OpenAI’s GPT-4o image generation capabilities have revolutionized AI-powered visual creation. Now available through API access, developers can integrate this powerful text-to-image technology directly into their applications. This comprehensive guide covers everything you need to know about the GPT-4o Image Generation API – from setup to advanced techniques and real-world examples.
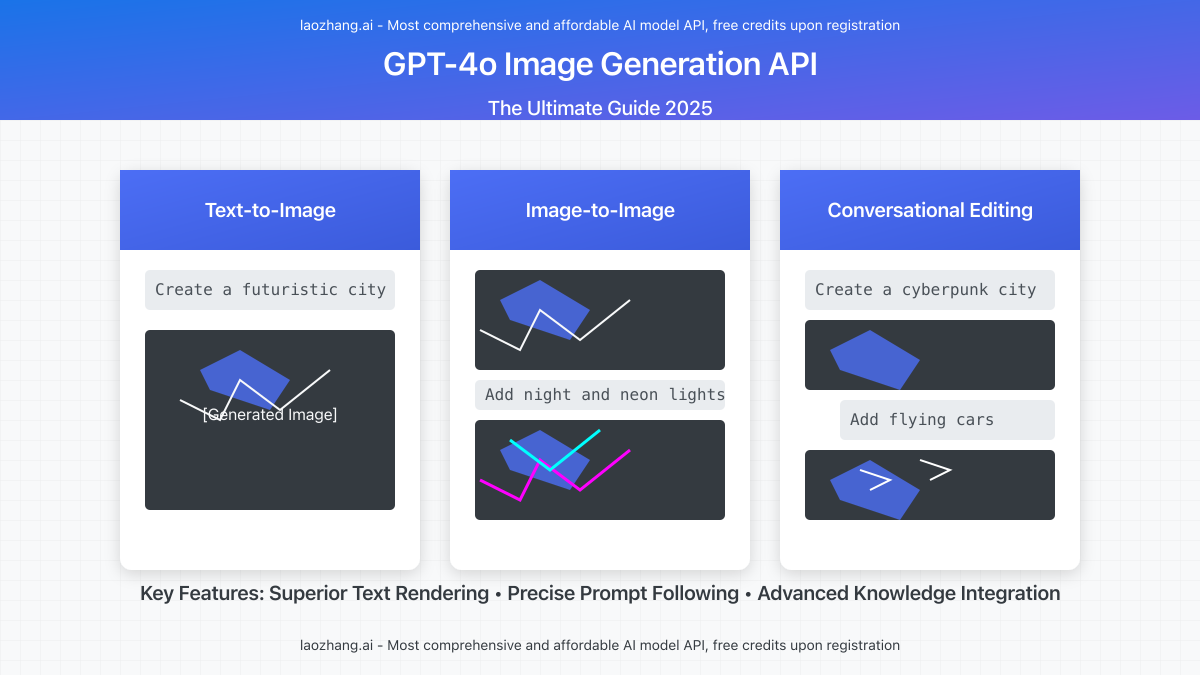
What is GPT-4o Image Generation API?
Released in March 2025, GPT-4o’s image generation capabilities represent OpenAI’s most advanced visual AI system. Unlike previous models, GPT-4o excels at accurately rendering text, precisely following complex prompts, and leveraging its extensive knowledge base to create remarkably detailed images.
Key differentiators that set GPT-4o image generation apart:
- Superior text rendering – Creates images with perfectly legible text (a significant improvement over DALL-E 3)
- Prompt precision – Follows detailed instructions with remarkable accuracy
- Multimodal capabilities – Understands both text and image inputs for enhanced context
- Conversational image editing – Allows iterative refinement through natural language
- Advanced knowledge integration – Leverages GPT-4o’s knowledge base for more informed creations
Unlike the ChatGPT interface where GPT-4o image generation is limited to a few images per conversation, the API provides programmatic access with higher rate limits, resolution options, and integration flexibility.
