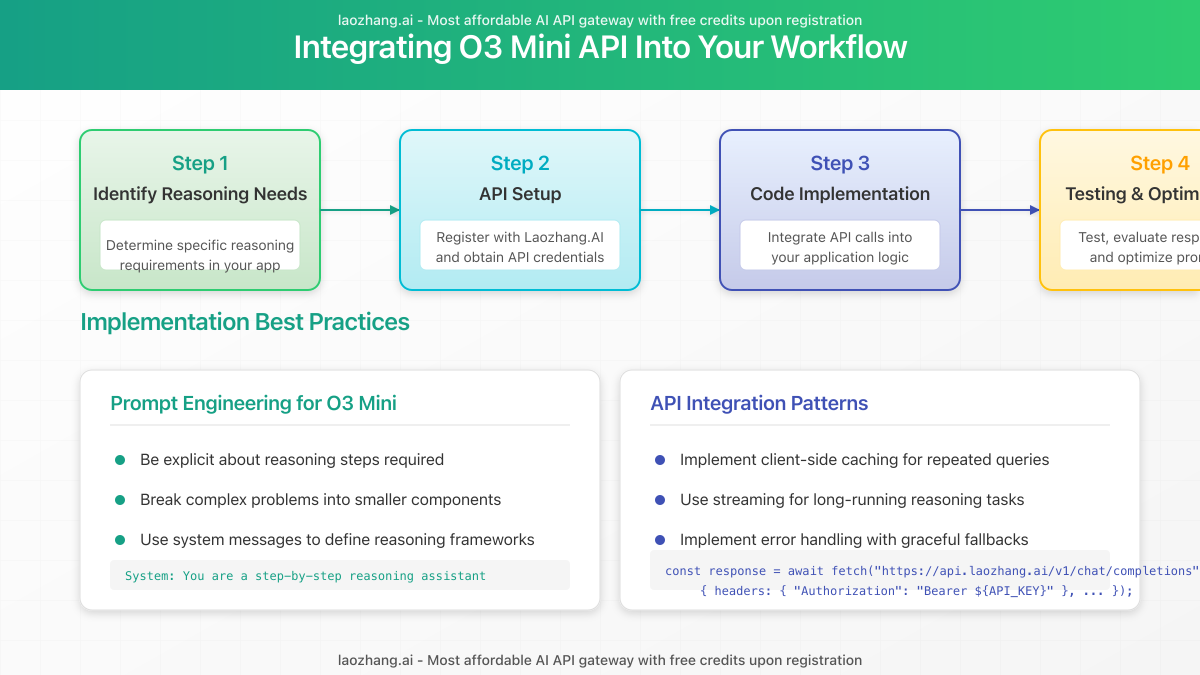
Conclusion: Making the Most of Free O3 Mini API Access
O3 Mini represents a significant advancement in AI reasoning capabilities, and the various free access methods provide developers with opportunities to explore and implement these capabilities without immediate cost barriers.
For sustainable long-term usage, Laozhang.AI emerges as the most balanced option, offering free starter credits and affordable rates for continued use. The service provides the reliability and consistency needed for serious development while maintaining API compatibility with OpenAI’s standard.
As you begin integrating O3 Mini into your applications:
- Start with Puter.js for quick prototyping and concept validation
- Register with Laozhang.AI to secure your free credits for more extensive testing
- Optimize your implementation with the best practices outlined in this guide
- Monitor usage patterns to determine the most cost-effective long-term strategy
By leveraging these approaches, you can harness the advanced reasoning capabilities of O3 Mini while managing costs effectively, opening new possibilities for AI-enhanced applications across various domains.
Frequently Asked Questions
What makes O3 Mini different from other OpenAI models?
O3 Mini is specifically optimized for reasoning tasks, providing advanced logical analysis and problem-solving capabilities at a fraction of the computational cost of larger models. While models like GPT-4o excel at general tasks, O3 Mini is specialized for structured thinking and complex reasoning.
How long will free access to O3 Mini be available?
Free promotional access from OpenAI is typically time-limited, while services like Puter.js and Laozhang.AI offer ongoing free tiers with specific limitations. For the most current information, check the respective platforms’ websites regularly.
Can I use O3 Mini for commercial applications?
Yes, O3 Mini can be used for commercial applications. However, ensure you’re complying with the terms of service for whichever access method you choose. For commercial applications, services like Laozhang.AI provide reliable access with clear usage terms.
What are the usage limits for free O3 Mini access?
Usage limits vary by platform: OpenAI’s promotional credits have specific caps, Puter.js limits are determined by end-user constraints, and Laozhang.AI provides a set amount of free credits upon registration. Check each service’s current documentation for specific limitations.
How does O3 Mini compare to Claude or Google’s Gemini models?
O3 Mini specializes in reasoning capabilities, often outperforming comparable models in logical analysis and structured problem-solving. While Claude and Gemini offer strong general performance, O3 Mini’s focused design provides exceptional efficiency for specific reasoning tasks.
What happens when my free O3 Mini credits run out?
When free credits expire, you’ll typically need to purchase additional credits to continue usage. Laozhang.AI offers competitive pricing for continued access, while OpenAI requires standard API payment. Always monitor your usage to avoid unexpected interruptions.