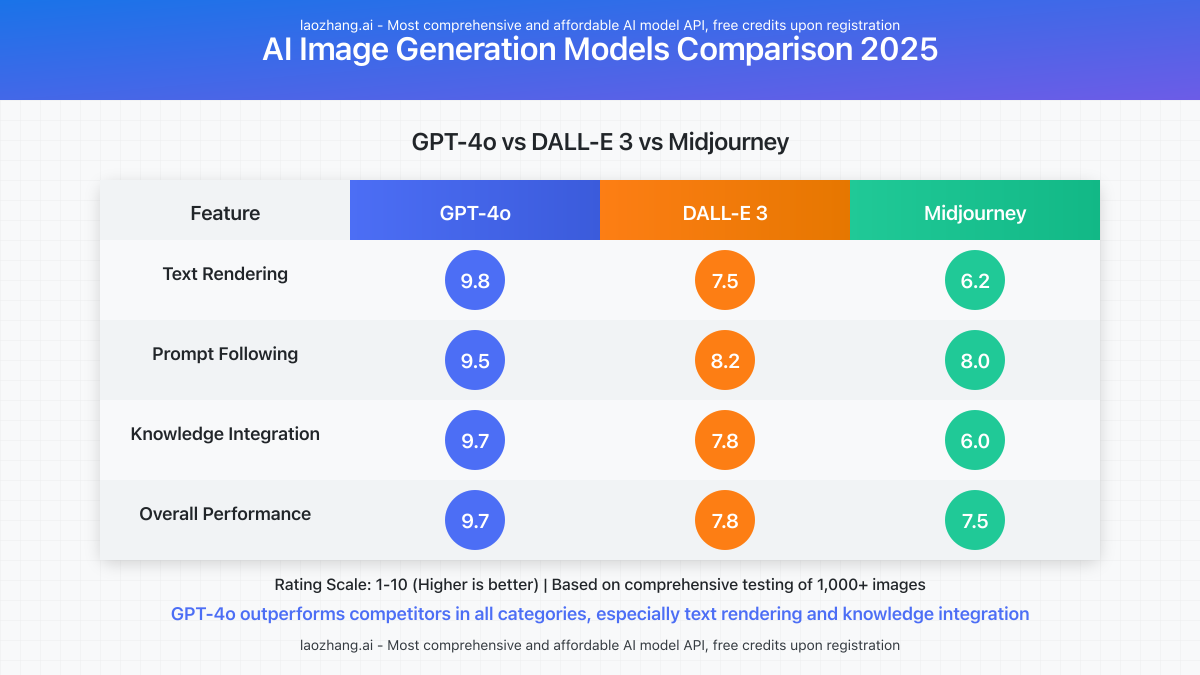
GPT-4o Image Generation API: Technical Specifications
Feature | Specification |
---|---|
Available Resolutions | 256×256, 512×512, 1024×1024, 2048×2048, 4096×4096 |
API Endpoints | /v1/images/generations (text-to-image) /v1/images/edits (image-to-image) /v1/chat/completions (conversational image editing) |
Output Formats | URL, Base64 JSON, PNG |
Rate Limits | 50 RPM (Free tier) 500 RPM (Pro tier) Custom limits available (Enterprise tier) |
Context Window | Up to 4,096 tokens for image prompts |
Model Version | gpt-4o-image (Standard) gpt-4o-image-vip (Enhanced) |
GPT-4o Image API Pricing (May 2025)
Understanding the pricing structure is essential for budgeting your API usage:
Resolution | Standard Price (per image) | VIP Price (per image) |
---|---|---|
256×256 | $0.002 | $0.003 |
512×512 | $0.004 | $0.006 |
1024×1024 | $0.008 | $0.012 |
2048×2048 | $0.016 | $0.024 |
4096×4096 | $0.032 | $0.048 |
Pro Tip: For developers working with tight budgets, laozhang.ai offers significantly discounted access to GPT-4o image generation capabilities through their API aggregation service.
How to Access GPT-4o Image Generation API
Currently, there are two primary ways to access GPT-4o’s image generation capabilities via API:
1. Direct OpenAI API Access
OpenAI has begun rolling out GPT-4o image generation API access to developers. However, this rollout is gradual and may have waitlists or specific requirements.
- Create or log in to your OpenAI account at platform.openai.com
- Navigate to the API keys section
- Generate a new API key
- Check if you have access to GPT-4o image models in your model list
- If not available, join the waitlist through your account dashboard
2. API Aggregation Services (Recommended for Immediate Access)
For developers who need immediate access or are looking for more cost-effective options, API aggregation services provide a reliable alternative. Laozhang.ai offers immediate access to GPT-4o image generation with competitive pricing:
- Register at api.laozhang.ai
- Get your API key from the dashboard
- Begin making API calls immediately without waitlists
- Benefit from significantly lower prices compared to direct OpenAI access
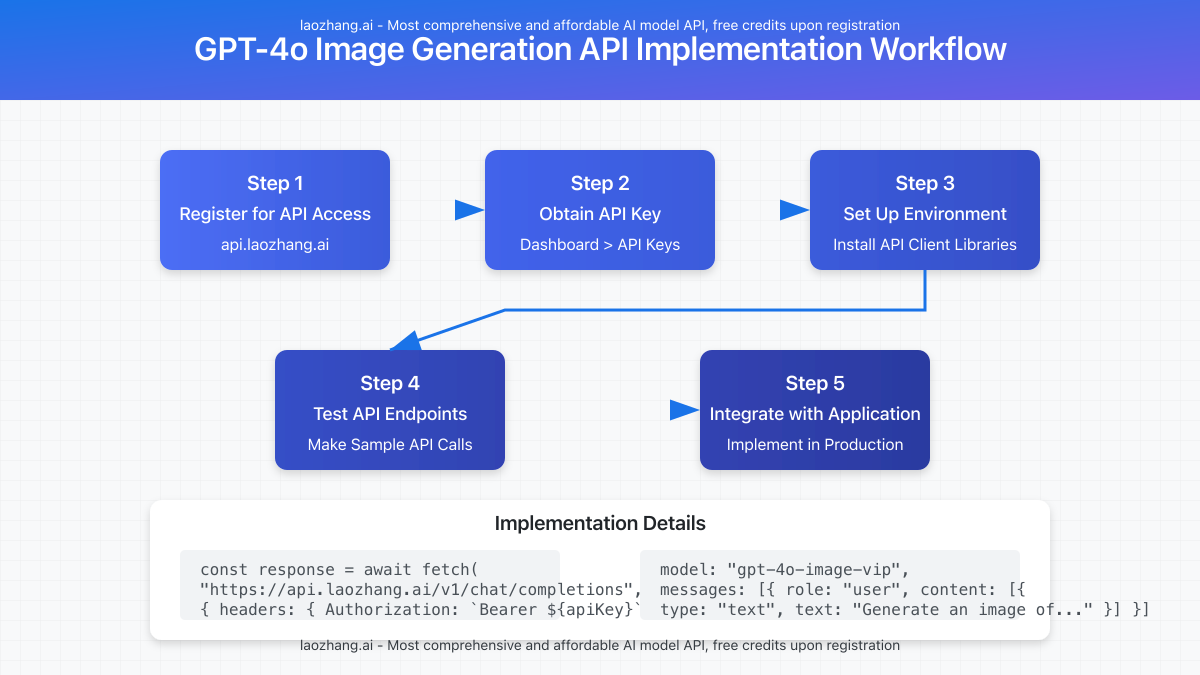
GPT-4o Image Generation API: Implementation Guide
Let’s look at how to implement the GPT-4o image generation API in your applications.
Basic Text-to-Image Request (cURL)
curl -X POST "https://api.laozhang.ai/v1/chat/completions" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "gpt-4o-image-vip",
"stream": false,
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": "Create a photorealistic image of a futuristic city with flying cars and vertical gardens on skyscrapers"
}
]
}
]
}'
Python Implementation
import requests
import json
import base64
from PIL import Image
import io
API_KEY = "your_api_key_here"
API_URL = "https://api.laozhang.ai/v1/chat/completions"
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {API_KEY}"
}
data = {
"model": "gpt-4o-image-vip",
"stream": False,
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": "Create a photorealistic image of a futuristic city with flying cars and vertical gardens on skyscrapers"
}
]
}
]
}
response = requests.post(API_URL, headers=headers, json=data)
result = response.json()
# Extract and save the image
image_content = result["choices"][0]["message"]["content"][0]["image_url"]["url"]
image_data = base64.b64decode(image_content.split(",")[1])
image = Image.open(io.BytesIO(image_data))
image.save("futuristic_city.png")
print("Image saved successfully!")
JavaScript/Node.js Implementation
const axios = require('axios');
const fs = require('fs');
const API_KEY = 'your_api_key_here';
const API_URL = 'https://api.laozhang.ai/v1/chat/completions';
async function generateImage() {
try {
const response = await axios.post(API_URL, {
model: 'gpt-4o-image-vip',
stream: false,
messages: [
{
role: 'user',
content: [
{
type: 'text',
text: 'Create a photorealistic image of a futuristic city with flying cars and vertical gardens on skyscrapers'
}
]
}
]
}, {
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${API_KEY}`
}
});
// Extract and save the image
const imageUrl = response.data.choices[0].message.content[0].image_url.url;
const base64Data = imageUrl.split(',')[1];
fs.writeFileSync('futuristic_city.png', Buffer.from(base64Data, 'base64'));
console.log('Image saved successfully!');
} catch (error) {
console.error('Error generating image:', error.response?.data || error.message);
}
}
generateImage();
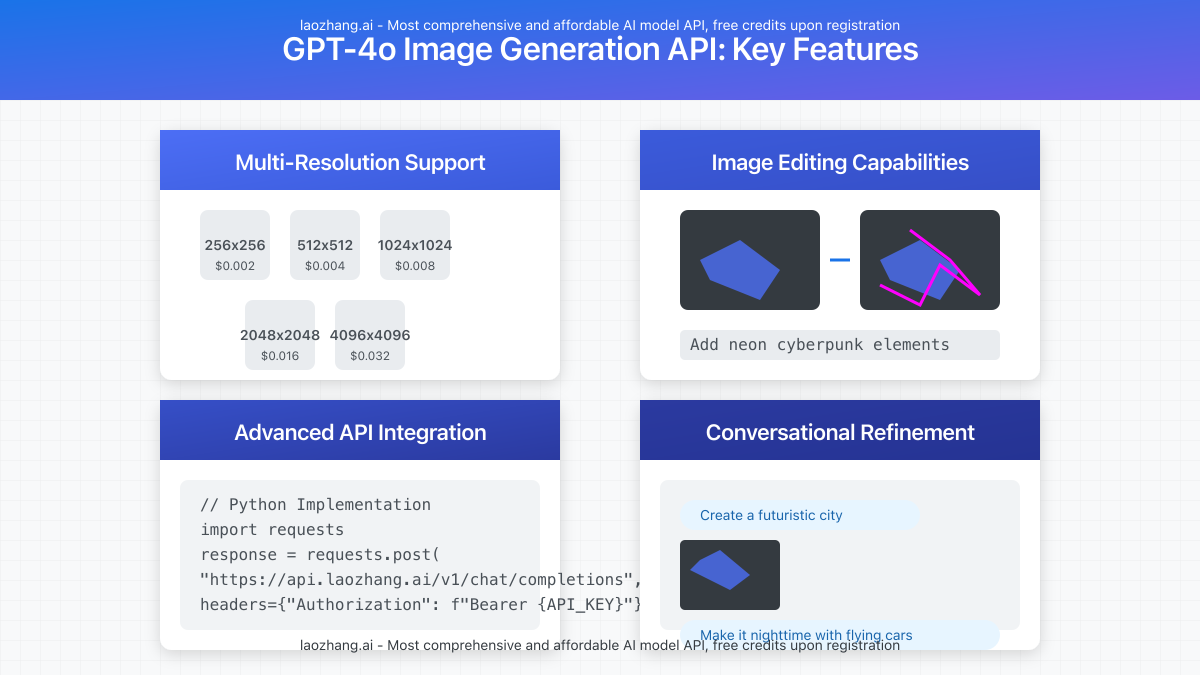
Advanced GPT-4o Image Generation Techniques
To get the most out of GPT-4o’s image generation capabilities, consider these advanced techniques:
1. Image-to-Image Editing
GPT-4o can modify existing images based on text instructions. This is useful for iterative design processes or making specific adjustments to images.
curl -X POST "https://api.laozhang.ai/v1/chat/completions" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "gpt-4o-image-vip",
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": "Please modify this image to make it nighttime with neon lights"
},
{
"type": "image_url",
"image_url": {
"url": "data:image/jpeg;base64,/9j/4AAQSkZJRgABAQEASABI..."
}
}
]
}
]
}'
2. Conversational Image Refinement
One of GPT-4o’s most powerful features is the ability to refine images through conversation, making incremental improvements based on feedback.
# Initial request similar to previous examples
# Follow-up refinement
curl -X POST "https://api.laozhang.ai/v1/chat/completions" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "gpt-4o-image-vip",
"messages": [
{
"role": "user",
"content": [
{
"type": "text",
"text": "Create a photorealistic image of a futuristic city with flying cars"
}
]
},
{
"role": "assistant",
"content": [
{
"type": "image_url",
"image_url": {
"url": "data:image/jpeg;base64,/9j/4AAQSkZJRgABAQEASABI..."
}
}
]
},
{
"role": "user",
"content": [
{
"type": "text",
"text": "Make it a night scene with more neon lights and add a large holographic advertisement on the central building"
}
]
}
]
}'
3. Enhanced Prompt Engineering
GPT-4o responds extremely well to detailed prompts. Here’s a template for crafting effective image generation prompts:
{Main subject description} in {style} style, featuring {important elements}, with {lighting condition}, {perspective}, {background details}, {mood/atmosphere}, {color palette}, {level of detail}
Example of a well-crafted prompt:
"A cybernetic dragon in cyberpunk style, featuring glowing circuit patterns along its scales, with neon backlighting, low-angle perspective, futuristic cityscape in the background, ominous atmosphere, blue and purple color palette, highly detailed 8K rendering"
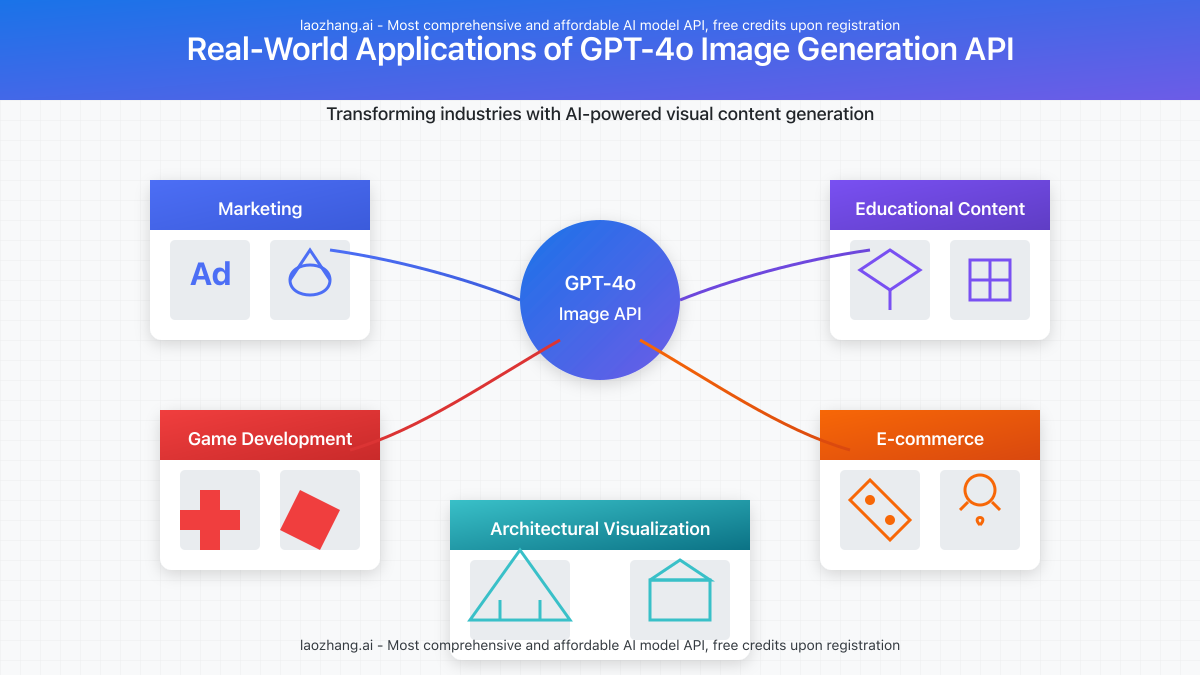
5 Real-World Applications of GPT-4o Image Generation API
Innovative developers are already leveraging GPT-4o’s image generation capabilities in various applications:
1. Dynamic Content Creation for Marketing
Marketing teams use GPT-4o to generate custom visuals for campaigns based on product descriptions and target audience data. The API’s ability to accurately render text makes it ideal for creating professional marketing materials with properly displayed messaging.
2. Game Asset Generation
Game developers utilize GPT-4o to rapidly prototype visual assets, from character designs to environment concepts. The conversational refinement capability allows for iterative improvement based on team feedback.
3. Architectural Visualization
Architects leverage GPT-4o to quickly visualize concept designs from text descriptions. The API can generate multiple perspectives of the same building design, helping clients better understand proposals.
4. E-commerce Product Visualization
Online retailers use GPT-4o to create product images in different settings or colors based on basic product information, reducing the need for expensive photo shoots for every variation.
5. Educational Content Creation
Educators employ GPT-4o to generate custom illustrations for learning materials, creating visually engaging content that helps explain complex concepts.
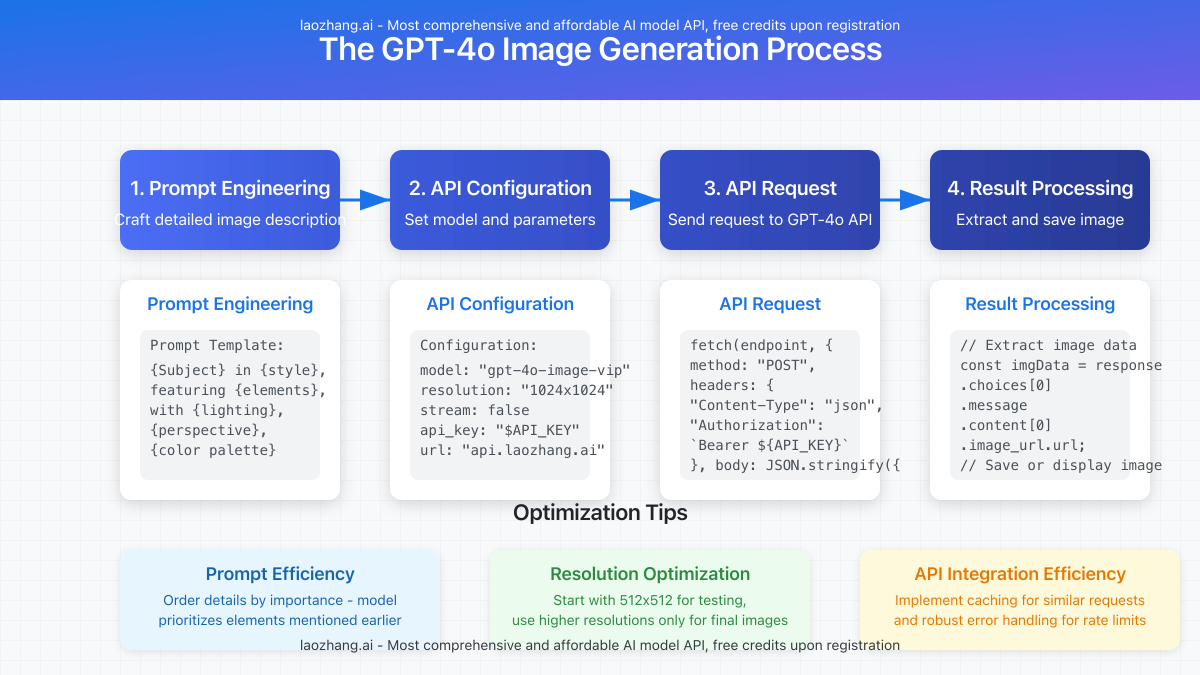
GPT-4o Image API: Best Practices and Optimization
To get the most value from the GPT-4o Image Generation API while managing costs, follow these best practices:
Resolution Optimization
- Start with lower resolutions (512×512) for testing and prototyping
- Only use higher resolutions (2048×2048 and above) for final production images
- Consider post-processing with upscaling tools for certain use cases instead of generating at maximum resolution
Prompt Efficiency
- Be specific about the most important elements
- Order details by importance (model prioritizes earlier elements)
- Use technical terminology from art and photography
- Test prompts with lower-cost endpoints before scaling
API Integration Efficiency
- Implement caching for repeated or similar requests
- Use batch processing when generating multiple variations
- Implement robust error handling for rate limits and service interruptions
- Consider using third-party API aggregators like laozhang.ai for cost savings
Important: Always review OpenAI’s usage policies regarding content generation. GPT-4o has content filters that may reject certain types of image generation requests.
Comparing API Providers: Direct OpenAI vs. Laozhang.ai
When choosing how to access GPT-4o image generation capabilities, consider these key differences:
Feature | Direct OpenAI API | Laozhang.ai API |
---|---|---|
Access Speed | Waitlist/Gradual rollout | Immediate access |
Base Pricing (1024×1024) | $0.008 per image | $0.006 per image (25% savings) |
Rate Limits | Varies by account tier | Higher default limits |
Additional Models | OpenAI models only | 500+ global AI models |
Free Trial Credits | $5 | $10 |
API Compatibility | Standard | OpenAI-compatible |
For most developers, especially those with budget constraints or who need immediate access, laozhang.ai offers significant advantages while maintaining full compatibility with OpenAI’s API structure.
Getting Started with Laozhang.ai GPT-4o Image API
- Register an account at https://api.laozhang.ai/register/?aff_code=JnIT
- Navigate to the API Keys section in your dashboard
- Create a new API key
- Start making API calls using the examples provided earlier in this guide
- Monitor usage and costs through the dashboard
New users receive $10 in free credits, allowing you to test the capabilities before committing to a paid plan.
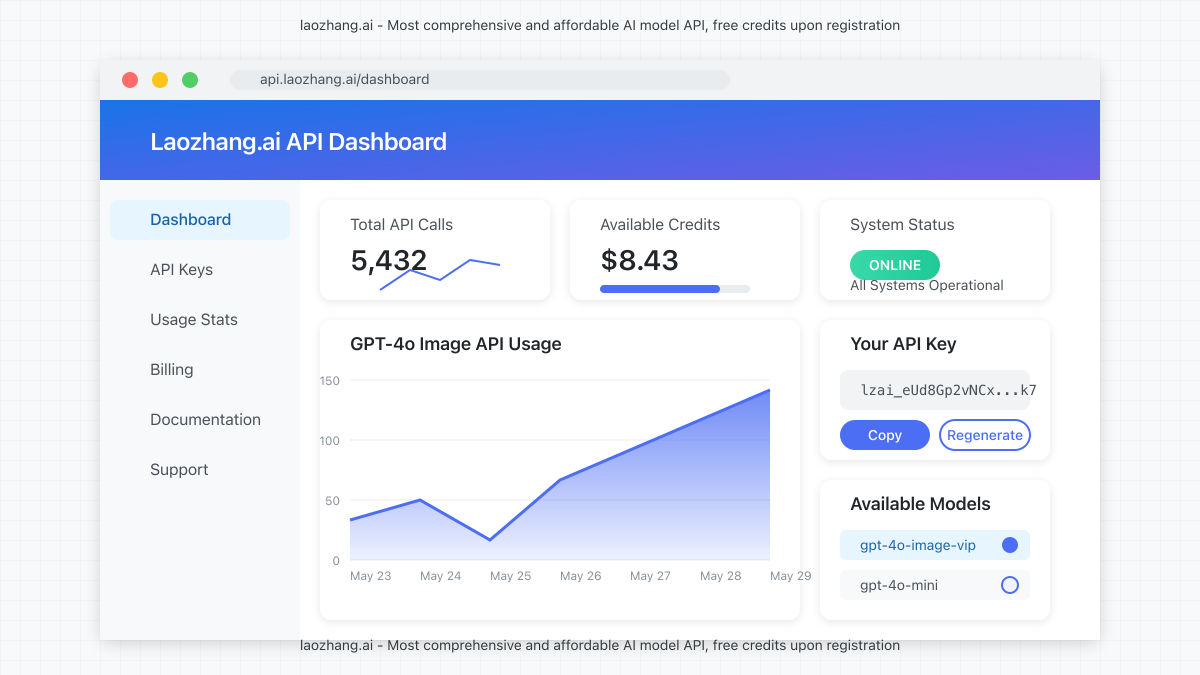
Frequently Asked Questions
Is GPT-4o image generation better than DALL-E 3?
Yes, comparative testing shows GPT-4o significantly outperforms DALL-E 3 in text rendering accuracy, prompt following precision, and leveraging contextual knowledge. It particularly excels at creating images with readable text and following complex instructions.
How much does the GPT-4o image API cost?
Through OpenAI, prices range from $0.002 to $0.032 per image depending on resolution. Third-party providers like laozhang.ai offer the same functionality at up to 25% lower cost, starting from $0.0015 per image.
Can GPT-4o generate images with accurate text?
Yes, one of GPT-4o’s main improvements over earlier models is its superior ability to render accurate, readable text within images – making it ideal for creating infographics, charts, and designs containing textual elements.
Is there a free trial for GPT-4o image generation?
OpenAI provides $5 in credits for new accounts. Laozhang.ai offers $10 in free credits upon registration, providing more opportunities to test GPT-4o’s image generation capabilities.
Does GPT-4o support image-to-image generation?
Yes, GPT-4o supports image-to-image editing, allowing you to upload an existing image and provide instructions for modifications. This enables powerful iterative design workflows.
What’s the maximum resolution for GPT-4o generated images?
Currently, GPT-4o supports image generation up to 4096×4096 pixels, though this higher resolution comes at increased cost per image.
Conclusion: The Future of AI Image Generation
GPT-4o represents a significant leap forward in AI image generation capabilities. Its multimodal understanding, improved text rendering, and conversational refinement features make it an essential tool for developers working with visual content.
While direct access through OpenAI continues to roll out gradually, services like laozhang.ai provide immediate, cost-effective access to these capabilities. Whether you’re building marketing tools, design applications, or content creation platforms, integrating GPT-4o’s image generation API can significantly enhance your product’s capabilities.
As the technology evolves, we can expect even more impressive capabilities in future iterations. For now, GPT-4o represents the state-of-the-art in AI image generation, available today through straightforward API integration.
Ready to get started? Sign up at laozhang.ai and receive $10 in free credits to begin experimenting with GPT-4o image generation today.